用ThreadPoolExecutor的时候,又想知道被执行的任务的执行情况,这时就可以用FutureTask。
原创不易,转载请注明出处:spring线程池ThreadPoolExecutor配置并且得到任务执行的结果
代码下载地址:http://www.zuidaima.com/share/1724478138158080.htm
ThreadPoolTask
01 | package com.zuidaima.threadpool; |
03 | import java.io.Serializable; |
04 | import java.util.concurrent.Callable; |
06 | public class ThreadPoolTask implements Callable<String>, Serializable { |
08 | private static final long serialVersionUID = 0 ; |
11 | private Object threadPoolTaskData; |
13 | private static int consumeTaskSleepTime = 2000 ; |
15 | public ThreadPoolTask(Object tasks) { |
16 | this .threadPoolTaskData = tasks; |
19 | public synchronized String call() throws Exception { |
21 | System.out.println( "开始执行任务:" + threadPoolTaskData); |
26 | for ( int i = 0 ; i < 100000000 ; i++) { |
30 | } catch (Exception e) { |
34 | threadPoolTaskData = null ; |
模拟客户端提交的线程
01 | package com.zuidaima.threadpool; |
03 | import java.util.concurrent.ExecutionException; |
04 | import java.util.concurrent.FutureTask; |
05 | import java.util.concurrent.TimeUnit; |
07 | import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor; |
09 | public class StartTaskThread implements Runnable { |
11 | private ThreadPoolTaskExecutor threadPoolTaskExecutor; |
14 | public StartTaskThread(ThreadPoolTaskExecutor threadPoolTaskExecutor, int i) { |
15 | this .threadPoolTaskExecutor = threadPoolTaskExecutor; |
20 | public synchronized void run() { |
21 | String task = "task@ " + i; |
22 | System.out.println( "创建任务并提交到线程池中:" + task); |
23 | FutureTask<String> futureTask = new FutureTask<String>( |
24 | new ThreadPoolTask(task)); |
25 | threadPoolTaskExecutor.execute(futureTask); |
30 | result = futureTask.get( 1000 , TimeUnit.MILLISECONDS); |
31 | } catch (InterruptedException e) { |
32 | futureTask.cancel( true ); |
33 | } catch (ExecutionException e) { |
34 | futureTask.cancel( true ); |
35 | } catch (Exception e) { |
36 | futureTask.cancel( true ); |
39 | System.out.println( "task@" + i + ":result=" + result); |
SPRING配置文件
01 | <?xml version= "1.0" encoding= "UTF-8" ?> |
11 | <bean id= "threadPoolTaskExecutor" |
12 | class = "org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor" > |
15 | <property name= "corePoolSize" value= "10" /> |
17 | <!-- 最大线程数,默认为Integer.MAX_VALUE --> |
18 | <property name= "maxPoolSize" value= "50" /> |
20 | <!-- 队列最大长度,一般需要设置值>=notifyScheduledMainExecutor.maxNum;默认为Integer.MAX_VALUE |
21 | <property name= "queueCapacity" value= "1000" /> --> |
23 | <!-- 线程池维护线程所允许的空闲时间,默认为60s --> |
24 | <property name= "keepAliveSeconds" value= "300" /> |
26 | <!-- 线程池对拒绝任务(无线程可用)的处理策略,目前只支持AbortPolicy、CallerRunsPolicy;默认为后者 --> |
27 | <property name= "rejectedExecutionHandler" > |
28 | <!-- AbortPolicy:直接抛出java.util.concurrent.RejectedExecutionException异常 --> |
29 | <!-- CallerRunsPolicy:主线程直接执行该任务,执行完之后尝试添加下一个任务到线程池中,可以有效降低向线程池内添加任务的速度 --> |
30 | <!-- DiscardOldestPolicy:抛弃旧的任务、暂不支持;会导致被丢弃的任务无法再次被执行 --> |
31 | <!-- DiscardPolicy:抛弃当前任务、暂不支持;会导致被丢弃的任务无法再次被执行 --> |
32 | <bean class = "java.util.concurrent.ThreadPoolExecutor$CallerRunsPolicy" /> |
测试类
01 | package com.zuidaima.test; |
03 | import org.junit.Test; |
04 | import org.junit.runner.RunWith; |
05 | import org.springframework.beans.factory.annotation.Autowired; |
06 | import org.springframework.scheduling.concurrent.ThreadPoolTaskExecutor; |
07 | import org.springframework.test.context.ContextConfiguration; |
08 | import org.springframework.test.context.junit4.AbstractJUnit4SpringContextTests; |
09 | import org.springframework.test.context.junit4.SpringJUnit4ClassRunner; |
11 | import com.zuidaima.threadpool.StartTaskThread; |
13 | @RunWith (SpringJUnit4ClassRunner. class ) |
15 | @ContextConfiguration (locations = "classpath*:applicationContext.xml" ) |
16 | public class TestThreadPool extends AbstractJUnit4SpringContextTests { |
18 | private static int produceTaskSleepTime = 10 ; |
20 | private static int produceTaskMaxNumber = 1000 ; |
23 | private ThreadPoolTaskExecutor threadPoolTaskExecutor; |
25 | public ThreadPoolTaskExecutor getThreadPoolTaskExecutor() { |
26 | return threadPoolTaskExecutor; |
29 | public void setThreadPoolTaskExecutor( |
30 | ThreadPoolTaskExecutor threadPoolTaskExecutor) { |
31 | this .threadPoolTaskExecutor = threadPoolTaskExecutor; |
35 | public void testThreadPoolExecutor() { |
36 | for ( int i = 1 ; i <= produceTaskMaxNumber; i++) { |
38 | Thread.sleep(produceTaskSleepTime); |
39 | } catch (InterruptedException e1) { |
42 | new Thread( new StartTaskThread(threadPoolTaskExecutor, i)).start(); |
原文中有些纰漏,我已经修改
项目截图(基于maven构建)
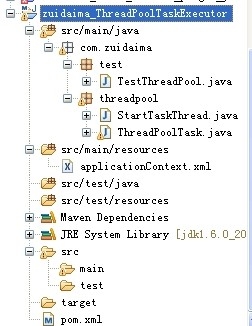
运行截图:
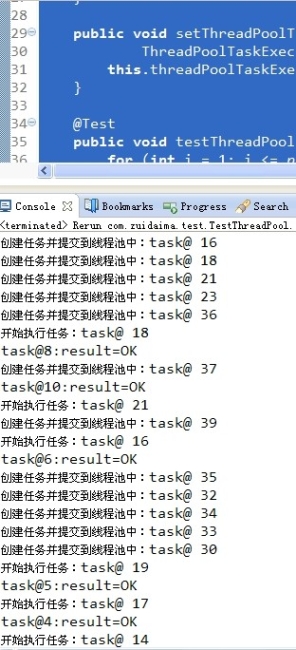
如果遇到cpu忙执行超过1秒的会返回null
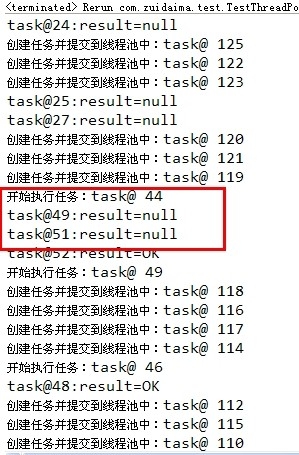
所有评论(0)