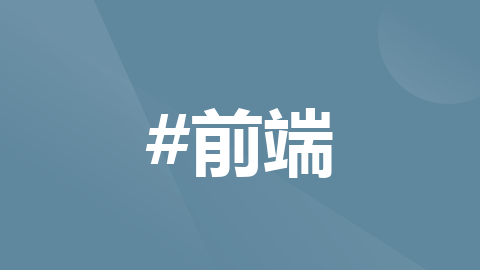
vue+elementUI实现在表格中添加输入框并校验的功能
element
A Vue.js 2.0 UI Toolkit for Web
项目地址:https://gitcode.com/gh_mirrors/eleme/element

·
背景:
vue2+elmui
需求:
需要在一个table中添加若干个输入框,并且在提交时需要添加校验
思路:
- 当需要校验的时候可以考虑添加form表单来触发校验,因此需要在table外面套一层form表单,表单的属性就是ref,model,rules三件套,ref来获取组件实例validate从而进行校验,model为绑定的数据,rules为对应规则。
- 在每一个input外面套一层form-item,并且添加对应的校验规则
- 通过计算属性提炼出需要绑定的数据
- 设置校验函数,在进行提交时调用该方法,获取这个方法的返回值,若是false则return,否则进行后续的代码逻辑
实现代码:
<el-form ref="formRef" :model="validateForm" :rules="rules">
<el-table :data="validateForm.tableData" border height="300">
<el-table-column label="安全最低温" property="tempMin">
<template slot-scope="scope">
<el-form-item :prop="'tableData.' + scope.$index + '.tempMin'" :rules="rules.tempMin">
<el-input v-model="scope.row.tempMin" placeholder="请填写安全最低温" size="small" />
</el-form-item>
</template>
</el-table-column>
<el-table-column label="安全最高温" property="tempMax">
<template slot-scope="scope">
<el-form-item :prop="'tableData.' + scope.$index + '.tempMax'" :rules="rules.tempMax">
<el-input v-model="scope.row.tempMax" placeholder="请填写安全最高温" size="small" />
</el-form-item>
</template>
</el-table-column>
<el-table-column label="温度预警区间" property="tempWarningVal">
<template slot-scope="scope">
<el-form-item :prop="'tableData.' + scope.$index + '.tempWarningVal'" :rules="rules.tempWarningVal">
<el-input v-model="scope.row.tempWarningVal" placeholder="请填写预警" size="small" />
</el-form-item>
</template>
</el-table-column>
<el-table-column label="安全湿度上限" property="humidityMax">
<template slot-scope="scope">
<el-form-item :prop="'tableData.' + scope.$index + '.humidityMax'" :rules="rules.humidityMax">
<el-input v-model="scope.row.humidityMax" placeholder="请填写安全最低温" size="small" />
</el-form-item>
</template>
</el-table-column>
<el-table-column label="安全湿度下限" property="humidityMin">
<template slot-scope="scope">
<el-form-item :prop="'tableData.' + scope.$index + '.humidityMin'" :rules="rules.humidityMin">
<el-input v-model="scope.row.humidityMin" placeholder="请填写安全最高温" size="small" />
</el-form-item>
</template>
</el-table-column>
<el-table-column label="湿度预警区间" property="humidityWarningVal">
<template slot-scope="scope">
<el-form-item :prop="'tableData.' + scope.$index + '.humidityWarningVal'" :rules="rules.humidityWarningVal">
<el-input v-model="scope.row.humidityWarningVal" placeholder="请填写安全最低温" size="small" />
</el-form-item>
</template>
</el-table-column>
</el-table>
</el-form>
computed: {
// 通过计算属性来得出table绑定的数据
validateForm() {
const validateForm = {}
const dataArr = this.gridData.map((item, index) => {
item.index = index
return item
})
const tempList = dataArr.filter(item => item.outSiteCode === this.outSiteSelect)
validateForm.tableData = tempList
return validateForm
}
},
rules: {
// 设置校验规则
tempMin: [
{ required: true, message: '', trigger: ['blur', 'change'] }
],
tempMax: [
{ required: true, message: '', trigger: ['blur', 'change'] }
],
tempWarningVal: [
{ required: true, message: '', trigger: ['blur', 'change'] }
],
humidityMax: [
{ required: true, message: '', trigger: ['blur', 'change'] }
],
humidityMin: [
{ required: true, message: '', trigger: ['blur', 'change'] }
],
humidityWarningVal: [
{ required: true, message: '', trigger: ['blur', 'change'] }
]
},
/*校验函数,通过设置flag来校验每一项,如果没填写就是false,并且返回*/
validateTable() {
let flag = false
this.$refs.formRef.validate((valid) => {
if (!valid) flag = false
else { flag = true }
})
return flag
},
/*提交函数,提交表单前,进行校验,没通过就return*/
handleFormChange() {
const validateFlag = this.validateTable()
if (!validateFlag) return
// ...后续的提交表单逻辑
}
最终效果:
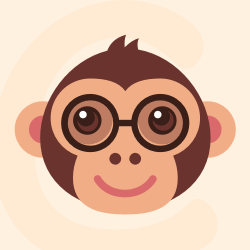



A Vue.js 2.0 UI Toolkit for Web
最近提交(Master分支:3 个月前 )
c345bb45
7 个月前
a07f3a59
* Update transition.md
* Update table.md
* Update transition.md
* Update table.md
* Update transition.md
* Update table.md
* Update table.md
* Update transition.md
* Update popover.md 7 个月前
更多推荐
所有评论(0)