SpringBoot整合Web开发:返回JSON数据

本文将通过一个简单的例子,来讲述如何返回JSON数据。
主要是靠@ResponseBody注解。
1.默认实现:
Spring MVC使用消息转换器HttpMessageConverter对JSON的转换提供了很好的支持。
SpringBoot中,添加Web依赖,这个依赖默认加入了jackson-databind作为json处理器。然后就不需要添加额外的JSON处理器就能返回一段JSON了。
2.代码实现:
person.java:
package com.example.myjson;
import com.fasterxml.jackson.annotation.JsonFormat;
import java.util.Date;
public class Person {
/**姓名*/
private String name;
/**年龄*/
private int age;
/**性别*/
private String sex;
/**日期*/
@JsonFormat(pattern = "yyyy-MM-dd")
private Date nowTime;
/**个性签名*/
private String personalWords;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public Date getNowTime() {
return nowTime;
}
public void setNowTime(Date nowTime) {
this.nowTime = nowTime;
}
public String getPersonalWords() {
return personalWords;
}
public void setPersonalWords(String personalWords) {
this.personalWords = personalWords;
}
}
PersonController.java:
package com.example.myjson;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
//@Controller
//@RestController = @Controller + @ResponseBody
@RestController
public class PersonController {
@GetMapping("/person")
// @ResponseBody可以放到类上,起到对全局方法配置返回json
public List<Person> person(){
List<Person> persons = new ArrayList<>();
Person person1 = new Person();
person1.setName("尹磊");
person1.setAge(21);
person1.setSex("男");
person1.setNowTime(new Date());
person1.setPersonalWords("我爱咱们的国呀,但是谁爱我呀。——《茶馆》常四爷");
Person person2 = new Person();
person2.setName("尹伟");
person2.setAge(19);
person2.setSex("男");
person2.setNowTime(new Date());
person2.setPersonalWords("阳光帅气男孩。");
persons.add(person1);
persons.add(person2);
return persons;
}
}
3.运行并用Postman接口测试工具查看(浏览器也行,随你)
只不过现在是死接口。
这是SpringBoot自带的处理方式。采用这种方式,那么对于字段忽略、日期格式化等需求可以通过注解来解决。
4.自定义转换器:
上述默认的是通过Spring提供的MappingJackson2HttpMessageConverter来实现的。也可以自定义JSON转换器。
GSON和fasejson,
(1).使用GSON:
使用GSON,需要先去掉默认的jackson-databind,然后加入Gson依赖。
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
</dependency>
SpringBoot默认提供了GSON自动转换类GsonHttpMessageConvertersConfiguration.因此依赖添加后就可以直接使用GSON。
但是如果想对日期数据进行格式化,还需要自定义HttpMessageConverter。
查看GsonHttpMessageConvertersConfiguration的源码可知道:
@ConditionalOnMissingBean表示当前项目没有提供GsonHttpMessageConverter时才使用默认的GsonHttpMessageConverter.所以只需要提供一个GsonHttpMessageConverter即可。
(2).使用fastjson:
不同于GSON,fastjson集成依赖后不能立即用,需要提供相应的HttpMessageConverter才能使用。(即使用和配置FastJsonHttpMessageConverter。或者web依赖又依赖了spring-boot-autoconfigure,在这个自动化配置中,有一个WebMveAutoConfiguration类提供了对SpringMVC最基本的配置。如果某一项自动化配置不满足开发要求,可以自定义配置针对该项,只需要实现WebMvcConfigurer接口即可,并重写configureMessageConverters())
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
</dependency>
对于可能中文乱码:
在application.properties中添加:
spring.http.encoding.force-response=true
补充:
当项目中没有GsonHttpMessageConverter时,Springboot会自动提供一个GsonHttpMessageConverter.此时重写configureMessageConverters(),参数converters已经有GsonHttpMessageConverter实例了,选哟替换已有的GsonHttpMessageConverter实例,操作麻烦。所以对于GSON,还是推荐直接使用GsonHttpMessageConverter。
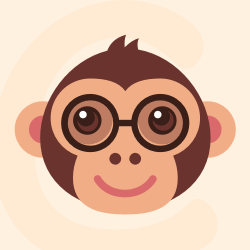



更多推荐
所有评论(0)