C++ STL 算法——最大值max_element,最小值min_element,求和accumulate

最大值max_element,最小值min_element,求和accumulate
min_element 和 max_element头文件是algorithm
,返回值是一个迭代器
accumulate 头文件是numeric
,第三个参数是初始值,返回值是一个数
举个栗子
#include <algorithm>
#include <iostream>
#include <vector>
#include <numeric>
using namespace std;
int main() {
int a[] = {1, 2, 3, 4, 5};
vector<int> v({1, 2, 3, 4, 5});
// 普通数组
int minValue = *min_element(a, a + 5);
int maxValue = *max_element(a, a + 5);
int sumValue = accumulate(a, a + 5, 0);
// Vector数组
int minValue2 = *min_element(v.begin(), v.end());
int maxValue2 = *max_element(v.begin(), v.end());
int sumValue2 = accumulate(v.begin(), v.end(), 0);
cout << minValue << endl;
cout << maxValue << endl;
cout << sumValue << endl << endl;
cout << minValue2 << endl;
cout << maxValue2 << endl;
cout << sumValue2 << endl << endl;
return 0;
}
输出
1
5
15
1
5
15
max_element()及min_element()函数 时间复杂度 O ( n ) O(n) O(n)
C++数组或vector求最大值最小值
可以用max_element()
及min_element()
函数,二者返回的都是迭代器或指针。
头文件:#include<algorithm>
函数源代码
template <class ForwardIterator>
ForwardIterator max_element ( ForwardIterator first, ForwardIterator last )
{
if (first==last) return last;
ForwardIterator largest = first;
while (++first!=last)
if (*largest<*first) // or: if (comp(*largest,*first)) for version (2)
largest=first;
return largest;
}
上面的源码,解释了为什么可以有max_element(a, a + 5);的用法,
相当于first = a,last = a + 5;
*first = *a = *(a + 0) = a[0];
*last = *(a + 5) = a[5];
示例:
int a[5] = {0, 3, 9, 4, 5};
int *b;
b = max_element(a, a+5);
cout << *b;
1.求数组的最大值或最小值
1)vector容器
例 vector vec
最大值:int maxValue = *max_element(v.begin(),v.end());
最小值:int minValue = *min_element(v.begin(),v.end());
2)普通数组
例 a[]={1,2,3,4,5,6};
最大值:int maxValue = *max_element(a,a+6);
最小值:int minValue = *min_element(a,a+6);
2.求数组最大值最小值对应的下标
1)vector容器
例 vector<int> vec
最大值下标:int maxPosition = max_element(v.begin(),v.end()) - v.begin();
最小值下标:int minPosition = min_element(v.begin(),v.end()) - v.begin();
2)普通数组
例 int a[]={1,2,3,4,5,6};
(数组名a就是地址)
最大值下标:int maxPosition = max_element(a,a+6) - a;
最小值下标:int minPosition = min_element(a,a+6) - a;
注意:返回的是第一个最大(小)元素的位置
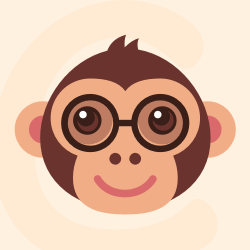



更多推荐
所有评论(0)