Spring-Boot添加MyBatis:手动添加代码方式

创建了一个MySQL数据库,并添加了一张表:
添加MyBatis后,有两种使用方式:
- 注解方式。简单快速,适合规模较小的数据库。
- xml配置方式。支持动态生成SQL,调整SQL更方便,适合大型数据库。
无论哪种方式,都需要共同执行的前期工作:
- 在pom.xml中添加依赖库。
<!-- 添加mybatis --> <dependency> <groupId>org.mybatis.spring.boot</groupId> <artifactId>mybatis-spring-boot-starter</artifactId> <version>1.3.2</version> </dependency> <!-- 添加mysql驱动 --> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency>
- 在application.properites中添加配置项来配置数据库。
spring.datasource.driverClassName = com.mysql.jdbc.Driver spring.datasource.url = jdbc:mysql://localhost:3306/mytest?useUnicode=true&characterEncoding=utf-8 spring.datasource.username = root spring.datasource.password = 123456
这些配置项的key都是固定的,只需要修改后面的值。
- 创建表对应的实体类Student。
import java.io.Serializable; public class Student implements Serializable { private String id = ""; private String name = ""; private Integer age = 0; public String getId() { return id; } public void setId(String id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } }
-
注解方式
- 在src/main/java/com/template/dao文件夹下创建操作表用的mapper.java,其中引用了实体类Student。
import com.template.model.Student; import org.apache.ibatis.annotations.*; public interface StudentMapper { @Select("select * from student where id = ${id}") @Results({ @Result(property = "id", column = "id"), @Result(property = "name", column = "name"), @Result(property = "age", column = "age") }) Student get(@Param("id") String id); }
该mapper文件是一个interface,每个接口都需要使用@Select /@Insert/@Update/@Delete等注解来添加SQL语句。对于需要返回值的,还要使用@Results来定义返回的数据。
特别注意,要在程序入口文件application类前添加注解:
@MapperScan("com.template.dao")
这样spring才会将该文件夹下的所有文件扫描为mapper文件,spring才会识别它们。
当然也可以在每个mapper文件上方添加@Mapper注解,只是这样就意味着每个mapper文件都要添加一次,比较麻烦。
- 在需要的地方通过@Autowired引入mapper文件,调用接口来操作数据表。
引入:
@Autowired private StudentMapper studentMapper;
调用:
Student student = studentMapper.get("201802"); String name = student.getName(); Integer age = student.getAge();
-
xml配置方式
- 在src/main/java/resources/下添加一个mybatis文件夹,在其下添加一个mybatis-config.xml文件,然后添加一个mapper文件夹,并在mapper文件夹下添加一个studentMapper.xml文件。添加完成后,结构如下:
然后修改application.properties,添加:
mybatis.config-location = classpath:mybatis/mybatis-config.xml mybatis.mapper-locations = classpath:mybatis/mapper/*.xml
这两个配置项指定了刚才添加的mybatis-config.xml配置文件以及mapper文件夹下所有的映射xml文件。
- 打开mybatis-config.xml,将内容修改为:
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <typeAliases> <typeAlias alias="Integer" type="java.lang.Integer" /> <typeAlias alias="Long" type="java.lang.Long" /> <typeAlias alias="HashMap" type="java.util.HashMap" /> <typeAlias alias="LinkedHashMap" type="java.util.LinkedHashMap" /> <typeAlias alias="ArrayList" type="java.util.ArrayList" /> <typeAlias alias="LinkedList" type="java.util.LinkedList" /> </typeAliases> </configuration>
这样就为常用的数据类型定义了别名。
- 打开mapper/studentMapper.xml,将内容修改为:
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > <mapper namespace="com.template.dao.StudentMapper"> <resultMap id="BaseResultMap" type="com.template.model.Student"> <result column="id" property="id" jdbcType="VARCHAR"/> <result column="name" property="name" jdbcType="VARCHAR"/> <result column="age" property="age" jdbcType="INTEGER"/> </resultMap> <sql id="Base_Column_List"> id, name, age </sql> <select id="get" parameterType="java.lang.String" resultMap="BaseResultMap"> SELECT <include refid="Base_Column_List"/> FROM student WHERE id = #{id} </select> </mapper>
注意:
①namespace属性必须与即将创建的mapper.java文件路径相同。
②jdbcType务必确保正确,否则编译错误。 - 在src/main/java/com/template/dao文件夹下创建操作表用的mapper.java,其中引用了实体类Student。
import com.template.model.Student; public interface StudentMapper { Student get(String id); }
可以看到相比于注解的方式,该方式的mapper文件由于将SQL语句,参数,返回值等移到了上面3中的xml里,因此更加简洁。
同1,需要在程序入口文件application类前添加注解:
@MapperScan("com.template.dao")
- 在需要的地方通过@Autowired引入mapper文件,调用接口来操作数据表。
引入:@Autowired private StudentMapper studentMapper;
调用:
Student student = studentMapper.get("201802"); String name = student.getName(); Integer age = student.getAge();
可见两种方式的区别仅仅在于mapper的实现。最终的使用方式是相同的。
其他:
- xml文件路径问题
在properties配置文件中,使用classpath来指定基础路径,也就是target/classes文件夹。就像上面引入mybiatis-config.xml时:
mybatis.config-location = classpath:mybatis/mybatis-config.xml
该路径等同于:
target/classes/mybatis/mybatis-config.xml
spring-boot编译后会将各种编译和资源文件放到classes文件夹下,程序实际运行需要从classes文件下引用自己所需要的各种资源,例如xml文件。spring-boot的classpath就是classes文件夹。
而针对于mapper,使用上面的配置,则java类放在:
src/main/java/com/template/dao
文件夹下,而配套的xml文件放在:
src/main/resources/mybatis/mapper
文件夹下。
要添加一个数据库操作,就必须同时修改这两个文件,而这两个文件的目录相隔有点远,来回切换并不是特别方便。因此希望可以将这两个文件的目录放在一起,就像这样:
然而,这样放置,就是将xml文件放在了src/main/java路径下。存在的一个问题就是只有java-resource类型文件夹才会将自身的子文件复制到classes下。工程默认的java-resource类型文件夹只有一个,那就是src/main/resources。因此这样放置xml,是不会被复制到classes文件夹下的。
解决的方法是将该文件夹设置为Resources类型文件夹。
可以使用修改iml文件的方式,也可以使用 Resource Plugin:
在pom.xml中,<build>节点下添加一个新的resource路径:
<build>
<resources>
<resource>
<directory>src/main/java/com/template/mapper</directory>
<targetPath>mapper</targetPath>
</resource>
<resource>
<directory>src/main/resources</directory>
</resource>
</resources>
</build>
这样,src/main/java/com/template/mapper这个目录下的所有资源就会被复制到classes/mapper文件夹下,mapper路径是<targetPath>设置的。然后修改application.properties文件中mybatis.mapper-locations设置:
mybatis.mapper-locations = classpath: mapper/*.xml
即告诉工程说,去classes/mapper文件夹下取所有的xml文件。
重新编译,即可。
- 调用时提示Could not autowire问题
在调用时:
@Autowired
private StudentMapper studentMapper;
结果提示错误:
Could not autowire. No beans of 'StudentMapper' type found. more... (Ctrl+F1)
然而却可以正常运行。
有两种解决方案:
- 降低提示等级,从而看到的是低等级提示,不再是错误警告。
- 为IDEA安装MyBatis plugin。
MyBatis plugin收费,推荐使用1。
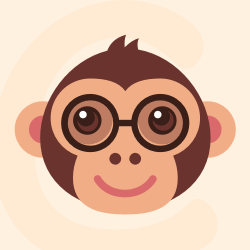



更多推荐
所有评论(0)