linux c hexdump之实现cat的16进制显示数据
linux-dash
A beautiful web dashboard for Linux
项目地址:https://gitcode.com/gh_mirrors/li/linux-dash

·
/*
hexdump.c reversible hexdump
Copyright (c) 1996 by Andreas Leitgeb (AvL) <avl@logic.tuwien.ac.at>
Permission to use, copy, modify, and distribute this software and its
documentation for any purpose and without fee is hereby granted,
provided that the above copyright notice appear in all copies and that
both that copyright notice and this permission notice appear in
supporting documentation.
*/
#include<stdio.h>
#include<stdlib.h>
#include<ctype.h>
#define MAXBPL 10240
int ch[MAXBPL],bpl=16;
long long line=0;
void outputbyte(int i,int max);
/* if the isprint(c) function does not exist on your system
(or for PC/MS-DOS machines) you can try to replace it by
'(c>=32)'
*/
int main(int argc,char *argv[]) {
int c,i,j,diff=1,noabbr=0,flush=0;
char *infname=NULL,*outfname=NULL;
/* Argument handling */
for (i=1 ; i<argc; ++i) {
if (argv[i][0]!='-') {
if (infname) {
fprintf(stderr,"%s: More than one inputfile given.\n",argv[0]);
exit(2);
}
infname=argv[i];
} else {
switch (argv[i][1]) {
case 'i': if (argv[i][2]) goto usage;
if (i+1 == argc) {
fprintf(stderr,"%s: Argument -%c requires a filename given.\n",argv[0],'i');
exit(2);
}
if (infname) {
fprintf(stderr,"%s: More than one %sputfile given.\n",argv[0],"in");
exit(2);
}
infname=argv[i+1]; i++; break;
case 'o': if (argv[i][2]) goto usage;
if (i+1 == argc) {
fprintf(stderr,"%s: Argument -%c requires a filename given.\n",argv[0],'o');
exit(2);
}
if (outfname) {
fprintf(stderr,"%s: More than one %sputfile given.\n",argv[0],"out");
exit(2);
}
outfname=argv[i+1]; i++; break;
case 'w': if (argv[i][2]) goto usage;
if (i+1 == argc) {
fprintf(stderr,"%s: Argument -%c requires a number given.\n",argv[0],'w');
exit(2);
}
bpl=atoi(argv[i+1]); i++;
if (bpl < 1 || bpl >MAXBPL ) {
fprintf(stderr,"%s: invalid argument. Valid range is 1-%d.\n",argv[0],MAXBPL);
exit(2);
}
break;
case 'n': // old option
case 'v': if (argv[i][2]) goto usage;
noabbr=1; break;
case 'f': if (argv[i][2]) goto usage;
flush=1; break;
default:
usage:
fprintf(stderr,"Usage: %s [-w #] [[-i] inputfile] [-o outfile] [-v]\n",argv[0]);
fprintf(stderr," if in/outfile are omitted, stdin/stdout are used instead\n");
fprintf(stderr," -v: repeating lines will NOT be abbreviated (no '*'-lines).\n");
fprintf(stderr," -f: show each incoming byte. (implies -v) \n");
fprintf(stderr," -w #: bytes per line (default: 16)\n");
exit(2);
}
}
}
if (infname && !freopen(infname,"rb",stdin)) {
fprintf(stderr,"%s: Cannot open %s for reading.\n",argv[0],infname);
perror(argv[0]);
exit(1);
}
if (outfname && !freopen(outfname,"w",stdout)) {
fprintf(stderr,"%s: Cannot open %s for writing.\n",argv[0],outfname);
perror(argv[0]);
exit(1);
}
if (flush) { noabbr=1; }
/*
three states:
diff==1 : usual state: hexdump a chunk of 16 Bytes
diff==2 : this line is the first repetition of the previous line.
output "*\n"
diff==0 : more repeating lines: nothing to do.
*/
i=0; line=0;
while(!feof(stdin) && ((c=fgetc(stdin))!=EOF)){
if (ch[i]!=c) { ch[i]=c; diff=1;}
if (flush) {
outputbyte(i,bpl);
fflush(stdout);
} else if (i+1==bpl) { /* chunk complete */
if (diff==1 || noabbr) {
for(j=0;j<bpl;j++){
outputbyte(j,bpl);
}
diff=2;
} else if (diff==2) { printf("*\n"); diff=0; }
}
i++; if (i>=bpl) { line+=i; i=0; }
}
if (i) { j=i;
/* if flush was on, then `i' bytes have already been output */
if (!flush) { j=0; } /* otherwise, they still need to ! */
for(;j<bpl;j++){
outputbyte(j,i);
}
line+=i;
}
printf("%08llx;\n",line);
return 0;
}
void outputbyte(int i, int max) { int c=ch[i];
/* reads global variable: line */
if (i == 0) { printf("%08llx: ",line); }
printf( (i<max) ? "%2.2X " : " " ,c);
if (bpl==16 && i==7) { printf("- "); }
if (i+1==bpl) {
putchar('|');
for(i=0;i<max;i++){ c=ch[i];
putchar( (isprint(c)) ? c : ' ');
}
puts("|");
}
}
http://avl.enemy.org/utils/hextools/
gcc -o hexdump.exe hexdump.c
即可
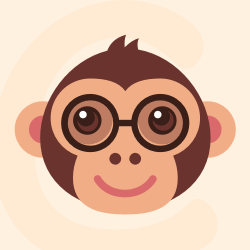



A beautiful web dashboard for Linux
最近提交(Master分支:2 个月前 )
186a802e
added ecosystem file for PM2 4 年前
5def40a3
Add host customization support for the NodeJS version 4 年前
更多推荐
所有评论(0)