Java以post请求发送文件或json数据
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
分别给出了post发送文件和json数据的函数,其中使用到了Jackson库来转化Json数据,使用log4j2来打印日记,可自行剔除。
public class HttpUtils {
static private ObjectMapper objectMapper=new ObjectMapper();
static private Logger logger= LogManager.getLogger();
/**
* 以post请求方式发送文件
* @param url
* @param name 文件的请求参数名
* @param fileName 文件名
* @param fileInput 文件的输入流
* @return 默认返回参数为Json格式的数据
* @throws IOException
* @throws InterruptedException
*/
static public Map<String,Object> postFile2(String url,String name,String fileName,InputStream fileInput) throws IOException, InterruptedException {
String charset = "UTF-8";
String boundary = Long.toHexString(System.currentTimeMillis()); // Just generate some unique random value.
String CRLF = "\r\n"; // Line separator required by multipart/form-data.
URLConnection connection = new URL(url).openConnection();
connection.setDoOutput(true);
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=" + boundary);
try (
OutputStream output = connection.getOutputStream();
PrintWriter writer = new PrintWriter(new OutputStreamWriter(output, charset), true);
) {
// Send binary file.
writer.append("--" + boundary).append(CRLF);
writer.append("Content-Disposition: form-data; name=\""+URLEncoder.encode(name,"UTF-8")+"\"; filename=\"" + URLEncoder.encode(fileName,"UTF-8") + "\"").append(CRLF);
writer.append("Content-Type: " + URLConnection.guessContentTypeFromName(fileName)).append(CRLF);
writer.append("Content-Transfer-Encoding: binary").append(CRLF);
writer.append(CRLF).flush();
fileInput.transferTo(output);
output.flush(); // Important before continuing with writer!
writer.append(CRLF).flush(); // CRLF is important! It indicates end of boundary.
// End of multipart/form-data.
writer.append("--" + boundary + "--").append(CRLF).flush();
}
// Request is lazily fired whenever you need to obtain information about response.
int responseCode = ((HttpURLConnection) connection).getResponseCode();
System.out.println(responseCode); // Should be 200
//读取响应消息
BufferedReader reader=new BufferedReader(new InputStreamReader(connection.getInputStream()));
StringBuilder stringBuilder=new StringBuilder();
String tempStr=null;
while((tempStr=reader.readLine())!=null){
stringBuilder.append(tempStr);
}
//转化为JSON
Map<String,Object> response= objectMapper.readValue(stringBuilder.toString(),Map.class);
return response;
}
/**
* 以post请求发送Json文件
*/
public static Map<String,Object> sendJsonPost(String url,String jsonStr) throws IOException, Utils.ReceiveBadCodeException {
HttpURLConnection httpURLConnection= (HttpURLConnection) new URL(url).openConnection();
//配置连接
httpURLConnection.setDoOutput(true);
httpURLConnection.setRequestMethod("POST");
httpURLConnection.setRequestProperty("Content-Type","application/json;charset=UTF-8");
OutputStream out=httpURLConnection.getOutputStream();
//发送
out.write(jsonStr.getBytes("UTF-8"));
//检查响应
InputStream in=httpURLConnection.getInputStream();
StringBuilder stringBuilder=new StringBuilder();
byte[] bytes=new byte[1024];
int num;
while((num=in.read(bytes))!=-1){
stringBuilder.append(new String(bytes,"utf-8"));
}
Map<String,Object> response=objectMapper.readValue(stringBuilder.toString(),Map.class);
return response;
}
}
参考:
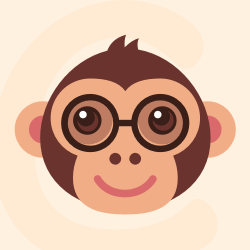



适用于现代 C++ 的 JSON。
最近提交(Master分支:1 个月前 )
960b763e
3 个月前
8c391e04
6 个月前
更多推荐
所有评论(0)