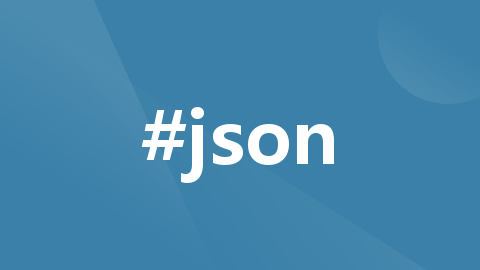
RestTemplate接口请求发送json、form数据格式以及处理接口错误状态码400 null
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
在使用RestTemplate
发送HTTP请求时,你可以通过不同的方式发送JSON或表单数据(application/x-www-form-urlencoded
)。同时,处理接口错误状态码(如400)和返回null
的情况也是很重要的。以下是一些示例代码,展示了如何使用RestTemplate
发送不同格式的数据,并处理错误。
发送JSON数据
要发送JSON数据,你需要将你的对象转换为JSON字符串,并设置正确的Content-Type
头为application/json
。你可以使用HttpEntity
和HttpHeaders
来构建请求,并使用RestTemplate
的postForObject
或postForEntity
方法发送请求。
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.web.client.RestTemplate;
// ...
RestTemplate restTemplate = new RestTemplate();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON);
// 假设你有一个名为MyObject的对象,你想将它发送为JSON
MyObject myObject = new MyObject();
// ... 设置myObject的属性
String jsonPayload = new ObjectMapper().writeValueAsString(myObject); // 使用Jackson库将对象转换为JSON字符串
HttpEntity<String> entity = new HttpEntity<>(jsonPayload, headers);
String url = "http://example.com/api/resource";
ResponseEntity<String> response = restTemplate.postForEntity(url, entity, String.class);
if (response.getStatusCode().is2xxSuccessful()) {
// 处理成功的响应
} else {
// 处理错误,例如状态码400
if (response.getStatusCode() == HttpStatus.BAD_REQUEST) {
// 错误处理逻辑,例如打印错误消息或记录日志
System.err.println("Bad request: " + response.getBody());
}
// 注意:如果响应体为null,response.getBody()将返回null
}
发送表单数据
要发送表单数据,你可以使用MultiValueMap
来存储表单字段和值,并使用formHttpMessageConverter
。
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.RestTemplate;
// ...
RestTemplate restTemplate = new RestTemplate();
MultiValueMap<String, String> map = new LinkedMultiValueMap<>();
map.add("key1", "value1");
map.add("key2", "value2");
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_FORM_URLENCODED);
HttpEntity<MultiValueMap<String, String>> request = new HttpEntity<>(map, headers);
String url = "http://example.com/api/resource";
ResponseEntity<String> response = restTemplate.postForEntity(url, request, String.class);
// 错误处理与上述相同
处理接口错误状态码400和null响应体
如上所示,你可以通过检查ResponseEntity
的getStatusCode
方法来处理不同的HTTP状态码。对于状态码400(Bad Request),你可以根据需要执行特定的错误处理逻辑。
如果响应体为null,response.getBody()
将返回null。在这种情况下,你可能需要根据你的业务逻辑来决定如何处理它。例如,你可以记录一个错误消息,或者抛出一个异常来指示调用者发生了问题。
请注意,上述示例使用了Jackson库来将对象转换为JSON字符串。如果你的项目中还没有包含Jackson,你需要在你的pom.xml
或build.gradle
中添加相应的依赖项。
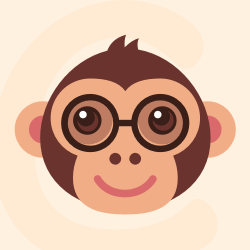



适用于现代 C++ 的 JSON。
最近提交(Master分支:1 个月前 )
960b763e
4 个月前
8c391e04
6 个月前
更多推荐
所有评论(0)