Linux下使用Shell脚本删除一个目录下的所有子目录和文件
linux-dash
A beautiful web dashboard for Linux
项目地址:https://gitcode.com/gh_mirrors/li/linux-dash

·
#!/bin/sh
#FileName:deleteDir.sh
#Function:Linux下使用Shell脚本删除一个目录下的所有子目录和文件(不可恢复删除且目录下目录名和文件名中没有空格)
#Version:V0.1
#Author:Sunrier
#Date:2012-08-01
CURRPATH=$PWD
#DESTPATH为删除的目标目录(目标目录本身不会删除)
DESTPATH="/home/Sunrier/Trash"
#isNullDir函数判断进入的目录下是否存在下级子目录或者文件
#存在返回1,不存在返回0
isNullDir()
{
local NewCurrentPath=$PWD
local NewDirName=$1
cd $NewDirName
local NewFileList=`ls 2>/dev/null`
# -n string : 如果字符串不为空,则结果为真
if [ -n "$NewFileList" ]
then
echo "目录$NewDirName下列表信息为$NewFileList"
cd $NewCurrentPath
return 1
else
echo "目录$NewDirName为空目录"
cd $NewCurrentPath
return 0
fi
}
if [ "Sunrier" != "$LOGNAME" ]
then
echo "您没有执行权限!请联系管理员!"
exit 1
fi
cd $DESTPATH 2>/dev/null
if [ $? -ne 0 ]
then
echo "没有找到目标目录!"
exit 1
fi
echo "您正准备删除的目录为$DESTPATH"
#不考虑隐藏目录和文件
FileList=`ls 2>/dev/null`
while [ "" != "$FileList" ]
do
echo "当前列表信息为 $FileList"
for pFile in $FileList
do
echo "加载 ${pFile} 中......"
if [ -d "${pFile}" ]
then
echo "检查到 ${pFile} 为目录 "
echo "目录 ${pFile} 处理中......"
#注:在Linux的Shell脚本中,调用函数作为判断条件时,函数返回值为0时,if后的条件才为真;否则if后的条件为假!
if isNullDir "${pFile}"
then
echo "删除目录 ${pFile} 中......"
rm -rf ${pFile}
else
echo "移动目录${pFile}下的所有目录和文件中......"
mv ${pFile}/* . >/dev/null 2>&1
if [ $? -ne 0 ]
then
ModifyTime=`date +%Y%m%d%H%M%S`
mv ${pFile} $ModifyTime
fi
fi
else
echo "检查到 ${pFile} 为文件 "
echo "正在把文件 ${pFile} 的文件名更改为文件名 1 中......"
mv ${pFile} 1 2>/dev/null
fi
if [ -f 1 ]
then
echo "发现文件名为1的文件,正在删除文件1中......"
echo "123456"> 1
rm -rf 1
fi
done
echo "更新新的文件列表中......"
cd $DESTPATH
FileList=`ls 2>/dev/null`
done
if [ $? -eq 0 ]
then
echo "清理成功!"
cd $CURRPATH
exit 0
else
echo "清理失败!"
cd $CURRPATH
exit 1
fi
注:去掉一些显示信息
#!/bin/sh
#FileName:deleteDir.sh
#Function:Linux下使用Shell脚本删除一个目录下的所有子目录和文件(不可恢复删除且目录下目录名和文件名中没有空格)
#Version:V0.2
#Author:Sunrier
#Date:2012-08-27
CURRPATH=$PWD
#DESTPATH为删除的目标目录(目标目录本身不会删除)
DESTPATH="/home/Sunrier/Trash"
#isNullDir函数判断进入的目录下是否存在下级子目录或者文件
#存在返回1,不存在返回0
isNullDir()
{
local NewCurrentPath=$PWD
local NewDirName=$1
cd $NewDirName
local NewFileList=`ls 2>/dev/null`
# -n string : 如果字符串不为空,则结果为真
if [ -n "$NewFileList" ]
then
cd $NewCurrentPath
return 1
else
cd $NewCurrentPath
return 0
fi
}
if [ "Sunrier" != "$LOGNAME" ]
then
echo "您没有执行权限!请联系管理员!"
exit 1
fi
cd $DESTPATH 2>/dev/null
if [ $? -ne 0 ]
then
echo "没有找到目标目录!"
exit 1
fi
echo "您正准备删除的目录为$DESTPATH"
#不考虑隐藏目录和文件
FileList=`ls 2>/dev/null`
while [ "" != "$FileList" ]
do
for pFile in $FileList
do
echo "加载 ${pFile} 中......"
if [ -d "${pFile}" ]
then
#注:在Linux的Shell脚本中,调用函数作为判断条件时,函数返回值为0时,if后的条件才为真;否则if后的条件为假!
if isNullDir "${pFile}"
then
rm -rf ${pFile}
else
mv ${pFile}/* . >/dev/null 2>&1
if [ $? -ne 0 ]
then
ModifyTime=`date +%Y%m%d%H%M%S`
mv ${pFile} $ModifyTime
fi
fi
else
mv ${pFile} 1 2>/dev/null
fi
if [ -f 1 ]
then
echo "123456">1
rm -rf 1
fi
done
cd $DESTPATH
FileList=`ls 2>/dev/null`
done
if [ $? -eq 0 ]
then
echo "清理成功!"
cd $CURRPATH
exit 0
else
echo "清理失败!"
cd $CURRPATH
exit 1
fi
//解决目录下目录名和文件名中含有空格的情况
#!/bin/sh
#FileName:deleteDir.sh
#Function:Linux下使用Shell脚本删除一个目录下的所有子目录和文件(不可恢复删除)
#Version:V0.3
#Author:Sunrier
#Date:2012-08-29
CURRPATH=$PWD
#DESTPATH为删除的目标目录(目标目录本身不会删除)
DESTPATH="/home/Sunrier/Trash"
#isNullDir函数判断进入的目录下是否存在下级子目录或者文件
#存在返回1,不存在返回0
isNullDir()
{
local NewCurrentPath=$PWD
local NewDirName=$1
cd "$NewDirName"
local NewFileList=`ls 2>/dev/null`
# -n string : 如果字符串不为空,则结果为真
if [ -n "$NewFileList" ]
then
echo "目录$NewDirName下列表信息为$NewFileList"
cd $NewCurrentPath
return 1
else
echo "目录$NewDirName为空目录"
cd $NewCurrentPath
return 0
fi
}
if [ "Sunrier" != "$LOGNAME" ]
then
echo "您没有执行权限!请联系管理员!"
exit 1
fi
cd $DESTPATH 2>/dev/null
if [ $? -ne 0 ]
then
echo "没有找到目标目录!"
exit 1
fi
echo "您正准备删除的目录为$DESTPATH"
#不考虑隐藏目录和文件
#FileList=`ls 2>/dev/null`
FileList=`ls | tr " " "?" 2>/dev/null`
while [ "" != "$FileList" ]
do
echo "当前列表信息为 $FileList"
for pFile in $FileList
do
echo "加载 ${pFile} 中......"
if [ -d "${pFile}" ]
then
echo "检查到 ${pFile} 为目录 "
echo "目录 ${pFile} 处理中......"
#注:在Linux的Shell脚本中,调用函数作为判断条件时,函数返回值为0时,if后的条件才为真;否则if后的条件为假!
if isNullDir "${pFile}"
then
echo "删除目录 ${pFile} 中......"
rm -rf "${pFile}"
else
echo "移动目录${pFile}下的所有目录和文件中......"
mv "${pFile}"/* . >/dev/null 2>&1
if [ $? -ne 0 ]
then
echo "发现父目录与子目录同名,试图更改父目录目录名......"
ModifyTime=`date +%Y%m%d%H%M%S`
mv "${pFile}" $ModifyTime
fi
fi
else
echo "检查到 ${pFile} 为文件 "
echo "正在把文件 ${pFile} 的文件名更改为文件名 1 中......"
mv "${pFile}" 1 2>/dev/null
fi
if [ -f 1 ]
then
echo "发现文件名为1的文件,正在删除文件1中......"
echo "123456" > 1
rm -rf 1
fi
done
echo "更新新的文件列表中......"
cd $DESTPATH
#FileList=`ls 2>/dev/null`
FileList=`ls | tr " " "?" 2>/dev/null`
done
if [ $? -eq 0 ]
then
echo "清理成功!"
cd $CURRPATH
exit 0
else
echo "清理失败!"
cd $CURRPATH
exit 1
fi
//去掉一些显示信息
#!/bin/sh
#FileName:deleteDir.sh
#Function:Linux下使用Shell脚本删除一个目录下的所有子目录和文件(不可恢复删除)
#Version:V0.4
#Author:Sunrier
#Date:2012-08-29
CURRPATH=$PWD
#DESTPATH为删除的目标目录(目标目录本身不会删除)
DESTPATH="/home/Sunrier/Trash"
#isNullDir函数判断进入的目录下是否存在下级子目录或者文件
#存在返回1,不存在返回0
isNullDir()
{
local NewCurrentPath=$PWD
local NewDirName=$1
cd "$NewDirName"
local NewFileList=`ls 2>/dev/null`
# -n string : 如果字符串不为空,则结果为真
if [ -n "$NewFileList" ]
then
cd $NewCurrentPath
return 1
else
cd $NewCurrentPath
return 0
fi
}
if [ "Sunrier" != "$LOGNAME" ]
then
echo "您没有执行权限!请联系管理员!"
exit 1
fi
cd $DESTPATH 2>/dev/null
if [ $? -ne 0 ]
then
echo "没有找到目标目录!"
exit 1
fi
echo "您正准备删除的目录为$DESTPATH"
#不考虑隐藏目录和文件
FileList=`ls | tr " " "?" 2>/dev/null`
while [ "" != "$FileList" ]
do
for pFile in $FileList
do
echo "加载 ${pFile} 中......"
if [ -d "${pFile}" ]
then
#注:在Linux的Shell脚本中,调用函数作为判断条件时,函数返回值为0时,if后的条件才为真;否则if后的条件为假!
if isNullDir "${pFile}"
then
rm -rf "${pFile}"
else
mv "${pFile}"/* . >/dev/null 2>&1
if [ $? -ne 0 ]
then
ModifyTime=`date +%Y%m%d%H%M%S`
mv "${pFile}" $ModifyTime
fi
fi
else
mv "${pFile}" 1 2>/dev/null
fi
if [ -f 1 ]
then
echo "123456" > 1
rm -rf 1
fi
done
cd $DESTPATH
FileList=`ls | tr " " "?" 2>/dev/null`
done
if [ $? -eq 0 ]
then
echo "清理成功!"
cd $CURRPATH
exit 0
else
echo "清理失败!"
cd $CURRPATH
exit 1
fi
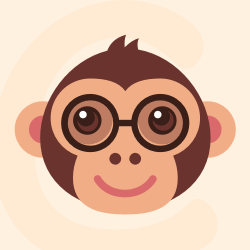



A beautiful web dashboard for Linux
最近提交(Master分支:2 个月前 )
186a802e
added ecosystem file for PM2 4 年前
5def40a3
Add host customization support for the NodeJS version 4 年前
更多推荐
所有评论(0)