GitCode 开源社区
JSON使用JsonProperty Attribute
JSON使用JsonProperty Attribute
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

一、JSON使用JsonPropertyAttribute重命名属性名
1.先创建一个Movie对象,然后在其属性上添加JsonProperty,并指定重命名的名称。注意:属性Name和Director已指定。
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using GongHuiNewtonsoft.Json;
- namespace JSONDemo
- {
- public class Movie
- {
- [JsonProperty("name")]
- public string Name { get; set; }
- [JsonProperty("Chinese Director")]
- public string Director { get; set; }
- public int ReleaseYear { get; set; }
- }
- }
2.实例化Movie对象,然后序列化。
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Data;
- using GongHuiNewtonsoft.Json;
- using GongHuiNewtonsoft.Json.Serialization;
- using GongHuiNewtonsoft.Json.Converters;
- namespace JSONDemo
- {
- class Program
- {
- static void Main(string[] args)
- {
- Movie m = new Movie
- {
- Name = "非诚勿扰1",
- Director = "冯小刚",
- ReleaseYear = 2008
- };
- string json = JsonConvert.SerializeObject(m, Formatting.Indented);
- Console.WriteLine(json);
- }
- }
- }
3.运行结果,注意:属性ReleaseYear未被重命名。
二、JSON使用JsonPropertyAttribute序列化升序排序属性
1.先创建一个Movie对象,然后指定JsonProperty,并添加Order属性。
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using GongHuiNewtonsoft.Json;
- namespace JSONDemo
- {
- public class Movie
- {
- [JsonProperty(Order=4)]
- public string Name { get; set; }
- [JsonProperty(Order=0)]
- public string Director { get; set; }
- public int ReleaseYear { get; set; }
- [JsonProperty(Order=-3)]
- public string ChiefActor { get; set; }
- [JsonProperty(Order=2)]
- public string ChiefActress { get; set; }
- }
- }
2.实例化Movie对象,然后序列化。
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Data;
- using GongHuiNewtonsoft.Json;
- using GongHuiNewtonsoft.Json.Serialization;
- using GongHuiNewtonsoft.Json.Converters;
- namespace JSONDemo
- {
- class Program
- {
- static void Main(string[] args)
- {
- Movie m = new Movie
- {
- Name = "非诚勿扰1",
- Director = "冯小刚",
- ReleaseYear = 2008,
- ChiefActor="葛优",
- ChiefActress="舒淇"
- };
- string json = JsonConvert.SerializeObject(m, Formatting.Indented);
- Console.WriteLine(json);
- }
- }
- }
3.运行结果。注意:未指定Order序号的属性,界定于大于负数小于正数,并按默认顺序排序。
三、JSON使用JsonPropertyAttribute反序列化属性时,Required指定属性性质
1.创建一个Movie对象,给属性添加JsonProperty,并指定其Required的性质。属性Name必须有值,DateTime可以为空.
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using GongHuiNewtonsoft.Json;
- namespace JSONDemo
- {
- public class Movie
- {
- [JsonProperty(Required=Required.Always)]
- public string Name { get; set; }
- [JsonProperty(Required = Required.AllowNull)]
- public DateTime? ReleaseDate { get; set; }
- public string Director { get; set; }
- }
- }
2.实例化Movie对象,反序列化JSON。
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Data;
- using GongHuiNewtonsoft.Json;
- using GongHuiNewtonsoft.Json.Serialization;
- using GongHuiNewtonsoft.Json.Converters;
- namespace JSONDemo
- {
- class Program
- {
- static void Main(string[] args)
- {
- string json = @"{
- 'Name':'举起手来1',
- 'Director':'冯小宁',
- 'ReleaseDate':null
- }";
- Movie m = JsonConvert.DeserializeObject<Movie>(json);
- Console.WriteLine(m.Name);
- Console.WriteLine(m.Director);
- Console.WriteLine(m.ReleaseDate);
- }
- }
- }
3.运行结果是
四、JSON使用JsonPropertyAttribute序列化引用类型集合
1.创建一个Director对象,并声明一个本身类型的属性,指定JsonProperty中的IsReference为true.
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using GongHuiNewtonsoft.Json;
- namespace JSONDemo
- {
- public class Director
- {
- public string Name { get; set; }
- [JsonProperty(IsReference=true)]
- public Director ExecuteDir { get; set; }
- }
- }
2.创建一个Movie对象,声明一个Director集合的属性,指定JsonProperty中的IsReference为true.
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using GongHuiNewtonsoft.Json;
- namespace JSONDemo
- {
- public class Movie
- {
- public string Name { get; set; }
- [JsonProperty(ItemIsReference=true)]
- public IList<Director> Directors { get; set; }
- }
- }
3.序列化对象
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Data;
- using GongHuiNewtonsoft.Json;
- using GongHuiNewtonsoft.Json.Serialization;
- using GongHuiNewtonsoft.Json.Converters;
- namespace JSONDemo
- {
- class Program
- {
- static void Main(string[] args)
- {
- Director dir = new Director
- {
- Name = "冯小刚"
- };
- Director dir1 = new Director
- {
- Name = "张艺谋",
- ExecuteDir = dir
- };
- Movie m = new Movie
- {
- Name = "满城尽带黄金甲",
- Directors = new List<Director>
- {
- dir,
- dir1
- }
- };
- string json = JsonConvert.SerializeObject(m, Formatting.Indented);
- Console.WriteLine(json);
- }
- }
- }
4.运行结果
五、JSON使用JsonPropertyAttribute序列化忽略属性null
1.创建一个Movie对象,并在属性上指定JsonProperty,添加NullValueHandling,忽略null
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using GongHuiNewtonsoft.Json;
- namespace JSONDemo
- {
- public class Movie
- {
- public string Name { get; set; }
- public string Director { get; set; }
- [JsonProperty(NullValueHandling=NullValueHandling.Ignore)]
- public DateTime? LaunchDate { get; set; }
- }
- }
2.实例化对象Movie对象,然后序列化
[csharp]
view plain
copy
- using System;
- using System.Collections.Generic;
- using System.Linq;
- using System.Text;
- using System.Data;
- using GongHuiNewtonsoft.Json;
- using GongHuiNewtonsoft.Json.Serialization;
- using GongHuiNewtonsoft.Json.Converters;
- namespace JSONDemo
- {
- class Program
- {
- static void Main(string[] args)
- {
- Movie m = new Movie
- {
- Name = "爱情呼叫转移",
- Director = "张建亚"
- };
- string json = JsonConvert.SerializeObject(m, Formatting.Indented);
- Console.WriteLine(json);
- }
- }
- }
3.运行的结果
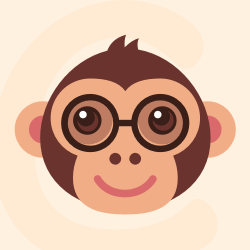



适用于现代 C++ 的 JSON。
最近提交(Master分支:1 个月前 )
960b763e
3 个月前
8c391e04
6 个月前
更多推荐
7951
2
0
- 0
扫一扫分享内容
分享
回到
顶部
顶部
所有评论(0)