TensorFlow实现卷积、池化操作

1.调用tf.nn.conv2d()实现卷积
首先是调用卷积函数实现卷积操作:
这里说明一下conv2d的定义及参数含义: 参考
【定义:】
tf.nn.conv2d (input, filter, strides, padding, use_cudnn_on_gpu=None, data_format=None, name=None)
【参数:】
input : 输入的要做卷积的图片,要求为一个张量,shape为 [ batch, in_height, in_weight, in_channel ],其中batch为图片的数量,in_height 为图片高度,in_weight 为图片宽度,in_channel 为图片的通道数,灰度图该值为1,彩色图为3。(也可以用其它值,但是具体含义不是很理解)
filter: 卷积核,要求也是一个张量,shape为 [ filter_height, filter_weight, in_channel, out_channels ],其中 filter_height 为卷积核高度,filter_weight 为卷积核宽度,in_channel 是图像通道数 ,和 input 的 in_channel 要保持一致,out_channel 是卷积核数量。
strides: 卷积时在图像每一维的步长,这是一个一维的向量,[ 1, strides, strides, 1],第一位和最后一位固定必须是1
padding: string类型,值为“SAME” 和 “VALID”,表示的是卷积的形式,是否考虑边界。"SAME"是考虑边界,不足的时候用0去填充周围,"VALID"则不考虑
use_cudnn_on_gpu: bool类型,是否使用cudnn加速,默认为true
2.TensorFlow tf.nn.max_pool实现池化操作方式
max pooling是CNN当中的最大值池化操作,其实用法和卷积很类似
tf.nn.max_pool(value, ksize, strides, padding, name=None)
参数是四个,和卷积很类似:
第一个参数value:需要池化的输入,一般池化层接在卷积层后面,所以输入通常是feature map,依然是[batch, height, width, channels]这样的shape
第二个参数ksize:池化窗口的大小,取一个四维向量,一般是[1, height, width, 1],因为我们不想在batch和channels上做池化,所以这两个维度设为了1
第三个参数strides:和卷积类似,窗口在每一个维度上滑动的步长,一般也是[1, stride,stride, 1]
第四个参数padding:和卷积类似,可以取'VALID' 或者'SAME'
返回一个Tensor,类型不变,shape仍然是[batch, height, width, channels]这种形式
import tensorflow as tf
import numpy as np
input = np.array([[12,21,1,1],
[11,10,10,2],
[1,10,10,23],
[45,31,11,32]],dtype='float32')
input = input.reshape([1,4,4,1]) #因为conv2d的参数都是四维的,因此要reshape成四维
kernel = np.array([[2,-1],[-1,0]])
kernel = kernel.reshape([2,2,1,1]) #kernel也要reshape
print(input.shape,kernel.shape) #(1, 4, 4, 1) (2, 2, 1, 1)
x = tf.placeholder(tf.float32,[1,4,4,1])
k = tf.placeholder(tf.float32,[2,2,1,1])
conv_result = tf.nn.conv2d(x,k,strides=[1,1,1,1],padding='SAME')
max_pooling=tf.nn.max_pool(input,[1,2,2,1],[1,1,1,1],padding='VALID')
ave_polling = tf.nn.avg_pool(input,[1,2,2,1],[1,1,1,1],padding='VALID')
with tf.Session() as sess:
y = sess.run(conv_result,feed_dict={x:input,k:kernel})
max_polling=sess.run(max_pooling)
ave_polling=sess.run(ave_polling)
y=y.reshape([4,4])
max_polling = max_polling.reshape([3,3])
ave_polling = ave_polling.reshape([3,3])
print(y.shape) #(1,3,3,1)
print(max_polling.shape)
print(ave_polling.shape)
print(y) #因为y有四维,输出太长了,我就只写一下中间两维的结果(3*3部分):[[4,3,4],[2,4,3],[2,3,4]]
print(max_polling)
print(ave_polling)
reference:https://blog.csdn.net/aaon22357/article/details/85283502
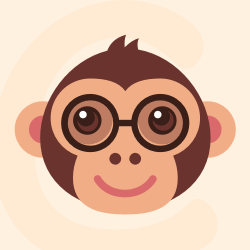



更多推荐
所有评论(0)