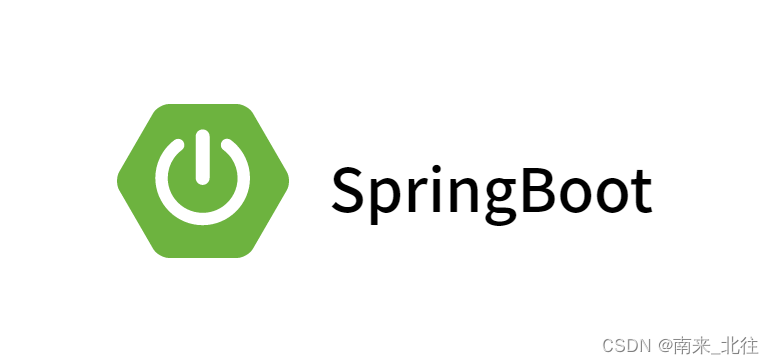
Spring Boot 中使用 JSON Schema 来校验复杂JSON数据

博客主页: 南来_北往
系列专栏:Spring Boot实战
前言
在应用程序接口(API)的开发中,JSON作为一种数据交换格式被广泛利用。然而,对数据的结构和规则进行标准化是至关重要的,这正是JSON Schema发挥作用的地方。JSON Schema通过一套精确的关键字,能够详细地定义数据的属性,包括类型、格式及其关系等。尽管如此,如果缺乏合适的工具来执行这些标准,JSON Schema本身无法确保数据实例严格遵循所设定的模式。因此,引入JSON Schema验证器成为确保数据一致性的关键步骤。这些验证器的功能就如同一个严格的审查过程,保证每个JSON文档都严格符合模式规范。作为实现JSON Schema规范的技术手段,验证器的集成灵活性使其适用于各种规模的项目,并能够无缝整合进开发流程中,从而显著提高数据处理的效率和准确性。
下面我们来看看如何在Spring Boot应用中使用JSON Schema校验JSON数据
实践
要在Spring Boot中使用JSON Schema来校验复杂JSON数据,你可以使用Everit JSON Schema
库。以下是如何在Spring Boot项目中集成和使用该库的步骤:
1、在你的pom.xml
文件中添加Everit JSON Schema
依赖:
<dependency>
<groupId>com.github.everit-org.json-schema</groupId>
<artifactId>org.everit.json.schema</artifactId>
<version>1.12.1</version>
</dependency>
2、建一个JSON Schema文件(例如user-schema.json
),用于描述你的JSON数据结构:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"age": {
"type": "integer",
"minimum": 0
},
"email": {
"type": "string",
"format": "email"
}
},
"required": ["name", "age", "email"]
}
3、在Spring Boot项目中,创建一个方法来加载JSON Schema文件并验证JSON数据:
import org.everit.json.schema.Schema;
import org.everit.json.schema.loader.SchemaLoader;
import org.json.JSONObject;
import org.json.JSONTokener;
import org.springframework.stereotype.Service;
import java.io.InputStream;
@Service
public class JsonValidationService {
public boolean validateJson(String jsonData) {
try {
// 加载JSON Schema文件
InputStream schemaStream = getClass().getResourceAsStream("/path/to/user-schema.json");
JSONObject rawSchema = new JSONObject(new JSONTokener(schemaStream));
Schema schema = SchemaLoader.load(rawSchema);
// 将JSON数据转换为JSONObject
JSONObject jsonObject = new JSONObject(jsonData);
// 验证JSON数据是否符合Schema
schema.validate(jsonObject);
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
}
4、在你的控制器或其他需要验证JSON数据的地方,调用validateJson
方法:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
@Autowired
private JsonValidationService jsonValidationService;
@PostMapping("/users")
public String createUser(@RequestBody String userJson) {
if (jsonValidationService.validateJson(userJson)) {
// 保存用户数据到数据库等操作
return "User created successfully";
} else {
return "Invalid user data";
}
}
}
这样,当你的应用程序接收到一个POST请求时,它会使用JSON Schema来验证请求体中的JSON数据是否符合预期的结构。如果验证失败,将返回一个错误消息。
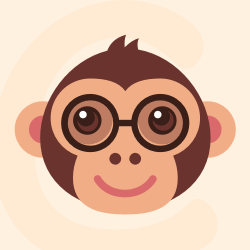



更多推荐
所有评论(0)