Linux open/close函数
linux-dash
A beautiful web dashboard for Linux
项目地址:https://gitcode.com/gh_mirrors/li/linux-dash

·
Linux open/close函数
查看函数
man 2 open
man 2 close
简述
打开文件可能创建并打开文件(open and possibly create a file)
头文件
前两个 unistd.h中包含
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>// 主要定义宏
函数签名
// 第一参数,文件路径;第二个参数,打开方式
int open(const char *pathname, int flags);// 只能打开,不能创建
// 第三个参数(mode_t)所创建的文件权限,受 umask影响
int open(const char *pathname, int flags, mode_t mode);
注:
- 最终创建的文件权限=mode & ~umask;
- mode_t一个4位的八进制数,第一位为0,表示八进制;
- 有mode,就要有O_CREAT;
关闭文件
int close(int fd);
Return Value:
close() returns zero on success. On error, -1 is returned, and errno is set appropriately.
常用flags(file creation flags and file status flags)
三种文件打开方式(三选一):
O_RDONLY(只读)、O_WRONLY(只写)、O_RDWR(读写)
int fd = open("./test.txt", O_RDONLY);
O_APPEND(追加)、O_CREAT(创建)、O_TRUNC(截断)、O_NONBLOCK(非阻塞)
示例:
以读写方式打开当前路径下的test.txt文件,不存在则创建,创建的文件权限为0664(110 110 100);
int fd = open("./test.txt", O_RDWR | O_CREAT, 0664);
返回值(RETURN VALUE)
成功则返回打开文件的文件描述符,失败返回-1,并正确设置errno;
常见错误
- 打开文件不存在
- 以写方式打开只读文件(打开文件没有对应权限)
- 以只写方式打开目录
完整示例(打开文件)
open_test.c
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
int main(int argc, char* argv[]){
// 以只读的方式打开已存在文件
const char* path1 = "./test1.txt";
int fd1 = open(path1, O_RDONLY);
if(fd1 == -1){
perror("Read Error");
return 0;
}
printf("File %s is opened\nfd = %d\n", path1, fd1);
close(fd1);
// 创建并以读写方式打开文件,文件权限设置为0664(不考虑umask)
const char* path2 = "./test2.txt";
int fd2 = open(path2, O_RDWR | O_CREAT, 0664);
if(fd2 == -1){
perror("Open Error");
return 0;
}
printf("File %s is created and opened\nfd = %d\n", path2, fd2);
close(fd2);
return 0;
}
makefile
# get the .c files
src = $(wildcard *.c)
# target that we need
obj = $(patsubst %.c, %, $(src))
# warn
wrning = -wall -g
# target
all:$(obj)
# rules
%:%.c
gcc $< -o $@
# fake file
.PHONY:clean
# clean
clean:
-rm -rf $(obj)
运行结果
文件 test1.txt不存在
文件 test1.txt存在
2020/07/23 13:30
@luxurylu
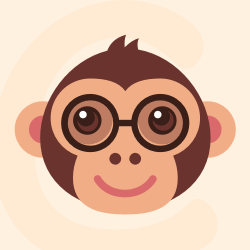



A beautiful web dashboard for Linux
最近提交(Master分支:1 个月前 )
186a802e
added ecosystem file for PM2 4 年前
5def40a3
Add host customization support for the NodeJS version 4 年前
更多推荐
所有评论(0)