嵌入式Linux应用程序开发第2版(多路复用)
linux-dash
A beautiful web dashboard for Linux
项目地址:https://gitcode.com/gh_mirrors/li/linux-dash

·
多路复用
I/O处理的模型5种
阻塞IO
非阻塞IO
IO复用(select和poll)
信号驱动
异步IO(Posix.1 的 aio...系列函数)
IO执行的两个阶段
在Linux中,对于一次读取IO的操作,数据并不会直接拷贝到程序的程序缓冲区。通常包括两个不同阶段:
(1)等待数据准备好,到达内核缓冲区;
(2)从内核向进程复制数据。
对于一个套接字上的输入操作,第一步通常涉及等待数据从网络中到达。当所有等待分组到达时,它被复制到内核中的某个缓冲区。第二步就是把数据从内核缓冲区复制到应用程序缓冲区。
select()和poll()的I/O多路转接模型是处理I/O复用的一个高效的方法
在本实例中,分别用select()函数和poll()函数实现同一功能
1.select()函数
// multiplex_select.c
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <time.h>
#include <errno.h>
#include <string.h>
#define MAX_BUFFER_SIZE 1024 //缓冲区的大小
#define IN_FILES 3 //多路复用输入文件数目
#define TIME_DELAY 60 // 超时秒数
#define MAX(a,b) ((a > b)?(a):(b))
int main()
{
int fds[IN_FILES];
char buf[MAX_BUFFER_SIZE];
int i, res, real_read, maxfd;
struct timeval tv;
fd_set inset, tmp_inset;
//首先以只读非阻塞方式打开两个管道文件
fds[0] = 0;
if((fds[1] = open("in1", O_RDONLY | O_NONBLOCK)) < 0)
{
printf("Open inl error!\n");
return 1;
}
if((fds[2] = open("in2", O_RDONLY | O_NONBLOCK)) < 0)
{
printf("Open in2 error!\n");
return 1;
}
//取出两个文件描述符中的较大者
maxfd = MAX(MAX(fds[0],fds[1]),fds[2]);
//初始化读集合inset,并在读集合中加入相应的描述集
FD_ZERO(&inset);
for(i = 0; i < IN_FILES; i++)
{
FD_SET(fds[i],&inset);
}
FD_SET(0, &inset);
tv.tv_sec = TIME_DELAY;
tv.tv_usec = 0;
//循环测试该文件描述符是否准备就绪,并调用select函数对象相关文件描述符做对应操作
while(FD_ISSET(fds[0], &inset) || FD_ISSET(fds[1], &inset)
|| FD_ISSET(fds[2], &inset))
{
//文件描述符的备份,这样可以避免每次进行初始化
tmp_inset = inset;
res = select(maxfd + 1, &tmp_inset, NULL,NULL, &tv);
switch(res)
{
case -1:
{
printf("Select error!\n");
return 1;
}
break;
case 0: //超时
{
printf("Time out !\n");
return 1;
}
break;
default:
{
for(i = 0; i < IN_FILES; i++)
{
if (FD_ISSET(fds[i], &tmp_inset))
{
memset(buf, 0, MAX_BUFFER_SIZE);
real_read = read(fds[i], buf, MAX_BUFFER_SIZE);
if(real_read < 0)
{
if(errno != EAGAIN)
{
return 1;
}
}
else if (!real_read)
{
close(fds[i]);
FD_CLR(fds[i], &inset);
}
else
{
if (i == 0)
{
//主程序终端控制
if((buf[0] == 'q') || (buf[0] == 'Q'))
{
return 1;
}
}
else
{
//显示管道输入字符串
buf[real_read] = '\0';
printf("%s", buf);
}
}
}
}
}
break;
}
}
return 0;
}
为了充分表现select()调用的功能,在运行主程序的时候,需要打开3个虚拟终端:
首先:用mknod 创建两个管道in1和in2
在另外两个终端上分别运行 cat > in1 和cat > in2
同时在第三个虚拟终端上运行主程序
(在两个管道终端上输入字符串,在主程序上可以观察到同样的内容在虚拟终端上逐行显示,结束主程序输入Q即可)
mknod in1 p
mknod in2 p
cat > in1
cat > in2
./multiplex_select
注
:所有的文件,管道 尽量都在同一目录(文件夹)下,可以避免寻找路径问题
2.poll()
select()函数存在一系列的问题
例如:内核必须检查多余的文件描述符,每次调用select()之后必须重置被监听的文件描述符集,而且可监听的文件个数首先等
实际上,poll机制与select机制相比效率更高,使用范围更广,下面给出用poll()函数实现同样功能的代码
// multiplex_poll.c
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <time.h>
#include <errno.h>
#include <poll.h>
#include <string.h>
#define MAX_BUFFER_SIZE 1024 //缓冲区的大小
#define IN_FILES 3 //多路复用输入文件数目
#define TIME_DELAY 60 // 超时秒数
#define MAX(a,b) ((a > b)?(a):(b))
int main(void)
{
struct pollfd fds[IN_FILES];
char buf[MAX_BUFFER_SIZE];
int i, res, real_read, maxfd;
//首先按照一定的权限打开两个源文件
fds[0].fd = 0;
if((fds[1].fd = open("in1", O_RDONLY |O_NONBLOCK)) < 0)
{
printf("Open in1 error!\n");
return 1;
}
if((fds[2].fd = open("in2", O_RDONLY | O_NONBLOCK)) < 0)
{
printf("Open in2 error!\n");
return 1;
}
//取出两个文件描述符中的较大者
for(i = 0; i < IN_FILES; i++)
{
fds[i].events = POLLIN;
}
//循环测试该文件描述符是否准备就绪,并调用select函数对象相关文件描述符做对应操作
while(fds[0].events || fds[1].events
|| fds[2].events)
{
if(poll(fds, IN_FILES,0) < 0)
{
printf("Poll error!\n");
return 1;
}
for (i = 0; i < IN_FILES; i++)
{
if (fds[i].revents)
{
memset(buf, 0, MAX_BUFFER_SIZE);
real_read = read (fds[i].fd, buf, MAX_BUFFER_SIZE);
if (real_read < 0)
{
if (errno != EAGAIN)
{
return 1;
}
}
else if (!real_read)
{
close(fds[i].fd);
fds[i].events = 0;
}
else
{
if (i == 0)
{
if ((buf[0] == 'q') || (buf[0] == 'Q'))
{
return 1;
}
}
else
{
buf[real_read] = '\0';
printf("%s", buf);
}
}// end of if real_read
} //end of if revents
} //end of for
} //end of while
return 0;
}
运行的结果与select程序类似
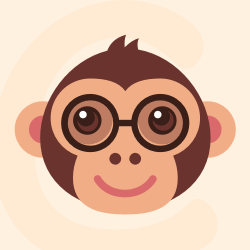



A beautiful web dashboard for Linux
最近提交(Master分支:3 个月前 )
186a802e
added ecosystem file for PM2 4 年前
5def40a3
Add host customization support for the NodeJS version 4 年前
更多推荐
所有评论(0)