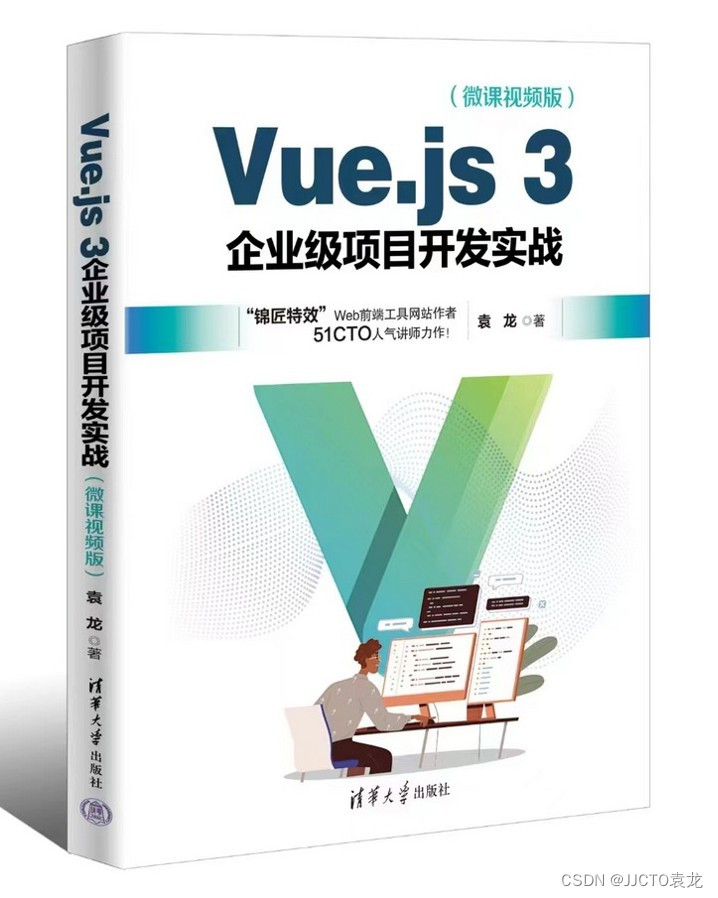
如何在Vue3中创建一个分页组件分割大量数据
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
在现代Web开发中,当数据量庞大时,分页已经成为一种基本需求。分页不仅能提高用户体验,还能有效减少性能瓶颈。作为一名前端开发者,你可能会在面试中被问到如何在 Vue 3 项目中实现分页。本文将详细讲解如何在 Vue 3 中创建一个分页组件,分割大量数据并优化用户体验。
思路概述
在创建一个分页组件时,我们需以下几个关键点:
- 数据分割:将大量数据分成适当大小的小段。
- 页码导航:提供跳转到特定页码的功能。
- 数据展示:仅展示当前页的数据。
- 用户交互:处理用户的页码点击、前进、后退等操作。
分步实现
第一步:项目初始化
首先,确保你的开发环境已有 Vue CLI,如果没有,可以通过以下命令安装:
npm install -g @vue/cli
接下来,通过 Vue CLI 创建一个新项目:
vue create pagination-demo
cd pagination-demo
npm run serve
第二步:创建分页组件
在 src/components
目录下创建一个名为 Pagination.vue
的文件,用于实现分页组件。
<template>
<div class="pagination">
<button @click="prevPage" :disabled="currentPage === 1">上一页</button>
<span v-for="page in totalPages" :key="page" @click="goToPage(page)"
:class="{'active': page === currentPage}">
{{ page }}
</span>
<button @click="nextPage" :disabled="currentPage === totalPages">下一</button>
</div>
</template>
<script>
export default {
props: {
totalItems: {
type: Number,
required: true
},
itemsPerPage: {
type: Number,
default: 10
}
},
data() {
return {
currentPage: 1
};
},
computed: {
totalPages() {
return Math.ceil(this.totalItems / this.itemsPerPage);
}
},
methods: {
goToPage(page) {
this.currentPage = page;
this.$emit('page-changed', this.currentPage);
},
prevPage() {
if (this.currentPage > 1) {
this.currentPage--;
this.$emit('page-changed', this.currentPage);
}
},
nextPage() {
if (this.currentPage < this.totalPages) {
this.currentPage++;
this.$emit('page-changed', this.currentPage);
}
}
}
};
</script>
<style scoped>
.pagination {
display: flex;
justify-content: center;
align-items: center;
}
.pagination span {
margin: 0 5px;
cursor: pointer;
}
.pagination span.active {
font-weight: bold;
}
.pagination button {
margin: 0 10px;
}
</style>
第三步:数据处理
在 src/views
目录下创建一个 HomeView.vue
文件,用于展示分页效果。在这个文件中将模拟大量数据及其分页处理。
<template>
<div>
<h1>分页示例</h1>
<ul>
<li v-for="item in paginatedData" :key="item.id">{{ item.name }}</li>
</ul>
<Pagination :totalItems="totalItems" @page-changed="handlePageChange" />
</div>
</template>
<script>
import Pagination from '@//Pagination.vue';
export default {
components: {
Pagination
},
data() {
return {
items: [],
paginatedData: [],
itemsPerPage 10,
totalItems: 0
};
},
methods: {
fetchData() {
// 模拟大量数据
this.items = Array.from({ length: 100 }, (v, i) => ({
id: i + 1,
name: `Item ${i + 1}`
}));
this.totalItems = this.items.length;
this.paginateData(1);
},
paginateData(page) {
const start = (page - 1) * this.itemsPage;
const end = page * this.itemsPerPage;
this.paginatedData = this.items.slice(start, end);
},
handlePageChange(page) {
this.paginateData(page);
}
},
created() {
this.fetchData();
}
};
</script>
<style scoped>
ul {
list-style-type: none;
padding: 0;
}
li {
margin: 5px 0;
}
</style>
第四步:路由配置(如果需要)
根据你的项目结构,你可能需要在 src/router/index.js
中添加相应的路由配置。
import { createRouter, createWebHistory } from 'vue-router';
import HomeView from '../views/HomeView.vue';
const routes = [
{
path: '/',
name: 'Home',
component: HomeView
}
];
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes
});
export default router;
第五步:运行项目并测试
确保一切都设置完毕后,启动项目:
npm run serve
打开浏览器,访问 http://localhost:8080
,你应该能看到一个简单的分页示例。
结论
通过本文,你学习了如何在 Vue 3 中创建一个基本的分页组件以及如何将其集成并到项目中。分页功能不仅能优化用户体验,还能显著提升应用的性能。
无论是在面试中还是在实际的项目开发中,熟练掌握分页组件的实现一种非常有用的技能。
最后问候亲爱的朋友们,并邀请你们阅读我的全新著作
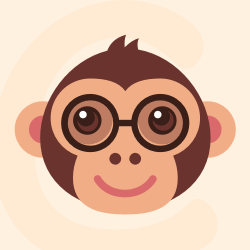



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:3 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 5 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 5 个月前
更多推荐
所有评论(0)