C++实操 - 如何在程序中delay一段时间

在程序中运行的都是以进程为单位,进程里面的任务或线程有时需要“sleep”或者进入一个休眠状态一段时间。
在这期间,此线程代码执行被暂停。
当时间到了后,代码恢复执行(或者由信号或中断导致)。
为了使一个程序(进程、任务、线程 / process, task, thread)进入sleep状态,可以使用系统调用。
比如,Linux/Unix下:
#include <unistd.h>
int main( )
{
sleep(3); // sleep 3 seconds
return 0;
}
Windows下:
#include <Windows.h>
int main( )
{
Sleep(3000); // sleep 3000 milliseconds
return 0;
}
如果代码要兼容Linux和Windows平台:
#ifdef _WIN32
#include <Windows.h>
#else
#include <unistd.h>
#endif
函数原型:
unsigned sleep(unsigned seconds)。
参数:
seconds => 程序暂停执行的时间(秒)。
返回值:
0 => 请求的休眠时间之后,函数返回。
如果休眠被信号打断,那么将返回一个不足的休眠时间量(指定的请求时间段减去实际经过的时间)。
说明:
sleep()函数使程序或被调用的进程暂时停止执行,时间由函数参数指定,单位为秒。执行被暂停,直到所要求的时间过后,或有信号或中断传递给该函数。
但是,如果系统调度了任何其他活动,那么暂停时间可能更长。
usleep( ) 函数
在Linux的头文件unistd.h中,还提供了另一个函数 "usleep()",它可以在指定时间内暂停程序的执行。其工作原理与前面介绍的sleep( )函数类似。
函数原型:int usleep(useconds_t useconds)。
参数: useconds => 暂停执行的微秒数
返回值:
0 => usleep已经成功返回。
-1 & errno => 函数执行失败。
说明:
函数usleep()暂停调用线程的执行,时间为参数指定的微秒或有信号传递给暂停的线程导致中断此函数执行。
usleep函数中使用的定时器值的最小单位(精度)可能依赖于特定的实现。
具体例子和上面的sleep函数类似。
------------------------------------------------
Thread Sleep (sleep_for & sleep_until)
在C++11中,提供了专门的函数来实现线程休眠。
有两个函数, 第一个:
std::this_thread::sleep_for
函数原型:
template< class Rep, class Period >
void sleep_for( const std::chrono::duration<Rep, Period>& sleep_duration ) 。
参数: sleep_duration => 睡眠的时间量
返回值:无
描述:
sleep_for()函数定义在头文件<thread>中。sleep_for()函数至少在指定的时间内阻断当前线程的执行,即sleep_duration。
由于调度活动或资源争夺的延迟,这个函数可能会阻塞比指定时间更长的时间。
#include <chrono>
#include <thread>
using namespace std;
int main()
{
this_thread::sleep_for(chrono::milliseconds(2000) ); // sleep for 2s
return 0;
}
注:chrono一般是一个表示时间意思的英语前缀。
第二个:
std::this_thread::sleep_until
函数原型:
template< class Clock, class Duration >
void sleep_until( const std::chrono::time_point<Clock,Duration>& sleep_time );
参数:sleep_time => 线程被阻塞的停止时间。
返回值:无
描述:
这个函数定义在头文件<thread>中。sleep_until( ) 函数会阻塞当前线程的执行,直到到达指定的时间点,恢复执行。
同样的,由于调度活动或资源争夺的延迟,这个函数可能会阻塞比指定时间更长的时间。
#include <chrono>
#include <thread>
using namespace std;
void threadFunc(void)
{
chrono::system_clock::time_point timePt = chrono::system_clock::now() + chrono::seconds(5);
this_thread::sleep_until(timePt);
}
int main()
{
std::thread th(&threadFunc);
th.join();
return 0;
}
$ g++ -o test test.cpp -pthread
上面程序将等待5s后,结束执行。
在C++14中,提供了字面值后缀功能(literal suffixex):
#include <chrono>
#include <thread>
int main() {
using namespace std::this_thread; // sleep_for, sleep_until
using namespace std::chrono_literals; // ns, us, ms, s, h, etc.
using std::chrono::system_clock;
sleep_for(10ns);
sleep_until(system_clock::now() + 1s);
}
/* Error */
$ g++ -o test test.cpp -std=c++11
/* OK */
$ g++ -o test test.cpp -std=c++14
上面这个例子,用到了C++的thread头文件,都没用到Linux中的thread库,所以g++编译时不需要加-pthread。
参考:
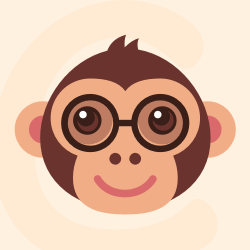



更多推荐
所有评论(0)