Java如何获取系统CPU、内存、硬盘使用情况(仅限windows,Linux)
linux-dash
A beautiful web dashboard for Linux
项目地址:https://gitcode.com/gh_mirrors/li/linux-dash

·
1.首先引入sigar依赖
<dependency>
<groupId>org.fusesource</groupId>
<artifactId>sigar</artifactId>
<version>1.6.4</version>
</dependency>
2.需要引入sigar的动态链接库 ,Windows放在jdk的bin下,Linux放在usr/lib64下,https://pan.baidu.com/s/1MXCypf1yNYu75ZLP1mz0gA ,提取码 :tzx9
3.代码
import org.hyperic.sigar.*;
import java.io.*;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
/**
* 获取系统CPU、内存、硬盘使用情况
*
* @author yanzy
* @date 2019/1/16 9:45
*/
public class SystemInfo {
public static void main(String[] args) {
try {
// Windows放在jdk的bin下,Linux放在usr/lib64下
System.out.println("位置:" + System.getProperty("java.library.path"));
SystemInfo systemInfo=new SystemInfo();
System.out.println(systemInfo.getCpuUsage());
System.out.println(systemInfo.getMemoryUsage());
System.out.println(systemInfo.getDiskIoUsage());
System.out.println(systemInfo.getDiskUsage());
System.out.println(systemInfo.path());
} catch (Exception e) {
e.getStackTrace();
}
}
/**
* 获取内存使用率
*
* @return java.lang.String
* @author yanzy
* @version 1.0
* @date 2019/1/16 16:56
*/
public String getMemoryUsage() throws SigarException {
Sigar sigar = new Sigar();
Mem mem = sigar.getMem();
// 获取总内存数
Long total = mem.getTotal() / 1024L;
// 获取已使用内存数
Long used = mem.getUsed() / 1024L;
// 取到百分比
Long percentile = total / 100;
return "内存使用率:" + used / percentile + "%";
}
/**
* 获取cpu使用率
*
* @return java.lang.String
* @author yanzy
* @version 1.0
* @date 2019/1/16 16:57
*/
public String getCpuUsage() throws SigarException {
Sigar sigar = new Sigar();
return "CPU的使用率: " + CpuPerc.format(sigar.getCpuPerc().getCombined());
}
/**
* 获取磁盘使用率
*
* @return java.util.List<java.lang.String>
* @author yanzy
* @version 1.0
* @date 2019/1/16 16:57
*/
public List<String> getDiskUsage() throws Exception {
Sigar sigar = new Sigar();
List<String> stringList = new ArrayList<>();
FileSystem[] fslist = sigar.getFileSystemList();
for (int i = 0; i < fslist.length; i++) {
FileSystem fileSystem = fslist[i];
FileSystemUsage usage = sigar.getFileSystemUsage(fileSystem.getDirName());
double usePercent = usage.getUsePercent() * 100D;
stringList.add(fileSystem.getDevName() + "磁盘的使用率:" + (int) usePercent + "%");
}
return stringList;
}
/**
* 获取当前磁盘IO的使用情况
*
* @return java.lang.String
* @author yanzy
* @version 1.0
* @date 2019/1/17 18:31
*/
public String getDiskIoUsage() throws Exception {
// 获取当前每秒的读写量
long tempTotal = getTotalByte();
Thread.sleep(1000);
long total = getTotalByte();
// 字节转成kb
double cha = (double) (total - tempTotal) / 1024;
// 转成MB,保留1位小数
BigDecimal bigDecimal = new BigDecimal(cha / 1024);
double value = bigDecimal.setScale(2, BigDecimal.ROUND_HALF_UP).doubleValue();
return "磁盘IO的使用情况:" + value + " MB/S";
}
/**
* 获取当前磁盘IO的读写数量
*
* @return long
* @author yanzy
* @version 1.0
* @date 2019/1/17 16:11
*/
public long getTotalByte() {
Sigar sigar = new Sigar();
long totalByte = 0;
try {
FileSystem[] fslist = sigar.getFileSystemList();
for (int i = 0; i < fslist.length; i++) {
if (fslist[i].getType() == 2) {
FileSystemUsage usage = sigar.getFileSystemUsage(fslist[i].getDirName());
totalByte += usage.getDiskReadBytes();
totalByte += usage.getDiskWriteBytes();
}
}
} catch (SigarException e) {
e.printStackTrace();
}
return totalByte;
}
/**
* 获取当前项目的磁盘使用情况
*
* @return java.lang.String
* @author yanzy
* @version 1.0
* @date 2019/1/21 10:34
*/
public String path() throws Exception {
String path = getClass().getResource("/").getFile();
if (path.contains("/") && path.contains(":")) {
path = path.replace("/", "").substring(0, path.indexOf(":"));
}
for (String disk : this.getDiskUsage()) {
if (disk.contains(path) || disk.contains("rootfs")) {
return "当前服务所在磁盘的使用率:" + disk;
}
}
return "未找到相应的磁盘";
}
/**
* Linux下获取磁盘IO使用率
*
* @return float
* @author yanzy
* @version 1.0
* @date 2019/1/17 10:07
*/
public float getHdIOpPercent() {
System.out.println("开始收集磁盘IO使用率");
float ioUsage = 0.0f;
Process pro = null;
Runtime runtime = Runtime.getRuntime();
try {
String command = "iostat -d -x";
pro = runtime.exec(command);
BufferedReader in = new BufferedReader(new InputStreamReader(pro.getInputStream()));
String line = null;
int count = 0;
while ((line = in.readLine()) != null) {
if (++count >= 4) {
String[] temp = line.split("\\s+");
if (temp.length > 1) {
float util = Float.parseFloat(temp[temp.length - 1]);
ioUsage = (ioUsage > util) ? ioUsage : util;
}
}
}
if (ioUsage > 0) {
System.out.println("本节点磁盘IO使用率为: " + ioUsage);
ioUsage /= 100;
}
in.close();
pro.destroy();
} catch (IOException e) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
System.out.println("IoUsage发生InstantiationException. " + e.getMessage());
System.out.println(sw.toString());
}
return ioUsage;
}
}
不引用第三方依赖获取系统信息,https://blog.csdn.net/qq_39486119/article/details/100043309
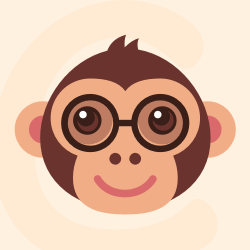



A beautiful web dashboard for Linux
最近提交(Master分支:2 个月前 )
186a802e
added ecosystem file for PM2 4 年前
5def40a3
Add host customization support for the NodeJS version 4 年前
更多推荐
所有评论(0)