Linux 驱动读取当前内核中代码段数据
linux-dash
A beautiful web dashboard for Linux
项目地址:https://gitcode.com/gh_mirrors/li/linux-dash

·
直接上代码,思路就是得到代码段的起始地址和结束地址,然后先将数据存在一个buffer中,最后写入文件。
kallsym.c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/kallsyms.h>
static char *path = NULL;
module_param(path, charp, S_IRUGO);
static int __write_to_file(char *buffer, int size)
{
struct file *filep = NULL;
loff_t pos = 0;
int ret = 0;
filep = filp_open(path, O_RDWR | O_CREAT, 0);
if(filep == NULL) {
printk("%s: Open file %s error\n", __func__, path);
return 0;
}
ret = kernel_write(filep, buffer, size, &pos);
if(ret < size) {
printk("%s: Write file %s error\n", __func__, path);
return 0;
}
filp_close(filep, NULL);
return ret;
}
static int __init kallsyms_init(void)
{
unsigned long stext, etext, size = 0;
char *ptr = NULL;
char *buffer = NULL;
int i = 0;
stext = kallsyms_lookup_name("_stext");
etext = kallsyms_lookup_name("_etext");
printk("%s: the _stext address is %lx\n", __func__, stext);
printk("%s: the _stext address is %lx\n", __func__, etext);
if(!stext || !etext) {
printk("%s: Get text start end failed\n", __func__);
return -EINVAL;
}
size = etext - stext;
ptr = (char *)stext;
buffer = vzalloc(size + 1024);
if(buffer == NULL) {
printk("%s: Alloc temp buffer memory failed\n", __func__);
return 0;
}
for(i = 0; i < size; i++) {
buffer[i] = ptr[i];
}
__write_to_file(buffer, size);
vfree(buffer);
return 0;
}
static void __exit kallsyms_exit(void)
{
printk("%s: good bye\n", __func__);
}
module_init(kallsyms_init);
module_exit(kallsyms_exit);
MODULE_LICENSE("GPL");
Makefile:
obj-m += kallsym.o
KBUILD_CFLAGS += -g
all:
make -C /lib/modules/$(shell uname -r)/build/ M=$(PWD) modules
clean:
make -C /lib/modules/$(shell uname -r)/build/ M=$(PWD) clean
以下代码为dump指定内存地址,大小为offset;
#include <linux/init.h>
#include <linux/module.h>
#include <linux/kallsyms.h>
static char *path = NULL;
static unsigned long base_addr = 0;
static unsigned int offset = 0;
module_param(path, charp, S_IRUGO);
module_param(base_addr, ulong, S_IRUGO);
module_param(offset, uint, S_IRUGO);
static int __write_to_file(char *buffer, int size)
{
struct file *filep = NULL;
loff_t pos = 0;
int ret = 0;
filep = filp_open(path, O_RDWR | O_CREAT, 0);
if(filep == NULL) {
printk("%s: Open file %s error\n", __func__, path);
return 0;
}
ret = kernel_write(filep, buffer, size, &pos);
if(ret < size) {
printk("%s: Write file %s error\n", __func__, path);
return 0;
}
filp_close(filep, NULL);
return ret;
}
static int __init kallsyms_init(void)
{
unsigned long stext, etext;
char *ptr = NULL;
char *buffer = NULL;
int i = 0;
stext = base_addr;
etext = base_addr + offset;
printk("%s: the _stext address is %lx\n", __func__, stext);
printk("%s: the _stext address is %lx\n", __func__, etext);
ptr = (char *)stext;
buffer = vzalloc(offset + 100);
if(buffer == NULL) {
printk("%s: Alloc temp buffer memory failed\n", __func__);
return 0;
}
for(i = 0; i < offset; i++) {
buffer[i] = ptr[i];
}
__write_to_file(buffer, offset);
vfree(buffer);
return 0;
}
static void __exit kallsyms_exit(void)
{
printk("%s: good bye\n", __func__);
}
module_init(kallsyms_init);
module_exit(kallsyms_exit);
MODULE_LICENSE("GPL");
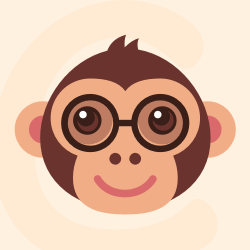



A beautiful web dashboard for Linux
最近提交(Master分支:2 个月前 )
186a802e
added ecosystem file for PM2 4 年前
5def40a3
Add host customization support for the NodeJS version 4 年前
更多推荐
所有评论(0)