vue2.9 使用croppa实现图像裁剪并上传服务器 (croppa裁剪工具 + element 框架搭建)

首先安装croppa
npm install --save vue-croppa
安装完成之后,在需要的页面引入
import Vue from "vue";
//引用Croppa图片裁剪
import Croppa from "vue-croppa";
import "vue-croppa/dist/vue-croppa.css";
Vue.use(Croppa);
其中基本参数
- v-model="croppa" 绑定别名croppa来操作js
- :show-remove-button="false" 是否显示取消按钮(默认true)
- :width="360" :height="360" 设置默认宽高
-
:prevent-white-space="true" 防止出现空白(默认false)
-
accept="image/png" 接受文件类型
-
:initial-image=“http://....” 默认加载的图像
-
initial-size="natural" 初始大小不缩放 (cover,contain,natural)
-
initial-position="center" 初始位置剧中(left,right,top,bottom,center,100% 20%)
-
:zoom-speed="10" 缩放/放大 速度
-
:disabled="false" 禁止选中
-
:disable-click-to-choose="true" 禁止再次点击
-
:show-loading="true" 是否展示加载动画
-
:loading-size="50" 加载动画大小
-
:loading-color="#606060" 加载动画颜色
-
placeholder="选择图片" 默认提示文字
-
placeholder-color="#000" 默认提示文字颜色
-
:placeholder-font-size="16" 默认提示文字大小
在template模板中添加el弹框组件和croppa裁剪组件
<!-- 引用el的dialog弹框组件,默认data中设置croppaVisible=true -->
<el-dialog title="图片裁剪" :visible.sync="croppaVisible" width="640px" :before-close="handleClose">
<div class="croppa_box">
<croppa v-model="croppa" :show-remove-button="false" :width="360" :height="360" :prevent-white-space="true" initial-size="natural"
initial-position="center" :initial-image="'http://t00img.yangkeduo.com/goods/images/2018-07-19/15a2e96caa3851da064e464cf60eea3a.jpeg'"></croppa>
<div class="croppa_btns_box">
<div class="croppa_btns_box_top">
<el-tooltip class="item" effect="dark" content="上移" placement="top">
<el-button type="primary" circle @click="croppa.moveUpwards(10)" icon="el-icon-arrow-up"></el-button>
</el-tooltip>
<el-tooltip class="item" effect="dark" content="下移" placement="top">
<el-button type="primary" circle @click="croppa.moveDownwards(10)" icon="el-icon-arrow-down"></el-button>
</el-tooltip>
<el-tooltip class="item" effect="dark" content="左移" placement="top">
<el-button type="primary" circle @click="croppa.moveLeftwards(10)" icon="el-icon-arrow-left"></el-button>
</el-tooltip>
<el-tooltip class="item" effect="dark" content="右移" placement="top">
<el-button type="primary" circle @click="croppa.moveRightwards(10)" icon="el-icon-arrow-right"></el-button>
</el-tooltip>
<el-tooltip class="item" effect="dark" content="放大" placement="top">
<el-button type="primary" circle @click="croppa.zoomIn(10)" icon="el-icon-zoom-in"></el-button>
</el-tooltip>
<el-tooltip class="item" effect="dark" content="缩小" placement="top">
<el-button type="primary" circle @click="croppa.zoomOut(10)" icon="el-icon-zoom-out"></el-button>
</el-tooltip>
</div>
<div class="croppa_btns_box_bottom">
<el-button type="primary" round @click="croppa.rotate()">旋转90°</el-button>
<el-button type="primary" round @click="croppa.rotate(2)">旋转180°</el-button>
<el-button type="primary" round @click="croppa.rotate(-1)">旋转-90°</el-button>
<el-button type="primary" round @click="croppa.flipX()">水平翻转</el-button>
<el-button type="primary" round @click="croppa.flipY()">垂直翻转</el-button>
</div>
</div>
</div>
<span slot="footer" class="dialog-footer">
<el-button @click="croppaVisible = false">取 消</el-button>
<el-button type="primary" @click="uploadCroppedImage">确 定</el-button>
</span>
</el-dialog>
点击确认按钮,进行文件的上传
文件上传接口和post两处不同
一:是header设置为'Content-Type': 'multipart/form-data'
二:千万不要对data数据进行Qs.stringify()...太坑了
在data中添加变量
croppa: {}
在methods中添加方法
//图片裁剪
uploadCroppedImage() {
let that = this;
this.croppa.generateBlob(
blob => {
// 编写代码上传裁剪的图像文件
// this.croppa.generateDataUrl() base64
// console.log(blob);
let formdata = new FormData();
formdata.append("file", blob, "image.png"); //封装到formdata中
//uploadFile接口和post两处不同
//一:是header设置为'Content-Type': 'multipart/form-data'
//二:千万不要对data数据进行Qs.stringify()...太坑了
that.$http.uploadFile(上传地址, formdata).then(res => {
console.log(res);
let data = res.data;
if (data.status) {
that.$message.success("保存成功");
that.dialogVisible = false;
}
});
},
"image/jpeg",
0.8
); // 80%压缩文件
}
就可以直接从服务器中获取到文件地址并使用了
官网实例
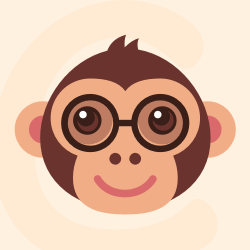



更多推荐
所有评论(0)