用C写蓝牙通讯程序:扫描、读取、发送
linux-dash
A beautiful web dashboard for Linux
项目地址:https://gitcode.com/gh_mirrors/li/linux-dash

·
在Linux下,通过bluez 蓝牙库可以用C语言轻松实现蓝牙通信。在ubuntu下可以用 apt-get install libbluetooth-dev 安装该库。下面是几个简单示例。
一个简单的扫描程序,得到周边的蓝牙从机设备名和地址:
读和写示例:
一个简单的扫描程序,得到周边的蓝牙从机设备名和地址:
- #include <stdio.h>
- #include <stdlib.h>
- #include <unistd.h>
- #include <sys/socket.h>
- #include <bluetooth/bluetooth.h>
- #include <bluetooth/hci.h>
- #include <bluetooth/hci_lib.h>
-
- int main(int argc, char **argv)
- {
- inquiry_info *ii = NULL;
- int max_rsp, num_rsp;
- int dev_id, sock, len, flags;
- int i;
- char addr[19] = { 0 };
- char name[248] = { 0 };
-
- dev_id = hci_get_route(NULL);
- sock = hci_open_dev( dev_id );
- if (dev_id < 0 || sock < 0) {
- perror("opening socket");
- exit(1);
- }
-
- len = 8;
- max_rsp = 255;
- flags = IREQ_CACHE_FLUSH;
- ii = (inquiry_info*)malloc(max_rsp * sizeof(inquiry_info));
-
- num_rsp = hci_inquiry(dev_id, len, max_rsp, NULL, &ii, flags);
- if( num_rsp < 0 ) perror("hci_inquiry");
-
- for (i = 0; i < num_rsp; i++) {
- ba2str(&(ii+i)->bdaddr, addr);
- memset(name, 0, sizeof(name));
- if (hci_read_remote_name(sock, &(ii+i)->bdaddr, sizeof(name),
- name, 0) < 0)
- strcpy(name, "[unknown]");
- printf("%s %s\n", addr, name);
- }
-
- free( ii );
- close( sock );
- return 0;
- }
读和写示例:
- #include <stdio.h>
- #include <unistd.h>
- #include <sys/socket.h>
- #include <bluetooth/bluetooth.h>
- #include <bluetooth/rfcomm.h>
-
- int main(int argc, char **argv)
- {
- struct sockaddr_rc addr = { 0 };
- int s, status, len=0;
- char dest[18] = "00:12:01:31:01:13";
- char buf[256];
- // allocate a socket
- s = socket(AF_BLUETOOTH, SOCK_STREAM, BTPROTO_RFCOMM);
-
- // set the connection parameters (who to connect to)
- addr.rc_family = AF_BLUETOOTH;
- addr.rc_channel = (uint8_t) 1;
- str2ba( dest, &addr.rc_bdaddr );
-
- // connect to server
- status = connect(s, (struct sockaddr *)&addr, sizeof(addr));
-
-
- if(status){
- printf(" failed to connect the device!\n");
- return -1;
- }
-
-
- do{
- len = read(s, buf, sizeof buf);
-
- if( len>0 ) {
- buf[len]=0;
- printf("%s\n",buf);
- write(s, buf, strlen(buf));
- }
- }while(len>0);
-
- close(s);
- return 0;
- }
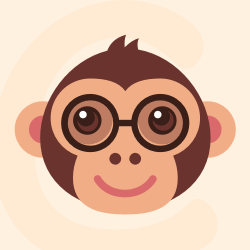



A beautiful web dashboard for Linux
最近提交(Master分支:2 个月前 )
186a802e
added ecosystem file for PM2 4 年前
5def40a3
Add host customization support for the NodeJS version 4 年前
更多推荐
所有评论(0)