Druid使用步骤及详细案例(赞)
druid
阿里云计算平台DataWorks(https://help.aliyun.com/document_detail/137663.html) 团队出品,为监控而生的数据库连接池
项目地址:https://gitcode.com/gh_mirrors/druid/druid

·
Druid(德鲁伊) 是什么? 与 c3p0 和 dbcp 类似,Druid 是阿里的 Druid 数据库连接池。
文章目录
一、Druid 的使用步骤:
1.1 导入 jar 包:
druid-1.1.20.jar
1.2 定义配置文件:
a、编写 properties 文件。
b、文件名是任意的,可以在任意的目录下。
1.3 加载配置文件:
加载 druid.properties 文件到我们的项目中。
1.4 从数据库连接池工厂中获取连接池对象:
a、数据库连接池工厂 -> DruidDataSourceFactory。
b、获取连接池对象 -> createDataSource(Properties 对象)。
1.5 获取连接对象:
getConnection();
二、Druid 在项目中的部分:
项目目录结构:
2.1 导入 jar 文件:
2.2 定义配置文件:
druid.properties:
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql://localhost:3306/db_druid?useSSL=false&serverTimezone=UTC
username=root
password=root
initialSize=3
maxActive=5
maxWait=3000
2.3 加载配置文件:
public static DataSource ds;
static {
Properties properties = new Properties();
/**
* 利用反射获取类加载器,以此来得到加载项目中的其他文件
*/
InputStream resourceAsStream = JDBCUtils.class.getClassLoader().getResourceAsStream("druid.properties");
try {
// 加载配置文件 druid.properties
properties.load(resourceAsStream);
// 获取连接池对象
ds = DruidDataSourceFactory.createDataSource(properties);
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
if(resourceAsStream != null) {
try {
resourceAsStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
2.4 从数据库连接池工厂中获取连接池对象:
public static DataSource getDataSource(){
return ds;
}
2.5 获取连接对象:
/**
* 从连接池中获取一个数据库的连接
* @return
* @throws SQLException
*/
public static Connection getConnection() throws SQLException {
return ds.getConnection();
}
2.6 关闭数据库连接:
/**
*
* 关闭数据库的连接
* @param set
* @param connection
* @param statement
* @throws SQLException
*/
public static void close(ResultSet set, Connection connection, Statement statement) throws SQLException {
if(set != null) {
set.close();
}
if(connection != null){
connection.close();
}
if(statement != null) {
statement.close();
}
}
三、帖子点赞数 完整项目:
3.1 数据库设计:
3.2 项目目录结构:
3.3 JDBCUtils:
package com.xptx.druid.utils;
import com.alibaba.druid.pool.DruidDataSource;
import com.alibaba.druid.pool.DruidDataSourceFactory;
import javax.sql.DataSource;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Properties;
public class JDBCUtils {
public static DataSource ds;
static {
Properties properties = new Properties();
/**
* 利用反射获取类加载器,以此来得到加载项目中的其他文件
*/
InputStream resourceAsStream = JDBCUtils.class.getClassLoader().getResourceAsStream("druid.properties");
try {
// 加载配置文件 druid.properties
properties.load(resourceAsStream);
// 获取连接池对象
ds = DruidDataSourceFactory.createDataSource(properties);
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
if(resourceAsStream != null) {
try {
resourceAsStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public static DataSource getDataSource(){
return ds;
}
/**
* 从连接池中获取一个数据库的连接
* @return
* @throws SQLException
*/
public static Connection getConnection() throws SQLException {
return ds.getConnection();
}
/**
*
* 关闭数据库的连接
* @param set
* @param connection
* @param statement
* @throws SQLException
*/
public static void close(ResultSet set, Connection connection, Statement statement) throws SQLException {
if(set != null) {
set.close();
}
if(connection != null){
connection.close();
}
if(statement != null) {
statement.close();
}
}
}
3.4 Dao :
package com.xptx.druid.dao;
import com.xptx.druid.entity.Tip;
import com.xptx.druid.utils.JDBCUtils;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class TipDao {
/**
* 在帖子中添加一条记录
*/
public void add(Tip tip) throws SQLException {
Integer id = tip.getId();
String name = tip.getName();
Integer good = tip.getGood();
Integer bad = tip.getBad();
Date time = tip.getDate();
/**
* 将日期进行格式化,转换成 String 字符串形式
*/
SimpleDateFormat f = new SimpleDateFormat("yyyy-MM-dd");
String time1 = f.format(time);
String title = tip.getTitle();
// 获取连接
Connection conn = JDBCUtils.getConnection();
String sql = "insert into tip values (?,?,?,?,?,2,?)";
PreparedStatement pstam = conn.prepareStatement(sql);
pstam.setInt(1,id);
pstam.setString(2,name);
pstam.setInt(3,good);
pstam.setInt(4,bad);
pstam.setString(5,time1);
pstam.setString(6,title);
int result = pstam.executeUpdate();
if(result > 0) {
System.out.println("添加成功!");
}
JDBCUtils.close(null,conn,pstam);
}
/**
* 删除指定用户的所有帖子
*/
public void delete(String name) throws SQLException {
Connection conn = JDBCUtils.getConnection();
String sql = "delete from tip where tname = ?";
PreparedStatement pstam = conn.prepareStatement(sql);
pstam.setString(1,name);
int result = pstam.executeUpdate();
if(result > 0) {
System.out.println("删除成功!");
} else {
System.out.println("删除失败!");
}
JDBCUtils.close(null,conn,pstam);
}
/**
* 将小潘同学的点赞数 + 20%
*
*/
public void update(String name) throws SQLException {
Connection conn = JDBCUtils.getConnection();
String sql = "update tip set tgood = 1.2 * tgood where tname = ?";
PreparedStatement pstam = conn.prepareStatement(sql);
pstam.setString(1,name);
pstam.executeUpdate();
JDBCUtils.close(null,conn,pstam);
}
/**
* 查询帖子中带有 “同学” 字样的帖子
*/
public void select(String info) throws SQLException {
Connection conn = JDBCUtils.getConnection();
String sql = "select * from tip where tname LIKE ?";
PreparedStatement pstam = conn.prepareStatement(sql);
pstam.setString(1,"%"+info+"%");
ResultSet resultSet = pstam.executeQuery();
while (resultSet.next()) {
Integer id = resultSet.getInt(1);
String name = resultSet.getString(2);
Integer good = resultSet.getInt(3);
Integer bad = resultSet.getInt(4);
String time = resultSet.getString(5);
String title = resultSet.getString(7);
System.out.println(id +"----" +name + "----" + good +"----" + bad + "----" + time + "----" +title );
}
JDBCUtils.close(resultSet,conn,pstam);
}
}
3.5 Entity :
package com.xptx.druid.entity;
import java.util.Date;
public class Tip {
private Integer id;
private String name;
private Integer good;
private Integer bad;
private Date date;
private String title;
public Tip() {
}
public Tip(Integer id, String name, Integer good, Integer bad, Date date, String title) {
this.id = id;
this.name = name;
this.good = good;
this.bad = bad;
this.date = date;
this.title = title;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getGood() {
return good;
}
public void setGood(Integer good) {
this.good = good;
}
public Integer getBad() {
return bad;
}
public void setBad(Integer bad) {
this.bad = bad;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
@Override
public String toString() {
return "Tip{" +
"id=" + id +
", name='" + name + '\'' +
", good=" + good +
", bad=" + bad +
", date=" + date +
", title='" + title + '\'' +
'}';
}
}
3.6 Test:
package com.xptx.druid.test;
import com.xptx.druid.dao.TipDao;
import com.xptx.druid.entity.Tip;
import java.sql.SQLException;
import java.util.Date;
public class Test {
public static void main(String[] args) throws SQLException {
Tip tip = new Tip(3,"赵四同学",1,123,new Date(),"小美眉对我笑了");
TipDao tipDao = new TipDao();
// tipDao.add(tip);
// tipDao.delete("xptx");
// tipDao.update("小潘同学");
tipDao.select("同学");
}
}
四、后记:
本文只是 Druid(德鲁伊) 的一个简单实用,该数据库连接池的强大之处还没有运用到,比如 Druid 的监控功能,这也是 Druid 比 c3p0 和 dbcp 连接池厉害的地方,由于博主比较菜,之后再进一步学习 Druid 强大的地方。
如果该文对您有帮助,是小潘的荣幸,不如点个赞,点个关注给点鼓励,嘻嘻,在此先谢过啦。
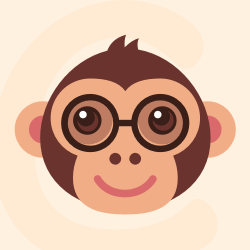



阿里云计算平台DataWorks(https://help.aliyun.com/document_detail/137663.html) 团队出品,为监控而生的数据库连接池
最近提交(Master分支:3 个月前 )
f060c270 - 7 天前
1613a765
* Improve gaussdb ddl parser
* fix temp table 8 天前
更多推荐
所有评论(0)