spring-boot-starter-quartz配置定时任务及Scheduling配置定时任务
spring-boot
spring-projects/spring-boot: 是一个用于简化Spring应用开发的框架。适合用于需要快速开发企业级Java应用的项目。特点是可以提供自动配置、独立运行和内置的Tomcat服务器,简化Spring应用的构建和部署。
项目地址:https://gitcode.com/gh_mirrors/sp/spring-boot

·
1.spring-boot-starter-quartz配置定时任务
引入依赖(不要找错):
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-quartz -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-quartz</artifactId>
<version>2.0.1.RELEASE</version>
</dependency>
执行类:
package com.demo.weather.job;
import java.util.List;
import org.quartz.JobExecutionContext;
import org.quartz.JobExecutionException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.scheduling.quartz.QuartzJobBean;
/**
* Weather Data Sync Job.
*
* @since 1.0.0 2017年11月23日
* @author <a href="https://waylau.com">Way Lau</a>
*/
public class WeatherDataSyncJob extends QuartzJobBean {
private final static Logger logger = LoggerFactory.getLogger(WeatherDataSyncJob.class);
/* (non-Javadoc)
* @see org.springframework.scheduling.quartz.QuartzJobBean#executeInternal(org.quartz.JobExecutionContext)
*/
@Override
protected void executeInternal(JobExecutionContext context) throws JobExecutionException {
logger.info("Weather Data Sync Job. Start!");
logger.info("Weather Data Sync Job. End!"); //定时执行
}
}
logger.info("Weather Data Sync Job. Start!");
logger.info("Weather Data Sync Job. End!"); //定时执行
}
}
配置类:
package com.demo.weather.config;
import com.demo.weather.job.WeatherDataSyncJob;
import org.quartz.JobBuilder;
import org.quartz.JobDetail;
import org.quartz.SimpleScheduleBuilder;
import org.quartz.Trigger;
import org.quartz.TriggerBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
/**
* Quartz Configuration.
*
* @since 1.0.0 2017年11月23日
* @author <a href="https://waylau.com">Way Lau</a>
*/
@Configuration
public class QuartzConfiguration {
private static final int TIME = 2; // 更新频率
// JobDetail
@Bean
public JobDetail weatherDataSyncJobDetail() {
return JobBuilder.newJob(WeatherDataSyncJob.class).withIdentity("weatherDataSyncJob")
.storeDurably().build();
}
// Trigger
@Bean
public Trigger weatherDataSyncTrigger() {
SimpleScheduleBuilder schedBuilder = SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(TIME).repeatForever();
return TriggerBuilder.newTrigger().forJob(weatherDataSyncJobDetail())
.withIdentity("weatherDataSyncTrigger").withSchedule(schedBuilder).build();
}
}
private static final int TIME = 2; // 更新频率
// JobDetail
@Bean
public JobDetail weatherDataSyncJobDetail() {
return JobBuilder.newJob(WeatherDataSyncJob.class).withIdentity("weatherDataSyncJob")
.storeDurably().build();
}
// Trigger
@Bean
public Trigger weatherDataSyncTrigger() {
SimpleScheduleBuilder schedBuilder = SimpleScheduleBuilder.simpleSchedule()
.withIntervalInSeconds(TIME).repeatForever();
return TriggerBuilder.newTrigger().forJob(weatherDataSyncJobDetail())
.withIdentity("weatherDataSyncTrigger").withSchedule(schedBuilder).build();
}
}
2.Scheduling配置定时任务(quartz视乎不能定多少点的任务,所以用这个)
package com.yizhen;
import com.yizhen.dataobject.MidTable;
import com.yizhen.service.MidTableService;
import com.yizhen.service.RoomService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.List;
@SpringBootApplication
@EnableScheduling
public class HotelmanageApplication {
@Autowired
private RoomService roomService;
@Autowired
private MidTableService midTableService;
public static void main(String[] args) {
SpringApplication.run(HotelmanageApplication.class, args);
}
// 每分钟启动
//@Scheduled(cron = "0 0/1 * * * ?")
@Scheduled(cron = "0 0 2 * * ?")//凌晨两点执行
public void updatejiageandfangjianshuliang(){
Date now = new Date();
SimpleDateFormat sf = new SimpleDateFormat("yyyy-MM-dd");
String nowDay = sf.format(now);
List<MidTable> midTableList=midTableService.findnowmidtables(nowDay);
for(MidTable midTable:midTableList){
Integer roomid=midTable.getMidTablePk().getRoom_id();
roomService.updateroomnumbyfangtaiweihu(Integer.valueOf(midTable.getFangtai()),roomid);
roomService.updateroompricebyfangtaiweihu(midTable.getPrice(),roomid);
}
System.out.println("更新成功!");
}
}
@EnableScheduling
public class HotelmanageApplication {
@Autowired
private RoomService roomService;
@Autowired
private MidTableService midTableService;
public static void main(String[] args) {
SpringApplication.run(HotelmanageApplication.class, args);
}
// 每分钟启动
//@Scheduled(cron = "0 0/1 * * * ?")
@Scheduled(cron = "0 0 2 * * ?")//凌晨两点执行
public void updatejiageandfangjianshuliang(){
Date now = new Date();
SimpleDateFormat sf = new SimpleDateFormat("yyyy-MM-dd");
String nowDay = sf.format(now);
List<MidTable> midTableList=midTableService.findnowmidtables(nowDay);
for(MidTable midTable:midTableList){
Integer roomid=midTable.getMidTablePk().getRoom_id();
roomService.updateroomnumbyfangtaiweihu(Integer.valueOf(midTable.getFangtai()),roomid);
roomService.updateroompricebyfangtaiweihu(midTable.getPrice(),roomid);
}
System.out.println("更新成功!");
}
}
启动类中配置
完整内容请参考:http://blog.maptoface.com/post/31
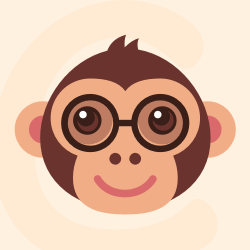



spring-projects/spring-boot: 是一个用于简化Spring应用开发的框架。适合用于需要快速开发企业级Java应用的项目。特点是可以提供自动配置、独立运行和内置的Tomcat服务器,简化Spring应用的构建和部署。
最近提交(Master分支:4 个月前 )
b444b8d3
* pr/43176:
Polish "Tighten rules around profile naming"
Tighten rules around profile naming
Closes gh-43176
4 小时前
53223529
See gh-43176
5 小时前
更多推荐
所有评论(0)