Vue性能优化之——引用CDN,提高首屏加载速度
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
Vue 项目*打包后的 vendor.js 过大,将导致打包速度慢,首屏加载慢 *。其原因是第三方库比如(vue、vue-router、vuex、axios等)都会被打包到 vendor.js 文件里面,而浏览器是在加载该文件之后,才开始显示首屏的。
CDN优化是指,把第三方库比如(vue、vue-router、vuex、axios等)通过cdn的方式引入到项目中。这样浏览器可以开启多个线程,将vendor.js、外部js、css等加载下来(bootcdn等资源,或其他服务器资源),使得vendor.js文件减少,从而提升首屏加载速度。
1…vue.config.js中配置如下:
const path = require('path');
const resolve = (dir) => path.resolve(__dirname, dir);
// 开启gzip压缩,先下载 npm i -D compression-webpack-plugin@5.0.0
const CompressionWebpackPlugin = require("compression-webpack-plugin");
// 是否是生产环境
const isProduction = process.env.NODE_ENV === 'production';
// 是否需要使用cdn
const isNeedCdn = false
// 是否需要进行 资源树 分析。安装 npm i -D webpack-bundle-analyzer
const isNeedAnalyzer = false
const assetsCDN = {
/**
externals对象的属性名为package.json中,对应的库的名称(固定写法),属性值为引入时你自定义的名称
*/
externals: {
vue: 'Vue',
'vue-router': 'VueRouter',
vuex: 'Vuex',
axios: 'axios'
},
css: [],
js: [
'https://cdn.bootcdn.net/ajax/libs/vue/3.2.0/vue.global.min.js',
'https://cdn.bootcdn.net/ajax/libs/vuex/4.0.2/vuex.global.min.js',
'https://cdn.bootcdn.net/ajax/libs/vue-router/4.0.14/vue-router.global.min.js',
'https://cdn.bootcdn.net/ajax/libs/axios/0.25.0/axios.min.js'
]
}
module.exports = {
lintOnSave: false,
productionSourceMap: false,
assetsDir: 'assets',
publicPath: isProduction ? '/subroot' : '/', // subroot 项目部署在 子目录subroot下
devServer: {
proxy: {
'/api': {
target: serve,
changeOrigin: true,
pathRewrite: {
'^/api': ''
}
}
}
},
css: {
extract: false, // 不使用css分离插件
loaderOptions: {
css: {},
postcss: {
plugins: [
require('postcss-px2rem')({ // 自适应
// 以设计稿750为例, 750 / 10 = 75
remUnit: 37.5
}),
]
}
},
},
configureWebpack: (config) => {
// 生产环境,则添加不参与打包的包名和依赖包的名称
if (isProduction || isNeedCDN) {
config.externals = assetsCDN.externals;
}
if (isProduction) {
config.plugins.push(
new CompressionWebpackPlugin({
filename: "[path].gz[query]",
algorithm: "gzip",
test: /\.(js|css)(\?.*)?$/i,
threshold: 10240, //只对10K以上进行压缩
minRatio: 0.8,
})
);
}
},
chainWebpack: (config) => {
// 优化首屏加载速度--移除 preload、prefetch 插件
config.plugins.delete('preload');
config.plugins.delete('prefetch');
// 生产环境或需要cdn时,才注入cdn
if (isProduction || isNeedCdn) {
config.plugin('html').tap(args => {
args[0].cdn = assetsCDN
return args
})
}
// 资源树分析
if (isNeedAnalyzer) {
config.plugin('webpack-bundle-analyzer').use(require('webpack-bundle-analyzer').BundleAnalyzerPlugin).end()
}
// splitChunks抽离公共文件,避免重复请求
config.when(isProductions, (config) => {
config.optimization.splitChunks({
chunks: 'all',
cacheGroups: {
libs: {
name: 'chunk-libs',
test: /[\\/]node_modules[\\/]/,
priority: 10,
chunks: 'initial' },
commons: {
name: 'chunk-commons',
test: resolve('src/components'),
minChunks: 3,
priority: 5,
reuseExistingChunk: true // 是否使用已有的 chunk,如果为 true, 则表示如果当前的 chunk 包含的模块已经被抽取出去了,那么将不会重新生成新的。
}
}
})
// https:// webpack.js.org/configuration/optimization/#optimizationruntimechunk
config.optimization.runtimeChunk('single')
})
}
}
2.在public/index.html中引入资源js、css
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width,initial-scale=1.0,minimum-scale=1.0,maximum-scale=1.0,user-scalable=no,viewport-fit=cover">
<meta http-equiv="Cache-Control" content="no-cache, no-store, must-revalidate" />
<meta http-equiv="Pragma" content="no-cache" />
<meta http-equiv="Expires" content="0" />
<meta http-equiv="Cache" content="no-cache">
<title>引用CDN静态资源</title>
<style>
body {
width: 100vw;
height: 100vh;
}
</style>
<!-- 使用CDN的CSS文件 -->
<% for (var i in htmlWebpackPlugin.options.cdn && htmlWebpackPlugin.options.cdn.css) { %>
<link rel="stylesheet" href="<%= htmlWebpackPlugin.options.cdn.css[i] %>" />
<% } %>
</head>
<body>
<div id="app"></div>
<!-- 使用CDN的JS文件 -->
<% for (var i in htmlWebpackPlugin.options.cdn && htmlWebpackPlugin.options.cdn.js) { %>
<script src="<%= htmlWebpackPlugin.options.cdn.js[i] %>"></script>
<% } %>
</body>
</html>
3.验证
打包部署到服务器上,打开开发者工具 network,如果看到请求的资源中有自己引入的cdn链接,则配置成功。
或者在console打印台输入this 可以看到
需注意的是:CDN虽然可以提供首屏加载速度,但没有本地打包稳定
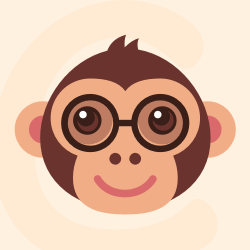



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:1 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 3 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 3 个月前
更多推荐
所有评论(0)