springboot使用HttpClient接受json参数转为对象,再将对象转为json
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
将json数据转为对象模型
http://wthrcdn.etouch.cn/weather_mini?city=深圳
上面链接可以请求天气数据,并返回json:
{
"data": {
"yesterday": {
"date": "4日星期三",
"high": "高温 32℃",
"fx": "无持续风向",
"low": "低温 27℃",
"fl": "<![CDATA[<3级]]>",
"type": "多云"
},
"city": "深圳",
"aqi": "26",
"forecast": [
{
"date": "5日星期四",
"high": "高温 32℃",
"fengli": "<![CDATA[<3级]]>",
"low": "低温 27℃",
"fengxiang": "无持续风向",
"type": "阵雨"
},
{
"date": "6日星期五",
"high": "高温 32℃",
"fengli": "<![CDATA[<3级]]>",
"low": "低温 28℃",
"fengxiang": "无持续风向",
"type": "阵雨"
},
{
"date": "7日星期六",
"high": "高温 33℃",
"fengli": "<![CDATA[<3级]]>",
"low": "低温 28℃",
"fengxiang": "无持续风向",
"type": "阵雨"
},
{
"date": "8日星期天",
"high": "高温 33℃",
"fengli": "<![CDATA[<3级]]>",
"low": "低温 28℃",
"fengxiang": "无持续风向",
"type": "阵雨"
},
{
"date": "9日星期一",
"high": "高温 32℃",
"fengli": "<![CDATA[<3级]]>",
"low": "低温 28℃",
"fengxiang": "无持续风向",
"type": "阵雨"
}
],
"ganmao": "各项气象条件适宜,发生感冒机率较低。但请避免长期处于空调房间中,以防感冒。",
"wendu": "29"
},
"status": 1000,
"desc": "OK"
}
首先创建对象接收json参数。分别在vo包中创建,四个对象。
其中weatherResponse为最顶级对象。
注意:对象属性名需要和json返回值相同,不然httpclient会解析失败。
引入httpclient依赖,(lombok可以在对象类上写@data,免除get、set的书写)
在实现类中写转换逻辑:
@Autowired
private RestTemplate restTemplate; //注入httpclient中的主要实现模板类
private static final String WEATHER_URI="http://wthrcdn.etouch.cn/weather_mini?"
@Override
public WeatherResponse getDataByCityName(String cityName) {
String uri=WEATHER_URI+"city="+cityName;
ResponseEntity<String> respString=restTemplate.getForEntity(uri,String.class); //httpclient中不能直接
将json转换为所需类,先转换成ResponseEntity
ObjectMapper mapper=new ObjectMapper(); //然后使用spring自带的进行转换
WeatherResponse resp=null;
String strBody=null;
if(respString.getStatusCodeValue()==200){ //判断返回的状态码
strBody=respString.getBody();
}
try {
resp=mapper.readValue(strBody,WeatherResponse.class);
}catch (IOException e){
e.printStackTrace();
}
return resp;
}
public WeatherResponse getDataByCityName(String cityName) {
String uri=WEATHER_URI+"city="+cityName;
ResponseEntity<String> respString=restTemplate.getForEntity(uri,String.class); //httpclient中不能直接
将json转换为所需类,先转换成ResponseEntity
ObjectMapper mapper=new ObjectMapper(); //然后使用spring自带的进行转换
WeatherResponse resp=null;
String strBody=null;
if(respString.getStatusCodeValue()==200){ //判断返回的状态码
strBody=respString.getBody();
}
try {
resp=mapper.readValue(strBody,WeatherResponse.class);
}catch (IOException e){
e.printStackTrace();
}
return resp;
}
然后还需要配置RestTemplate模板类:
最后:在controller中调用服务即可
。
至于将对象转换为json,在controller中返回对象,然后配置@RestController或者@ResponseBody即可自动转换。,也可以参考我另外一篇关于对象转换json的博客。
原文参考更多内容:http://blog.maptoface.com/post/29
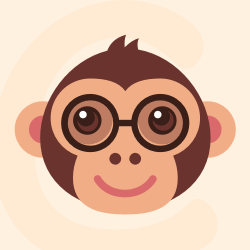



适用于现代 C++ 的 JSON。
最近提交(Master分支:3 个月前 )
2d42229f
* Support BSON uint64 de/serialization
Signed-off-by: Michael Valladolid <mikevalladolid@gmail.com>
* Treat 0x11 as uint64 and not timestamp specific
Signed-off-by: Michael Valladolid <mikevalladolid@gmail.com>
---------
Signed-off-by: Michael Valladolid <mikevalladolid@gmail.com> 4 天前
1809b3d8
Signed-off-by: Niels Lohmann <mail@nlohmann.me> 5 天前
更多推荐
所有评论(0)