CXF+Spring+JAXB+Json构建Restful服务
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
话不多说,先看具体的例子:
文件目录结构:
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
version="2.5">
<display-name>platform</display-name>
<!-- Spring ApplicationContext配置文件的路径,可使用通配符,多个路径用,号分隔 此参数用于后面的Spring Context
Loader -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:/applicationContext.xml,classpath:/applicationContext-rs.xml
</param-value>
</context-param>
<!-- Character Encoding filter -->
<filter>
<filter-name>encodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!-- Spring 刷新Introspector防止内存泄露 -->
<listener>
<listener-class>org.springframework.web.util.IntrospectorCleanupListener</listener-class>
</listener>
<!-- Apache CXF Servlet 配置 -->
<servlet>
<servlet-name>CXFServlet</servlet-name>
<servlet-class>org.apache.cxf.transport.servlet.CXFServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>CXFServlet</servlet-name>
<url-pattern>/rest/*</url-pattern>
</servlet-mapping>
</web-app>
Spring的xml—applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jee="http://www.springframework.org/schema/jee"
xmlns:tx="http://www.springframework.org/schema/tx" xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-3.2.xsd
http://www.springframework.org/schema/jee
http://www.springframework.org/schema/jee/spring-jee-3.2.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.2.xsd"
default-lazy-init="true">
<description>Spring公共配置文件</description>
<!-- 使用annotation 自动注册bean,并保证@Required,@Autowired的属性被注入 -->
<context:component-scan base-package="com.zd.daoImpl.*,com.zd.beanImpl.*,com.zd.serviceImpl.*,com.zd.service.*">
</context:component-scan>
<bean id="dozerMapper" class="org.dozer.DozerBeanMapper"
lazy-init="false" />
<!-- Matrix BPM -->
<bean id="transactionInterceptor"
class="org.springframework.transaction.interceptor.TransactionInterceptor">
<property name="transactionManager">
<ref bean="transactionManager" />
</property>
<property name="transactionAttributes">
<props>
<prop key="*">PROPAGATION_REQUIRED,-Exception</prop>
</props>
</property>
</bean>
</beans>
RestFul的xml—
applicationContext-rs.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:jaxrs="http://cxf.apache.org/jaxrs"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://cxf.apache.org/jaxrs http://cxf.apache.org/schemas/jaxrs.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd">
<description>Rails CM 的所有RESTFUL 服务配置</description>
<context:component-scan base-package="com.zd" />
<bean id="jsonProvider" class="org.codehaus.jackson.jaxrs.JacksonJsonProvider"></bean>
<jaxrs:server id="restContainer" address="/">
<jaxrs:inInterceptors>
</jaxrs:inInterceptors>
<jaxrs:serviceBeans>
<ref bean="userinfoServiceImpl" />
</jaxrs:serviceBeans>
</jaxrs:server>
</beans>
实体:
package com.zd.entity;
import java.io.Serializable;
/** 系统用户表 **/
public class UserInfo implements Serializable {
private String id;
private String name;// 用户姓名
private String sex;// 性别
private String idNo;//身份证号码
private String account;// 账号
private String pwd;// 密码
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getIdNo() {
return idNo;
}
public void setIdNo(String idNo) {
this.idNo = idNo;
}
}
构建的XML实体:
package com.zd.dtd;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
@XmlRootElement(name="UserInfoDTO")
@XmlAccessorType(XmlAccessType.PROPERTY)
@XmlType
public class UserInfoDTD {
private String id;
private String name;// 用户姓名
private String sex;// 性别
private String idNo;//身份证号码
private String account;// 账号
private String pwd;// 密码
@XmlElement(name="id",required=false)
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
@XmlElement(name="name",required=false)
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@XmlElement(name="account",required=false)
public String getAccount() {
return account;
}
public void setAccount(String account) {
this.account = account;
}
@XmlElement(name="sex",required=false)
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
@XmlElement(name="sex",required=false)
public String getIdNo() {
return idNo;
}
public void setIdNo(String idNo) {
this.idNo = idNo;
}
@XmlElement(name="sex",required=false)
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
}
接口服务层:
package com.zd.service;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
@Path("/Userinfo")
public interface UserinfoService {
/**
* 根据id获取用户信息
* @param id 用户id
* @return json数据
*/
@GET
@Path("/getUserinfo")
@Produces({ "text/html;charset=utf-8", MediaType.TEXT_HTML })
public Response getBasicUserInfo(@QueryParam("id") String id);
}
服务实现层:
package com.zd.serviceImpl;
import javax.ws.rs.core.Response;
import net.sf.json.JSONArray;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.zd.beanImpl.UserBeanImpl;
import com.zd.dtd.UserInfoDTD;
import com.zd.entity.UserInfo;
import com.zd.service.UserinfoService;
@Component
public class UserinfoServiceImpl implements UserinfoService {
@Autowired(required=true)
private UserBeanImpl userBeanImpl;
@Override
public Response getBasicUserInfo(String id) {
UserInfo userInfo = userBeanImpl.getUserInfo(id);
UserInfoDTD userDTD=new UserInfoDTD();
userDTD.setSex(userInfo.getSex());
userDTD.setId(userInfo.getId());
userDTD.setIdNo(userInfo.getIdNo());
userDTD.setName(userInfo.getName());
userDTD.setPwd(userInfo.getPwd());
userDTD.setAccount(userInfo.getAccount());
//json转换
JSONArray jsonArray = JSONArray.fromObject(userInfo);
return Response.ok(jsonArray.toString()).build();
}
}
ServiceBean:
package com.zd.beanImpl;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import com.zd.daoImpl.UserinfoDaoImpl;
import com.zd.entity.UserInfo;
@Service
//默认将类中的所有函数纳入事务管理.
@Transactional
public class UserBeanImpl {
@Autowired(required=true)
private UserinfoDaoImpl userinfoDaoImpl;
public UserInfo getUserInfo(String id){
UserInfo userInfo=userinfoDaoImpl.getUserinfo(id);
return userInfo;
}
}
Dao:
package com.zd.daoImpl;
import org.springframework.stereotype.Repository;
import org.springframework.transaction.annotation.Transactional;
import com.zd.entity.UserInfo;
@Repository
//默认将类中的所有函数纳入事务管理.
@Transactional
public class UserinfoDaoImpl {
public UserInfo getUserinfo(String id){
UserInfo userInfo=new UserInfo();
userInfo.setAccount("zhudan");
userInfo.setId("1111");
userInfo.setId("520911188119186770");
userInfo.setName("朱丹");
userInfo.setPwd("1221");
userInfo.setSex("女");
return userInfo;
}
}
页面访问地址:
http://localhost:8080/restful/rest/Userinfo/getUserinfo
页面访问结果:
总结:
先由这个例子,宏观了解下Restful的服务搭建,Restful的细节东西再在以后的运用中深入理解
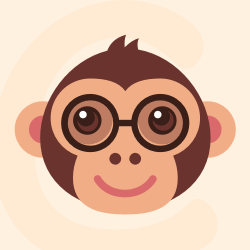



适用于现代 C++ 的 JSON。
最近提交(Master分支:1 个月前 )
960b763e
3 个月前
8c391e04
6 个月前
更多推荐
所有评论(0)