Linux /C/C++文件流读写操作的详解
linux-dash
A beautiful web dashboard for Linux
项目地址:https://gitcode.com/gh_mirrors/li/linux-dash

·
C语言文件指针定义
文件的打开与关闭
fopen函数
头文件:stdio.h
功 能:以type方式打开filename文件并返回该文件的指针
用 法:FILE *fopen(char *filename,char *type);
返回值:filename的文件指针
注意:
1.凡用“r”打开一个文件时,该文件必须已经存在,且只能从该文件读出。
2.用“w”打开的文件只能向该文件写入。若打开的文件不存在,则以指定的文件名建立该文件,若打开的文件已经存在,则将该文件删去,重建一个新文件。若以写或读写方式打开一个已存在的文件时将清除原来文件的内容,希望写入的字符以文件末开始存放,必须以追加方式打开文件。
3.若要向一个已存在的文件追加新的信息,只能用“a”方式打开文件。但此时该文件必须是存在的,否则将会出错。
fclose函数
头文件:stdio.h
功 能:关闭一个流
用 法:int fclose(FILE *stream);
返回值:成功返回0,不成功返回EOF(-1)
文件的读写(头文件均为stdio.h)
字符读写函数
fgetc函数
功 能:从fp所指向的文件中读取字符
用 法:int fgetc(FILE *fp);
返回值:返回文件fp所指向的文件中的字符值(EOF为文件尾)
补 充:
1.调用该函数时,文件使用方式必须是以读或读写方式打开的。
2.在文件内部有一个位置指针,用来指向文件的当前读写
fputc函数
功能:将字符(ch的值)输出到fp所指向的文件中去。
用法:int futc(int ch,FILE *fp);
返回值:写入成功返回写入字符ch
不成功返回EOF
- #include <stdio.h>
- int main()
- {
- FILE *fp;
- char ch,filename[20];
- printf("Please input your filename:");
- scanf("%s",filename);
- if(!(fp=fopen(filename,"w")))
- {
- printf("Can not open %s\n",filename);
- }
- else
- {
- printf("Please input the sentences you write:");
- ch=getchar();
- ch=getchar();
- while(ch!=EOF)
- {
- fputc(ch,fp);
- ch=getchar();
- }
- fclose(fp);
- }
- if(!(fp=fopen(filename,"r")))
- {
- printf("Can not open %s\n",filename);
- }
- else
- {
- printf("The content of %s is:",filename);
- while(!feof(fp))
- {
- ch=fgetc(fp);
- putchar(ch);
- }
- printf("\n");
- fclose(fp);
- }
- return 0;
- }
字符串读写函数
fgets函数
功 能:从fp所指向的文件(stdin特殊文件)中读取长度为n的字符串保存到string中
用 法:char *fgets(char *string, int n, FILE *fp);
返回值:成功,返回string
失败,返回NULL
fputs函数
功 能:将字符串string写入fp所指向的文件中。
用 法:int fputs(char *string, FILE *fp);
返回值:输入成功,返回值0
输入失败,返回EOF
示例:
- #include <stdio.h>
- #define LEN 100
- int main()
- {
- FILE *fp;
- char ch,string[LEN],filename[20],string1[LEN];
- printf("Please input your filename:");
- scanf("%s",filename);
- if(!(fp=fopen(filename,"w")))
- {
- printf("Can not open %s\n",filename);
- }
- else
- {
- printf("Please input the sentences you write:");
- ch=getchar();
- fgets(string,LEN,stdin);
- if(!fputs(string,fp))
- printf("写入成功\n");
- else
- printf("写入失败\n");
- fclose(fp);
- }
- if(!(fp=fopen(filename,"r")))
- {
- printf("Can not open %s\n",filename);
- }
- else
- {
- printf("The content of %s is:",filename);
- fgets(string1,LEN,fp);
- printf("%s\n",string1);
- fclose(fp);
- }
- return 0;
- }
数据块读写函数
fread函数
功 能:从fp指向的文件中读取n个size大小的数据写入ptr指向的地方
用 法:int fread(void *ptr, int size, int n, FILE *fp);
返回值:成功,返回读取元素个数
不成功,返回0
参数说明:
ptr:读入数据的存放地址(首地址)
size:要读写的字节数
n:要进行读写多少个size字节的数据项
fwrite函数
功 能:
从ptr指向的地方读取n个size大小的数据写入fp指向的文件中
用 法:int fwrite(void *ptr, int size, int n, FILE *fp);
返回值:返回写入文件的实际个数
参数说明:ptr:输出数据的地址(首地址)其余同上
注意:这个函数以二进制形式对文件进行操作,不局限于文本文件
示例:
- #include <stdio.h>
- #define SIZE 5
- typedef struct
- {
- char name[20];
- char num[15];
- int age;
- }student;
- student stu[SIZE],buf[SIZE];;
- bool save();
- void read();
- int main()
- {
- int i;
- for(i=0;i<SIZE;i++)
- scanf("%s %s %d",&stu[i].name,&stu[i].num,&stu[i].age);
- //fread(&stu[i],sizeof(student),1,stdin);
- if(save())
- {
- read();
- for(i=0;i<SIZE;i++)
- printf("name:%s num:%s age:%d\n",buf[i].name,buf[i].num,buf[i].age);
- }
- return 0;
- }
- bool save()
- {
- FILE *fp;
- int i;
- char filename[50];
- printf("Please input your filename:");
- scanf("%s",filename);
- if(!(fp=fopen(filename,"wb")))
- {
- printf("Can not open %s\n",filename);
- return false;
- }
- for(i=0;i<SIZE;i++)
- if(fwrite(&stu[i],sizeof(student),1,fp)!=1)
- printf("文件写入失败!\n");
- fclose(fp);
- return true;
- }
- void read()
- {
- FILE *fp;
- int i;
- char filename[50];
- printf("Please input the filename you want read:");
- scanf("%s",filename);
- if(!(fp=fopen(filename,"r")))
- {
- printf("Can not open %s\n",filename);
- return;
- }
- for(i=0;i<SIZE;i++)
- if(fread(&buf[i],sizeof(student),1,fp)!=1)
- printf("文件读取失败!\n");
- fclose(fp);
- }
- /*
- Alice 001 18
- Bob 002 18
- Cobe 003 18
- Dalin 004 18
- Ela 005 18
- */
格式化读写函数
fscanf函数
功 能:从磁盘文件中按格式读入字符
用 法:int fscanf(FILE *fp, char *format,[argument...]);
示 例:fscanf(fp,"%d,%f",&i,&t);
返回值:
成功返回读入的参数的个数
失败返回EOF(-1)
注 意:fscanf遇到空格和换行时结束,注意空格时也结束。
fprintf函数
功 能:从磁盘文件中按格式输出字符
用 法:int fprintf(FILE *fp, char *format,[argument...]);
示 例:fprintf(fp,"%d,%6.2f",i,t);
返回值:成功返回输出的字符数
失败时返回一个负值.
示例:
- #include <stdio.h>
- #define SIZE 5
- typedef struct
- {
- char name[20];
- char num[15];
- int age;
- }student;
- student stu[SIZE],buf[SIZE];
- int main()
- {
- FILE *fp;
- char filename[50],ch;
- printf("input your name num and age:\n");
- fscanf(stdin,"%s %s %d",&stu[0].name,&stu[0].num,&stu[0].age);//stdin为键盘输入
- printf("Please input your read filename:");
- scanf("%s",filename);
- if(!(fp=fopen(filename,"w")))
- {
- printf("Can not open %s",filename);
- return 1;
- }
- fprintf(fp,"name:%s num:%s age:%d",stu[0].name,stu[0].num,stu[0].age);
- fclose(fp);
- return 0;
- }
- /*
- Alice 001 18
- */
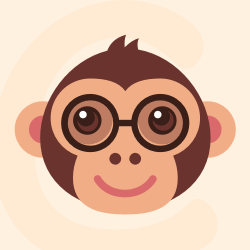



A beautiful web dashboard for Linux
最近提交(Master分支:3 个月前 )
186a802e
added ecosystem file for PM2 4 年前
5def40a3
Add host customization support for the NodeJS version 4 年前
更多推荐
所有评论(0)