Druid简单使用(一)
druid
阿里云计算平台DataWorks(https://help.aliyun.com/document_detail/137663.html) 团队出品,为监控而生的数据库连接池
项目地址:https://gitcode.com/gh_mirrors/druid/druid

·
注意:此例子使用eclipse+maven,请确保您的eclipse中已成功配置了mave
- 在eclipse中创建一个maven项目----------项目类型(maven-archetype-webapp)
- 在pom.xml文件中添加对包的依赖
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.hlj.druid</groupId>
<artifactId>druid_Dome1</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>druid_Dome1 Maven Webapp</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jsp-api</artifactId>
<version>2.0</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.0.27</version>
</dependency>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.0.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.34</version>
</dependency>
</dependencies>
<build>
<finalName>druid_Dome1</finalName>
</build>
</project>
- 对上面使用的包进行简单说明:jsp-api是对jsp的支持、servlet-api是对servlet的支持、druid是连接池的包、mysql-connector-java是java操作mysql数据库的包
public class DbPoolConnection {
private static DbPoolConnection databasePool=null;
private static DruidDataSource dds = null;
static {
Properties properties = loadPropertyFile("db_server.properties");
try {
dds = (DruidDataSource) DruidDataSourceFactory
.createDataSource(properties);
} catch (Exception e) {
e.printStackTrace();
}
}
private DbPoolConnection() {}
public static synchronized DbPoolConnection getInstance() {
if (null == databasePool) {
databasePool = new DbPoolConnection();
}
return databasePool;
}
public DruidPooledConnection getConnection() throws SQLException {
return dds.getConnection();
}
public static Properties loadPropertyFile(String fullFile) {
/*String webRootPath = null;
if (null == fullFile || fullFile.equals(""))
throw new IllegalArgumentException(
"Properties file path can not be null : " + fullFile);
webRootPath = DbPoolConnection.class.getClassLoader().getResource("")
.getPath();
//getParent()获取webRootPath的父级目录
webRootPath = new File(webRootPath).getParent();
System.out.println(webRootPath);
InputStream inputStream = null;
Properties p = null;
try {
inputStream = new FileInputStream(new File(webRootPath
+ File.separator + fullFile));
p = new Properties();
p.load(inputStream);
} catch (FileNotFoundException e) {
throw new IllegalArgumentException("Properties file not found: "
+ fullFile);
} catch (IOException e) {
throw new IllegalArgumentException(
"Properties file can not be loading: " + fullFile);
} finally {
try {
if (inputStream != null)
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return p;*/
Properties p = new Properties();
if(fullFile == "" || fullFile.equals(""))
{
System.out.println("属性文件为空!~");
}
else
{
//加载属性文件
InputStream inStream = DbPoolConnection.class.getClassLoader().getResourceAsStream(fullFile);
try {
p.load(inStream);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return p;
}
}
- 通过上面的代码可以看出Druid DAO实用了DruidDataSource的工厂模式DruidDataSourceFactory,此工厂模式极大的简化了我们实用Druid的开发过程,只需通过DruidDataSourceFactory的创建方法就可以获得DataSource实例
- 在上面类中使用的db_server.properties配置文件的配置如下:
driverClassName=com.mysql.jdbc.Driver
url=jdbc:mysql://localhost/druidDom
username=root
password=123456
filters=stat
initialSize=2
maxActive=300
maxWait=60000
timeBetweenEvictionRunsMillis=60000
minEvictableIdleTimeMillis=300000
validationQuery=SELECT 1
testWhileIdle=true
testOnBorrow=false
testOnReturn=false
poolPreparedStatements=false
maxPoolPreparedStatementPerConnectionSize=200
- 这个配置可以根据个人项目的实际情况进行修改。
- 使用实例:
private void executeUpdateBySQL(String sql) throws SQLException {
DbPoolConnection dbp = DbPoolConnection.getInstance();
DruidPooledConnection con = dbp.getConnection();
PreparedStatement ps = con.prepareStatement(sql);
ps.executeUpdate();
ps.close();
con.close();
dbp = null;
}
- 以上只是druid数据库连接池的简单使用,仅供参考,大神勿喷!
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.hlj.druid</groupId> <artifactId>druid_Dome1</artifactId> <packaging>war</packaging> <version>0.0.1-SNAPSHOT</version> <name>druid_Dome1 Maven Webapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>javax.servlet</groupId> <artifactId>jsp-api</artifactId> <version>2.0</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>druid</artifactId> <version>1.0.27</version> </dependency> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.0.1</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.34</version> </dependency> </dependencies> <build> <finalName>druid_Dome1</finalName> </build> </project>
public class DbPoolConnection {
private static DbPoolConnection databasePool=null;
private static DruidDataSource dds = null;
static {
Properties properties = loadPropertyFile("db_server.properties");
try {
dds = (DruidDataSource) DruidDataSourceFactory
.createDataSource(properties);
} catch (Exception e) {
e.printStackTrace();
}
}
private DbPoolConnection() {}
public static synchronized DbPoolConnection getInstance() {
if (null == databasePool) {
databasePool = new DbPoolConnection();
}
return databasePool;
}
public DruidPooledConnection getConnection() throws SQLException {
return dds.getConnection();
}
public static Properties loadPropertyFile(String fullFile) {
/*String webRootPath = null;
if (null == fullFile || fullFile.equals(""))
throw new IllegalArgumentException(
"Properties file path can not be null : " + fullFile);
webRootPath = DbPoolConnection.class.getClassLoader().getResource("")
.getPath();
//getParent()获取webRootPath的父级目录
webRootPath = new File(webRootPath).getParent();
System.out.println(webRootPath);
InputStream inputStream = null;
Properties p = null;
try {
inputStream = new FileInputStream(new File(webRootPath
+ File.separator + fullFile));
p = new Properties();
p.load(inputStream);
} catch (FileNotFoundException e) {
throw new IllegalArgumentException("Properties file not found: "
+ fullFile);
} catch (IOException e) {
throw new IllegalArgumentException(
"Properties file can not be loading: " + fullFile);
} finally {
try {
if (inputStream != null)
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return p;*/
Properties p = new Properties();
if(fullFile == "" || fullFile.equals(""))
{
System.out.println("属性文件为空!~");
}
else
{
//加载属性文件
InputStream inStream = DbPoolConnection.class.getClassLoader().getResourceAsStream(fullFile);
try {
p.load(inStream);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return p;
}
}
driverClassName=com.mysql.jdbc.Driver
url=jdbc:mysql://localhost/druidDom
username=root
password=123456
filters=stat
initialSize=2
maxActive=300
maxWait=60000
timeBetweenEvictionRunsMillis=60000
minEvictableIdleTimeMillis=300000
validationQuery=SELECT 1
testWhileIdle=true
testOnBorrow=false
testOnReturn=false
poolPreparedStatements=false
maxPoolPreparedStatementPerConnectionSize=200
private void executeUpdateBySQL(String sql) throws SQLException {
DbPoolConnection dbp = DbPoolConnection.getInstance();
DruidPooledConnection con = dbp.getConnection();
PreparedStatement ps = con.prepareStatement(sql);
ps.executeUpdate();
ps.close();
con.close();
dbp = null;
}
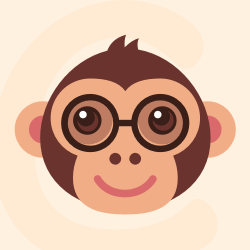



阿里云计算平台DataWorks(https://help.aliyun.com/document_detail/137663.html) 团队出品,为监控而生的数据库连接池
最近提交(Master分支:3 个月前 )
f060c270 - 8 天前
1613a765
* Improve gaussdb ddl parser
* fix temp table 9 天前
更多推荐
所有评论(0)