Python : Json Ascii <-> Hex互相转换
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
Python Tkinter : Json Ascii <-> Hex互转及Tkinter UI显示
使用背景
最近在做BLE设备端开发,为调试方便需要Json消息 Ascii-Hex格式互转,所以用tkinter简单做了一个图形化的Json Ascii - hex转换工具,照例分享一下下,开发过程还是习惯python随手打开一个Tools用着方便。。。
在嵌入式开发中,设备端获取的json数据通过16进制 hex进行存储和显示的,这时候就需要Ascii hex数据格式互相转换的工具,方便以Ascii核对具体json消息。
先上图:
Json Ascii -> Hex
Json Hex -> Ascii
代码解析 - Ascii-Hex转换
Asc-Hex直接使用binascii库函数,其实不止json,所有的ascii 都可以通过这个方法互转hex。。
def Ascii_to_Hex(ascii_str):
hex_str = binascii.hexlify(ascii_str.encode())
return hex_str
def Hex_to_Ascii(hex_str):
hex_str = hex_str.replace(' ', '').replace('0x', '').replace('\t', '').replace('\n', '')
ascii_str = binascii.unhexlify(hex_str.encode())
return ascii_str
代码解析 -Tkinter 图形界面,
界面很简单,主要就是两个Text框和三个Button,点击Button会获取输入Text框里面数据,然后调用上面函数对数据解析,再显示到Output Text里面,
这个结构也是我之前其他工具沿用的。。
root = Tk()
root.geometry('1000x600')
root.title(' Json Ascii<--> Hex Converter')
root.config(bg='#f0ffff')
#Lable
intro = Label(root,text='请在左侧输入要转换的消息,然后按相应按钮 HowieXue.blog.csdn.net',\
bg='#d3fbfb',\
fg='red',\
font=('华文新魏',14),\
width=20,\
height=2,\
relief=RIDGE)
intro.place(relx=0.1, rely=0.1, relwidth=0.8, relheight=0.1)
#Input
inputData = Text(root, font = ('',12))
inputData.place(relx=0.1, rely=0.2, relwidth=0.3, relheight=0.6)
#Output
txt = Text(root, font = ('',12))
txt.place(relx=0.6, rely=0.2, relwidth=0.3, relheight=0.6)
#Button
bt_json2hex = Button(root, text='Json -> Hex', command=json2hex, fg ='blue')
bt_json2hex.place(relx=0.4, rely=0.25, relwidth=0.2, relheight=0.1)
bt_hex2json = Button(root, text='Hex -> Json', command=hex2json, fg ='blue')
bt_hex2json.place(relx=0.4, rely=0.45, relwidth=0.2, relheight=0.1)
bt_clear = Button(root, text='Clear', command=clearContent, fg ='blue')
bt_clear.place(relx=0.4, rely=0.65, relwidth=0.2, relheight=0.1)
完整代码
# -*- coding: utf-8 -*-
#HowieXue
from tkinter import *
import binascii
def ascii_to_hex(ascii_str):
hex_str = binascii.hexlify(ascii_str.encode())
return hex_str
def hex_to_ascii(hex_str):
hex_str = hex_str.replace(' ', '').replace('0x', '').replace('\t', '').replace('\n', '')
ascii_str = binascii.unhexlify(hex_str.encode())
return ascii_str
def json2hex():
ascii_input = str(inputData.get(0.0,END))
#remove /n
ascii_input = ascii_input.strip("\n")
input_len = len(ascii_input)
hex_output = ascii_to_hex(ascii_input)
strHex = '{0}'.format(hex_output)
print('hex result is: '+ strHex)
#print('input Json msg len is: '+input_len)
strShow = ' >>Json -> Hex result:\n' + strHex
output.insert(END, strShow)
strShow = '\n >>Input Json msg length:\n' + '{0}\n'.format(hex(input_len))
output.insert(END, strShow)
#inputData.delete(0.0, END)
def hex2json():
hex_input = str(inputData.get(0.0, END))
# remove /n
hex_input = hex_input.strip("\n")
hex_output = hex_to_ascii(hex_input)
input_len = len(hex_output)
strjson = '{0}'.format(hex_output)
print('json result is: ' + strjson)
strShow = '\n >>Hex -> Json result:\n' + strjson
output.insert(END, strShow)
#strShow = '\n >> Json msg length:\n' + '{0}\n'.format(hex(input_len))
#output.insert(END, strShow)
# inputData.delete(0.0, END)
def clearContent():
inputData.delete(1.0, END)
output.delete(1.0,END)
root = Tk()
root.geometry('1000x600')
root.title(' Json Ascii<--> Hex Converter')
root.config(bg='#f0ffff')
#Lable
intro = Label(root,text='请在左侧输入要转换的消息,然后按相应按钮 HowieXue.blog.csdn.net',\
bg='#d3fbfb',\
fg='red',\
font=('华文新魏',14),\
width=20,\
height=2,\
relief=RIDGE)
intro.place(relx=0.1, rely=0.1, relwidth=0.8, relheight=0.1)
#Input
inputData = Text(root, font = ('',12))
inputData.place(relx=0.1, rely=0.2, relwidth=0.3, relheight=0.6)
#Output
output = Text(root, font = ('',12))
output.place(relx=0.6, rely=0.2, relwidth=0.3, relheight=0.6)
#Button
bt_json2hex = Button(root, text='Json -> Hex', command=json2hex, fg ='blue')
bt_json2hex.place(relx=0.4, rely=0.25, relwidth=0.2, relheight=0.1)
bt_hex2json = Button(root, text='Hex -> Json', command=hex2json, fg ='blue')
bt_hex2json.place(relx=0.4, rely=0.45, relwidth=0.2, relheight=0.1)
bt_clear = Button(root, text='Clear', command=clearContent, fg ='blue')
bt_clear.place(relx=0.4, rely=0.65, relwidth=0.2, relheight=0.1)
root.mainloop()
附:
使用过程中,如果有不同的消息类型,一般通过MsgHeader区分,可以使用CheckButton勾选,来实现对不同类型协议的解析,这和具体协议定义相关,相关代码就不在这里push了,仅举个checkbutton显示实例:
#checkButton
RWVar = IntVar() # 定义RWVar和FmtVar用来存放选择行为返回值
FmtVar = IntVar()
SegmVar = IntVar()
RWVar.set(1)
FmtVar.set(1)
readwrite = Checkbutton(root, text='Read',variable=RWVar, onvalue=1, offvalue=0, command=ConvertMsgHeader)
readwrite.place(relx=0.33, rely=0.85, relwidth=0.05, relheight=0.05)
dataformat = Checkbutton(root, text='Json',variable=FmtVar, onvalue=1, offvalue=0, command=ConvertMsgHeader)
dataformat.place(relx=0.23, rely=0.85, relwidth=0.05, relheight=0.05)
segmented = Checkbutton(root, text='NoSegment',variable=SegmVar, onvalue=1, offvalue=0, command=ConvertMsgHeader)
segmented.place(relx=0.41, rely=0.85, relwidth=0.09, relheight=0.05)
博主热门文章推荐:
一篇读懂系列:
LoRa Mesh系列:
网络安全系列:
- ATECC508A芯片开发笔记(一):初识加密芯片
- SHA/HMAC/AES-CBC/CTR 算法执行效率及RAM消耗 测试结果
- 常见加密/签名/哈希算法性能比较 (多平台 AES/DES, DH, ECDSA, RSA等)
- AES加解密效率测试(纯软件AES128/256)–以嵌入式Cortex-M0与M3 平台为例
嵌入式开发系列:
- 嵌入式学习中较好的练手项目和课题整理(附代码资料、学习视频和嵌入式学习规划)
- IAR调试使用技巧汇总:数据断点、CallStack、设置堆栈、查看栈使用和栈深度、Memory、Set Next Statement等
- Linux内核编译配置(Menuconfig)、制作文件系统 详细步骤
- Android底层调用C代码(JNI实现)
- 树莓派到手第一步:上电启动、安装中文字体、虚拟键盘、开启SSH等
- Android/Linux设备有线&无线 双网共存(同时上内、外网)
AI / 机器学习系列:
- AI: 机器学习必须懂的几个术语:Lable、Feature、Model…
- AI:卷积神经网络CNN 解决过拟合的方法 (Overcome Overfitting)
- AI: 什么是机器学习的数据清洗(Data Cleaning)
- AI: 机器学习的模型是如何训练的?(在试错中学习)
- 数据可视化:TensorboardX安装及使用(安装测试+实例演示)
其他好玩的python脚本
Python实现自动发送邮件 --自动抓取博客/网站中留言的邮箱并发送相应邮件
Python自动生成代码 - 通过tkinter图形化操作并生成代码框架
Python解析CSV数据 - 通过Pandas解析逻辑分析仪导出的CSV数据
Python通过Django搭建网站执行Lua脚本 (实现数据解析)
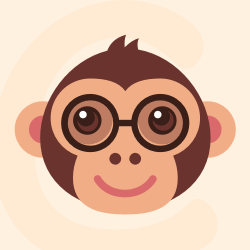



适用于现代 C++ 的 JSON。
最近提交(Master分支:3 个月前 )
f06604fc
* :page_facing_up: bump the copyright years
Signed-off-by: Niels Lohmann <mail@nlohmann.me>
* :page_facing_up: bump the copyright years
Signed-off-by: Niels Lohmann <mail@nlohmann.me>
* :page_facing_up: bump the copyright years
Signed-off-by: Niels Lohmann <niels.lohmann@gmail.com>
---------
Signed-off-by: Niels Lohmann <mail@nlohmann.me>
Signed-off-by: Niels Lohmann <niels.lohmann@gmail.com> 1 天前
d23291ba
* add a ci step for Json_Diagnostic_Positions
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* Update ci.cmake to address review comments
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* address review comment
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* fix typo in the comment
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* fix typos in ci.cmake
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* invoke the new ci step from ubuntu.yml
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* issue4561 - use diagnostic positions for exceptions
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* fix ci_test_documentation check
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* address review comments
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* fix ci check failures for unit-diagnostic-postions.cpp
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* improvements based on review comments
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* fix const correctness string
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* further refinements based on reviews
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* add one more test case for full coverage
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* ci check fix - add const
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* add unit tests for json_diagnostic_postions only
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* fix ci_test_diagnostics
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
* fix ci_test_build_documentation check
Signed-off-by: Harinath Nampally <harinath922@gmail.com>
---------
Signed-off-by: Harinath Nampally <harinath922@gmail.com> 1 天前
更多推荐
所有评论(0)