将子节点中含子节点的json数据转换成ztree适合的json数据格式
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
源数据格式:
{
"code": 10000,
"msg": "SUCCESS",
"data": [
{
"child": [
{
"child": [
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "字母",
"menuId": 6,
"seq": 0,
"id": 1635
},
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "查字典",
"menuId": 6,
"seq": 19,
"id": 1654
}
],
"file": null,
"resKnowledgeMenu": null,
"pointName": "字词",
"menuId": 6,
"seq": 0,
"id": 1634
},
{
"child": [
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "造句",
"menuId": 6,
"seq": 0,
"id": 1656
},
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "语气",
"menuId": 6,
"seq": 20,
"id": 1676
}
],
"file": null,
"resKnowledgeMenu": null,
"pointName": "句子",
"menuId": 6,
"seq": 1,
"id": 1655
},
{
"child": [
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "诗词默写",
"menuId": 6,
"seq": 0,
"id": 1678
}
],
"file": null,
"resKnowledgeMenu": null,
"pointName": "识字",
"menuId": 6,
"seq": 2,
"id": 1677
},
{
"child": [
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "口语交际,情景写话",
"menuId": 6,
"seq": 0,
"id": 1683
},
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "综合读写",
"menuId": 6,
"seq": 2,
"id": 1685
}
],
"file": null,
"resKnowledgeMenu": null,
"pointName": "语言表达及应用",
"menuId": 6,
"seq": 3,
"id": 1682
},
{
"child": [
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "诗词鉴赏",
"menuId": 6,
"seq": 0,
"id": 1687
},
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "现代文阅读",
"menuId": 6,
"seq": 2,
"id": 1689
}
],
"file": null,
"resKnowledgeMenu": null,
"pointName": "阅读理解及鉴赏",
"menuId": 6,
"seq": 4,
"id": 1686
},
{
"child": [
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "看图写话",
"menuId": 6,
"seq": 0,
"id": 1691
},
{
"child": null,
"file": null,
"resKnowledgeMenu": null,
"pointName": "大作文",
"menuId": 6,
"seq": 3,
"id": 1694
}
],
"file": null,
"resKnowledgeMenu": null,
"pointName": "写作",
"menuId": 6,
"seq": 5,
"id": 1690
}
],
"file": null,
"resKnowledgeMenu": null,
"pointName": "基础知识积累与运用",
"menuId": 6,
"seq": 29,
"id": 1633
}
]
}
目标数据格式:
{
"data": [
{
"checked": false,
"id": 2742,
"name": "基础知识",
"open": true,
"pId": 0,
"seq": 62
},
{
"checked": false,
"id": 2743,
"name": "词汇",
"open": true,
"pId": 2742,
"seq": 0
},
{
"checked": false,
"id": 2797,
"name": "其他作文",
"open": true,
"pId": 2793,
"seq": 3
}
],
"msg": {
"message": "查询成功!",
"status": 0
}
}
程序代码入口:
package com.sdzn.util;
import com.alibaba.fastjson.JSON;
import com.sdzn.enums.MsgCode;
import com.sdzn.thrift.dubbo.vo.Msg;
import com.sdzn.vo.tree.*;
import java.util.ArrayList;
import java.util.List;
/**
* TreeUtil 功能描述:树的格式转换,转换为zTree适合的格式
*
* @author RickyLee【lsr@sdzn.com.cn】
* @date 2017/4/13 9:42
*/
public class TreeUtil {
/**
* 将资源平台返回的数据转换为本平台需要的数据格式
* @param data 资源平台的json数据
* @return
*/
public zTreeResult parseTree(String data){
zTreeResult result=new zTreeResult();
Msg msg=new Msg();
ZyptTree tree= JSON.parseObject(data,ZyptTree.class);
if(tree.getCode()==10000){
msg.setStatus(MsgCode.SUCCESS);
msg.setMessage("查询成功!");
result.setMsg(msg);
result.setData(convertZyptTreeToZTree(tree.getData()));
}else{
msg.setStatus(MsgCode.FAIL);
msg.setMessage("资源平台数据查询出错!");
result.setMsg(msg);
}
return result;
}
/**
* 转换资源平台树的数据部分
* @param datas
* @return
*/
private List<zTreeVO> convertZyptTreeToZTree(List<Data> datas) {
List<zTreeVO> list=new ArrayList<zTreeVO>();
for(Data data:datas){
zTreeVO vo=new zTreeVO();
vo.setId(data.getId());
vo.setName(data.getPointName());
vo.setPId(0);
vo.setSeq(data.getSeq());
list.add(vo);
if(data.getChild()!=null){
list=putChildToList(vo.getId(),data.getChild(),list);
}
}
return list;
}
/**
* 递归转换子节点
* @param pId 父节点
* @param child 子节点集合
* @param list 存放ztree节点集合的全局list
* @return
*/
private List<zTreeVO> putChildToList(Integer pId, List<Child> child, List<zTreeVO> list) {
for(Child data:child){
zTreeVO vo=new zTreeVO();
vo.setId(data.getId());
vo.setName(data.getPointName());
vo.setPId(pId);
vo.setSeq(data.getSeq());
list.add(vo);
if(data.getChild()!=null){
list=putChildToList(vo.getId(),data.getChild(),list);
}
}
return list;
}
public static void main(String[] args) {
String data="{\n" +
" \"code\": 10000,\n" +
" \"msg\": \"SUCCESS\",\n" +
" \"data\": [\n" +
" {\n" +
" \"child\": [\n" +
" {\n" +
" \"child\": [\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"字音\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 0,\n" +
" \"id\": 2744\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"字形\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 1,\n" +
" \"id\": 2745\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"词性\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 2,\n" +
" \"id\": 2747\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"辨析解释字义、词义\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 3,\n" +
" \"id\": 2746\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"感情色彩\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 4,\n" +
" \"id\": 2748\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"构词方式\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 5,\n" +
" \"id\": 2749\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"短语的结构\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 6,\n" +
" \"id\": 2750\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"成语\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 7,\n" +
" \"id\": 2751\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"正确使用词语、熟语\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 8,\n" +
" \"id\": 2752\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"歇后语,谚语\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 9,\n" +
" \"id\": 2753\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"关联词语\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 10,\n" +
" \"id\": 2754\n" +
" }\n" +
" ],\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"词汇\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 0,\n" +
" \"id\": 2743\n" +
" },\n" +
" {\n" +
" \"child\": [\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"标点符号\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 0,\n" +
" \"id\": 2756\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"语句停顿\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 1,\n" +
" \"id\": 2757\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"语气\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 2,\n" +
" \"id\": 2758\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"修辞方法\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 3,\n" +
" \"id\": 2759\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"连词成句\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 4,\n" +
" \"id\": 2760\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"排列句子顺序\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 5,\n" +
" \"id\": 2761\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"病句辨析\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 6,\n" +
" \"id\": 2762\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"选用,仿用,变换句式\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 7,\n" +
" \"id\": 2763\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"划分句子成分及复句关系\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 8,\n" +
" \"id\": 2764\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"对联\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 9,\n" +
" \"id\": 2765\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"补充句子\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 10,\n" +
" \"id\": 2766\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"句式\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 11,\n" +
" \"id\": 2767\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"说明方法\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 12,\n" +
" \"id\": 2768\n" +
" }\n" +
" ],\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"句子\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 1,\n" +
" \"id\": 2755\n" +
" },\n" +
" {\n" +
" \"child\": [\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"文言实词\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 0,\n" +
" \"id\": 2770\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"文言虚词\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 1,\n" +
" \"id\": 2771\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"词类活用\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 2,\n" +
" \"id\": 2772\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"古今异义\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 3,\n" +
" \"id\": 2773\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"通假字\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 4,\n" +
" \"id\": 2774\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"一词多义\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 5,\n" +
" \"id\": 2775\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"文言句式\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 6,\n" +
" \"id\": 2776\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"翻译句子\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 7,\n" +
" \"id\": 2777\n" +
" }\n" +
" ],\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"古代汉语知识\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 2,\n" +
" \"id\": 2769\n" +
" },\n" +
" {\n" +
" \"child\": [\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"文学常识\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 0,\n" +
" \"id\": 2779\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"诗文默写\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 1,\n" +
" \"id\": 2780\n" +
" }\n" +
" ],\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"识记\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 3,\n" +
" \"id\": 2778\n" +
" }\n" +
" ],\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"基础知识\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 62,\n" +
" \"id\": 2742\n" +
" },\n" +
" {\n" +
" \"child\": [\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"文言文阅读\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 0,\n" +
" \"id\": 2782\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"古诗词阅读\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 1,\n" +
" \"id\": 2783\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"现代文阅读\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 2,\n" +
" \"id\": 2784\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"现代诗歌阅读\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 3,\n" +
" \"id\": 2785\n" +
" }\n" +
" ],\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"阅读理解及鉴赏\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 63,\n" +
" \"id\": 2781\n" +
" },\n" +
" {\n" +
" \"child\": [\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"综合读写\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 0,\n" +
" \"id\": 2787\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"口语交际\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 1,\n" +
" \"id\": 2788\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"扩展语段\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 2,\n" +
" \"id\": 2789\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"压缩语段\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 3,\n" +
" \"id\": 2790\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"理解句子\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 4,\n" +
" \"id\": 2791\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"课文理解,名著阅读\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 5,\n" +
" \"id\": 2792\n" +
" }\n" +
" ],\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"语言表达及应用\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 64,\n" +
" \"id\": 2786\n" +
" },\n" +
" {\n" +
" \"child\": [\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"材料作文\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 0,\n" +
" \"id\": 2794\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"话题作文\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 1,\n" +
" \"id\": 2795\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"命题作文、半命题作文\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 2,\n" +
" \"id\": 2796\n" +
" },\n" +
" {\n" +
" \"child\": null,\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"其他作文\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 3,\n" +
" \"id\": 2797\n" +
" }\n" +
" ],\n" +
" \"file\": null,\n" +
" \"resKnowledgeMenu\": null,\n" +
" \"pointName\": \"写作\",\n" +
" \"menuId\": 10,\n" +
" \"seq\": 65,\n" +
" \"id\": 2793\n" +
" }\n" +
" ]\n" +
"}";
zTreeResult result=new TreeUtil().parseTree(data);
System.out.println(JSON.toJSONString(result));
}
}
相关vo:
/**
* Copyright 2017 bejson.com
*/
package com.sdzn.vo.tree;
import java.util.List;
import org.codehaus.jackson.annotate.JsonIgnoreProperties;
import org.codehaus.jackson.annotate.JsonProperty;
/**
* Auto-generated: 2017-04-13 9:49:28
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
public class Child {
private List<Child> child;
private String file;
private String resKnowledgeMenu;
private String pointName;
private int menuId;
private int seq;
private int id;
public List<Child> getChild() {
return child;
}
public void setChild(List<Child> child) {
this.child = child;
}
public String getFile() {
return file;
}
public void setFile(String file) {
this.file = file;
}
public String getResKnowledgeMenu() {
return resKnowledgeMenu;
}
public void setResKnowledgeMenu(String resKnowledgeMenu) {
this.resKnowledgeMenu = resKnowledgeMenu;
}
public String getPointName() {
return pointName;
}
public void setPointName(String pointName) {
this.pointName = pointName;
}
public int getMenuId() {
return menuId;
}
public void setMenuId(int menuId) {
this.menuId = menuId;
}
public int getSeq() {
return seq;
}
public void setSeq(int seq) {
this.seq = seq;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
/**
* Copyright 2017 bejson.com
*/
package com.sdzn.vo.tree;
import java.util.List;
import org.codehaus.jackson.annotate.JsonIgnoreProperties;
import org.codehaus.jackson.annotate.JsonProperty;
/**
* Auto-generated: 2017-04-13 9:49:28
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
public class Data {
private List<Child> child;
private String file;
private String resKnowledgeMenu;
private String pointName;
private int menuId;
private int seq;
private int id;
public List<Child> getChild() {
return child;
}
public void setChild(List<Child> child) {
this.child = child;
}
public String getFile() {
return file;
}
public void setFile(String file) {
this.file = file;
}
public String getResKnowledgeMenu() {
return resKnowledgeMenu;
}
public void setResKnowledgeMenu(String resKnowledgeMenu) {
this.resKnowledgeMenu = resKnowledgeMenu;
}
public String getPointName() {
return pointName;
}
public void setPointName(String pointName) {
this.pointName = pointName;
}
public int getMenuId() {
return menuId;
}
public void setMenuId(int menuId) {
this.menuId = menuId;
}
public int getSeq() {
return seq;
}
public void setSeq(int seq) {
this.seq = seq;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
}
package com.sdzn.vo.tree;
import com.sdzn.thrift.dubbo.vo.Msg;
import java.io.Serializable;
import java.util.List;
/**
* zTreeResult 功能描述:zTree返回信息
*
* @author RickyLee【lsr@sdzn.com.cn】
* @date 2017/4/13 9:59
*/
public class zTreeResult implements Serializable{
private Msg msg;
private List<zTreeVO> data;
public Msg getMsg() {
return msg;
}
public void setMsg(Msg msg) {
this.msg = msg;
}
public List<zTreeVO> getData() {
return data;
}
public void setData(List<zTreeVO> data) {
this.data = data;
}
}
package com.sdzn.vo.tree;
import java.io.Serializable;
/**
* zTreeVO 功能描述:zTree树节点属性信息
*
* @author RickyLee【lsr@sdzn.com.cn】
* @date 2017/4/13 9:57
*/
public class zTreeVO implements Serializable{
private Integer id;
private Integer PId;
private String name;
private Integer seq;
private boolean checked=false;
private boolean open=true;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public Integer getPId() {
return PId;
}
public Integer getSeq() {
return seq;
}
public void setSeq(Integer seq) {
this.seq = seq;
}
public void setPId(Integer PId) {
this.PId = PId;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public boolean isChecked() {
return checked;
}
public void setChecked(boolean checked) {
this.checked = checked;
}
public boolean isOpen() {
return open;
}
public void setOpen(boolean open) {
this.open = open;
}
}
/**
* Copyright 2017 bejson.com
*/
package com.sdzn.vo.tree;
import java.util.List;
/**
* Auto-generated: 2017-04-13 9:49:28
*
* @author bejson.com (i@bejson.com)
* @website http://www.bejson.com/java2pojo/
*/
public class ZyptTree {
private int code;
private String msg;
private List<Data> data;
public void setCode(int code) {
this.code = code;
}
public int getCode() {
return code;
}
public void setMsg(String msg) {
this.msg = msg;
}
public String getMsg() {
return msg;
}
public void setData(List<Data> data) {
this.data = data;
}
public List<Data> getData() {
return data;
}
}
package com.sdzn.enums;
/**
* MsgCode 功能描述:返回状态码枚举
*
* @author RickyLee【lsr@sdzn.com.cn】
* @date 2016/11/21 13:02
*/
public class MsgCode {
//成功
public final static int SUCCESS =0;
//失败
public final static int FAIL =-1;
}
package com.sdzn.thrift.dubbo.vo;
import java.io.Serializable;
/**
* Msg 功能描述:接口调用返回状态说明
*
* @author RickyLee【lsr@sdzn.com.cn】
* @date 2016/11/21 12:57
*/
public class Msg implements Serializable{
private Integer status=null;
private String message=null;
public Integer getStatus() {
return status;
}
public void setStatus(Integer status) {
this.status = status;
}
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
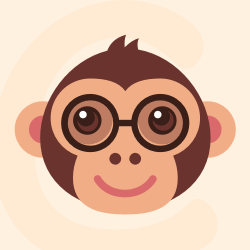



适用于现代 C++ 的 JSON。
最近提交(Master分支:1 个月前 )
960b763e
4 个月前
8c391e04
6 个月前
更多推荐
所有评论(0)