Unity中SimpleJSON的使用
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
导入DLL
To use SimpleJSON in Unity
DLL下载链接
里面除了DLL,还有两个C#的解析脚本。
脚本
其中一个脚本JsonParser,是要用到的文件读取脚本:
using SimpleJSON;
using System.IO;
using UnityEngine;
public class JsonParser
{
//从外边引进的json文件用ReadJson2;
//create创建的json文件用ReadJson;
string _StrPathURL;
public JsonParser()
{
#region 以前的多平台
//#if UNITY_IPHONE
// _StrPathURL = Application.dataPath + "/Raw/";
//#elif UNITY_STANDALONE_WIN || UNITY_EDITOR
// _StrPathURL = Application.streamingAssetsPath + "/";
//#elif UNITY_ANDROID
// _StrPathURL = Application.persistentDataPath + "/";
//#else
// _StrPathURL=string.Empty;
//#endif
#endregion
#if WINDOWS || UNITY_EDITOR
_StrPathURL = Application.streamingAssetsPath + "/";
#elif ANDROID
//_StrPathURL = Application.persistentDataPath + "/";
_StrPathURL = "jar:file://" + Application.dataPath + "!/assets/";
#else
_StrPathURL = string.Empty;
#endif
}
/// <summary>
/// 序列化
/// </summary>
public void SerializeJsonNode(string path, JSONNode Node)
{
var dataFile = _StrPathURL + path;
using (var fs = new FileStream(dataFile, FileMode.OpenOrCreate))
{
using (var bw = new BinaryWriter(fs))
{
bw.Flush();
Node.Serialize(bw);
Node.SaveToCompressedBase64();
//Debug.Log("创建ok");
bw.Close();
fs.Close();
}
}
}
/// <summary>
/// 对象序列化
/// </summary>
public void SerializeJsonClass(string path, JSONClass Obj)
{
var dataFile = _StrPathURL + path;
using (var fs = new FileStream(dataFile, FileMode.OpenOrCreate))
{
using (var bw = new BinaryWriter(fs))
{
bw.Flush();
Obj.Serialize(bw);
Obj.SaveToCompressedBase64();
//Debug.Log("创建ok");
bw.Close();
fs.Close();
}
}
}
/// <summary>
/// 数组序列化
/// </summary>
public void SerializeJsonArray(string path, JSONArray arr)
{
var dataFile = _StrPathURL + path;
using (var fs = new FileStream(dataFile, FileMode.OpenOrCreate))
{
using (var bw = new BinaryWriter(fs))
{
bw.Flush();
arr.Serialize(bw);
arr.SaveToCompressedBase64();
//Debug.Log("创建ok");
bw.Close();
fs.Close();
}
}
}
/// <summary>
/// 反序列化 JsonNode
/// </summary>
public JSONNode DeserializeJson(string route)
{
var dataFile = _StrPathURL + route;
JSONNode node = null;
using (var br = new BinaryReader(File.OpenRead(dataFile)))
{
node = JSONNode.Deserialize(br);
//Debug.Log("读取node成功" + node.ToString());
br.Close();
}
return node;
}
/// <summary>
/// 判断文件是否存在------安卓上无法使用
/// </summary>
/// <returns><c>true</c> if this instance is file exits the specified str; otherwise, <c>false</c>.</returns>
/// <param name="str">文件名.</param>
public bool IsFileExits(string str)
{
string dataFile = _StrPathURL + str;
return File.Exists(dataFile);
}
/// <summary>
/// 读非json格式的文档
/// </summary>
public JSONNode ReadJson(string route)
{
string dataFile = _StrPathURL + route;
JSONNode node = null;
#if UNITY_ANDROID
WWW www = new WWW(dataFile);
while (!www.isDone)
{ }
node = JSONNode.Parse(www.text);
//Debug.Log("读取node成功" + node);
#elif UNITY_STANDALONE_WIN || UNITY_EDITOR
string str = File.ReadAllText(dataFile);
node = JSONNode.Parse(str);
//Debug.Log("读取node成功" + node.ToString());
#endif
return node;
}
}
我这里编写了一个管理者便于之后的代码编写:
using SimpleJSON;
using System.Collections.Generic;
public class JsonDataManager<R, S, T> : Singleton<T>
where R : BaseData, new()
where S : JSONNode, new()
where T : JsonDataManager<R, S, T>
{
protected JsonDataManager() : base()
{
m_parser = new JsonParser();
}
protected JsonParser m_parser = null;
public string m_JsonFileName;
/// <summary>
/// JSON对象
/// </summary>
protected S m_json = null;
protected void Init(bool isDeserialize = false)
{
bool isFileExits = true;
#if WINDOWS || IPHONE || UNITY_EDITOR
isFileExits = m_parser.IsFileExits(m_JsonFileName);
#elif ANDROID
isFileExits = true;
#endif
if (isFileExits)
{
if (isDeserialize)
{
m_json = m_parser.DeserializeJson(m_JsonFileName) as S;
}
else
{
m_json = m_parser.ReadJson(m_JsonFileName) as S;
}
}
else
{
m_json = new S();
}
}
}
/// <summary>
/// 数据父类
/// </summary>
public class BaseData
{
}
脚本中BaseData类为数据类型,里面设置每个json文件里对应的每个键的字段。管理者中分别有链表和字典两种储存方式,以及DeserializeInit和ReadInit两种读取文件的方式。DeserializeInit用于读取通过程序序列化而得到的json文件;ReadInit用于读取通过自己手动输入建立的json文件,这里分享两个网页版的json数据编辑器(链接),编辑好的数据可以直接导出,很好用。
Singleton<T>
是单例
使用示例
举个栗子:
using System.Collections.Generic;
using SimpleJSON;
/// <summary>
/// 介绍数据管理者
/// </summary>
public class IntrDataManager : JsonDataManager<IntrData, JSONArray, IntrDataManager>
{
protected IntrDataManager() : base()
{
m_JsonFileName = "IntrData.json";
Init();
for (int i = 0; i < m_json.Count; i++)
{
IntrData data = new IntrData();
data.Name = m_json[i]["Name"];
data.NeedHeight = m_json[i]["Height"].AsFloat;
m_dic[m_json[i]["Name"]] = data;
}
}
}
public class IntrData : BaseData
{
/// <summary>
/// 名字
/// </summary>
public string Name { set; get; }
/// <summary>
/// 高度
/// </summary>
public float Height { set; get; }
}
例子中IntrData继承的数据父类BaseData;管理者使用的是ReadInit的继承的函数,其中m_JsonFileName为json文件的文件名;后面的for循环,便是将解析的数据存入每个对象对应的字典中。
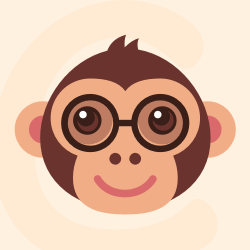



适用于现代 C++ 的 JSON。
最近提交(Master分支:1 个月前 )
960b763e
3 个月前
8c391e04
6 个月前
更多推荐
所有评论(0)