使用Newtonsoft.Json 解决Json日期格式问题
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
介绍
Asp.Net MVC默认是使用JavaScriptSerializer做Json序列化的,不好用。而且JavaScriptSerializer无法处理循环引用,对日期的格式化不友好。例如对当前日期序列化后的效果是这样的:【CreateTime: "/Date(1521983727837)/"】 这样的日期我们很难看懂
而且JavaScriptSerializer对一个对象的序列化,序列化后的json对象属性与C#中的对象的属性名称一致。因为我们在javascript中习惯将对象属性的第一个字母是以小写开头的,不习惯属性的第一个字母是大写开头的,比如:,比如 id,name,createTime而在C#中,我们对象的属性名称习惯是以大些字母开头,比如Id,Name,CreateTime
如果使用JavaScriptSerializer对C#对象进行序列化,序列化后的属性名称与c#定义的属性名称一致,无法将对象第一个字母变为小写的字母,这样对前端的开发人员就不太友好(前端开发人员会觉得这样的属性名称很恶心) 那有什么办法解决这个问题呢? 这里我们就得说说这个Newtonsoft.Json了
举列:
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime CreateTime { get; set; }
}
如果使用JavaScriptSerializer对这个对象序列化,序列化后的效果是这样的:
{Id: 1, Name: "张三", CreateTime: "/Date(1521983727837)/"}
那什么现在使用newtonjs 对这个对象进行序列化就能达到我们想要的效果:
{id: 1, name: "张三", createTime: "2018-03-25 22:26:07"}
那现在什么来看看那这个Newtonsoft.Json怎么用
第一步:首先去Nuget中 安装Newtonsoft.Json 版本是11.0.2
或者执行PM>Install-Package Newtonsoft.Json -Version 11.0.2
第二步: 新建一个JsonNetResult类 让这个类继承JsonResult类
namespace MvcApp.Controllers
{
public class JsonNetResult : JsonResult
{
public JsonNetResult()
{
Settings = new JsonSerializerSettings
{
ReferenceLoopHandling = ReferenceLoopHandling.Ignore,//忽略循环引用,如果设置为Error,则遇到循环引用的时候报错(建议设置为Error,这样更规范)
DateFormatString = "yyyy-MM-dd HH:mm:ss",//日期格式化,默认的格式也不好看
ContractResolver = new Newtonsoft.Json.Serialization.CamelCasePropertyNamesContractResolver()//json中属性开头字母小写的驼峰命名
};
}
public JsonSerializerSettings Settings { get; private set; }
public override void ExecuteResult(ControllerContext context)//重写JsonResult类的ExecuteResult方法
{
if (context == null)
throw new ArgumentNullException("context");
//判断是否运行Get请求
if (this.JsonRequestBehavior == JsonRequestBehavior.DenyGet
&& string.Equals(context.HttpContext.Request.HttpMethod, "GET", StringComparison.OrdinalIgnoreCase))
throw new InvalidOperationException("JSON GET is not allowed");
//设定响应数据格式。默认为json
HttpResponseBase response = context.HttpContext.Response;
response.ContentType = string.IsNullOrEmpty(this.ContentType) ? "application/json" : this.ContentType;
//设定内容编码格式
if (this.ContentEncoding != null)
response.ContentEncoding = this.ContentEncoding;
if (this.Data == null)
return;
var scriptSerializer = JsonSerializer.Create(this.Settings);
scriptSerializer.Serialize(response.Output, this.Data);
}
}
第三步:现在我们看怎么在控制器中使用:
namespace MvcApp.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
[HttpPost]
public JsonNetResult TestJson()
{
Person person = new Person() { Id = 1, Name = "张三", CreateTime = DateTime.Now };
//直接这样使用就可以啦
return new JsonNetResult() { Data = person };
}
}
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime CreateTime { get; set; }
}
}
目的结果:
{id: 1, name: "张三", createTime: "2018-03-25 22:26:07"}
第一种扩展 :为了使用方便使用,可以在过滤器中对JsonResult替换成我们自己的JsonNetResult(推荐)
由于直接return new JsonNetResult(){Data=person} 对MVC框架侵入式比较强,用起来还是不是很方便,那么如何尽量保持原来的使用方式不变的情况下来使用我们自定义的JsonNetResult呢?方法很简单,就是使用ActionFilterAttribute过滤器
我们新建一个JsonNetResultAttribute的顾虑器,让它继承ActionFilterAttribute 或者是直接实现IActionFilter接口,我这里是直接选择实现然后实现IActionFilter接口中的OnActionExecuted方法,在这个方法中将原先的JsonReuslt替换成我们的自定义的JsonNetResult,就达到目的了
第一步:自定义一个JsonNetResult过滤器
namespace MvcApp.Filters
{
using MvcApp.Controllers;
using System.Web.Mvc;
public class JsonNetResultAttritube : IActionFilter
{
/// <summary>
/// 注意:OnActionExecuted是在Action方法执行之后被执行
/// 在这里我们将JsonResult替换成我们的JsonNetResult
/// </summary>
/// <param name="filterContext"></param>
public void OnActionExecuted(ActionExecutedContext filterContext)
{
ActionResult result = filterContext.Result;
if (result is JsonResult && !(result is JsonNetResult))
{
JsonResult jsonResult = (JsonResult)result;
JsonNetResult jsonNetResult = new JsonNetResult();
jsonNetResult.ContentEncoding = jsonResult.ContentEncoding;
jsonNetResult.ContentType = jsonResult.ContentType;
jsonNetResult.JsonRequestBehavior = jsonResult.JsonRequestBehavior;
jsonNetResult.Data = jsonResult.Data;
jsonNetResult.MaxJsonLength = jsonResult.MaxJsonLength;
jsonNetResult.RecursionLimit = jsonResult.RecursionLimit;
filterContext.Result = jsonNetResult;
}
}
public void OnActionExecuting(ActionExecutingContext filterContext)
{
}
}
}
第二步:过滤器中注册这个过滤器
namespace MvcApp.App_Start
{
public class FilterConfig
{
public static void RegisterGlobalFilters(GlobalFilterCollection filters)
{
filters.Add(new HandleErrorAttribute());
filters.Add(new JsonNetResultAttritube());
}
}
}
第三步:在控制中使用
原来怎么用就怎么用,完全不用任何更改,就达到了我们的目的
namespace MvcApp.Controllers
{
using System.Web.Mvc;
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
[HttpPost]
public JsonResult TestJson()
{
Person person = new Person() { Id = 1, Name = "张三", CreateTime = DateTime.Now };
//原来该怎么用还是怎么用(只是通过过滤器,我们将这个Json(person)替换成我们自己的JsonNetResult了)
return Json(person);
}
}
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime CreateTime { get; set; }
}
}
目的结果:
{id: 1, name: "张三", createTime: "2018-03-25 22:26:07"}
第二种扩展:是对控制器做一些扩展,也可以让使用更加方便
第一步:扩展控制器
public static class ControllerExpand
{
public static JsonNetResult JsonNet(this Controller JsonNet, object data)
{
return new JsonNetResult() { Data = data };
}
public static JsonNetResult JsonNet(this Controller JonsNet, object data, JsonRequestBehavior behavior)
{
return new JsonNetResult()
{
Data = data,
JsonRequestBehavior = behavior
};
}
public static JsonNetResult JsonNet(this Controller JonsNet,object data, string contentType, Encoding contentEncoding)
{
return new JsonNetResult()
{
Data = data,
ContentType = contentType,
ContentEncoding = contentEncoding
};
}
public static JsonNetResult JsonNet(this System.Web.Mvc.Controller JonsNet, object data, string contentType, Encoding contentEncoding, JsonRequestBehavior behavior)
{
return new JsonNetResult()
{
Data = data,
ContentType = contentType,
ContentEncoding = contentEncoding,
JsonRequestBehavior = behavior
};
}
}
第二步:在控制器中使用
namespace MvcApp.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
[HttpPost]
public JsonNetResult TestJson()
{
Person person = new Person() { Id = 1, Name = "张三", CreateTime = DateTime.Now };
//直接这样使用就可以啦
//return new JsonNetResult() { Data = person };
//这样使用是不是更加方便了呢?哈哈
return this.JsonNet(person);
}
}
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public DateTime CreateTime { get; set; }
}
}
目的结果:
{id: 1, name: "张三", createTime: "2018-03-25 22:26:07"}
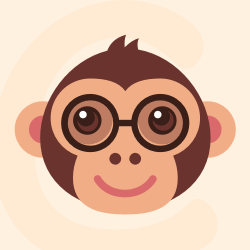



适用于现代 C++ 的 JSON。
最近提交(Master分支:1 个月前 )
960b763e
4 个月前
8c391e04
6 个月前
更多推荐
所有评论(0)