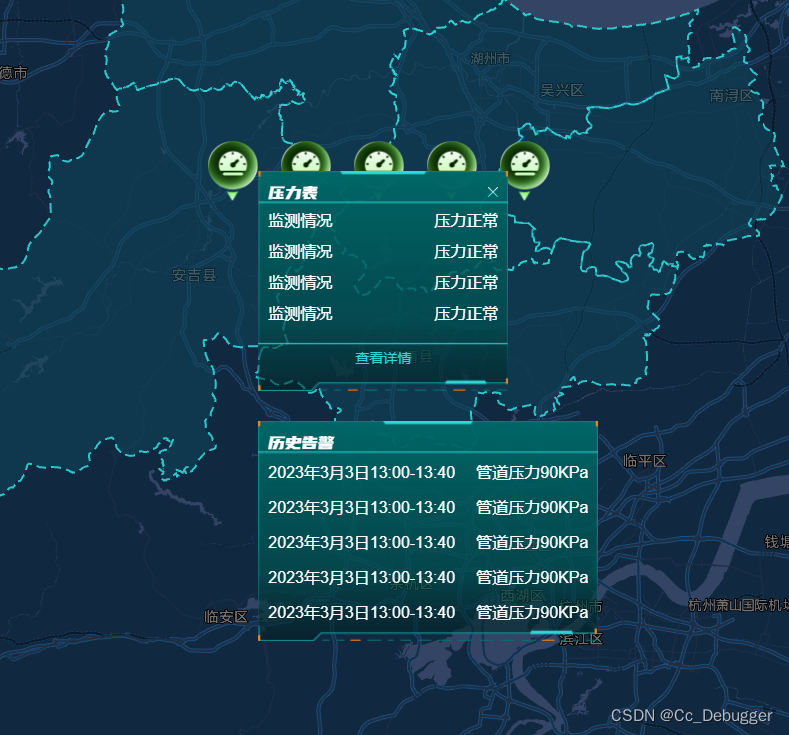
vue3/高德地图实现点击自定义点位/infoWindow自定义弹窗
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
<template>
<div class="app-container">
<div style="background-color: #ffffff;">
<div id="container"></div>
</div>
<div ref="dialog" style="position: fixed;">
<div class="dialog">
<div class="dialog-title"><span>压力表</span> <img @click="closeInfoWindow" src="../assets/images/删除 (17).png"
alt="" srcset="">
</div>
<div class="dialog-item"><span>监测情况</span> <span>压力正常</span></div>
<div class="dialog-item"><span>监测情况</span> <span>压力正常</span></div>
<div class="dialog-item"><span>监测情况</span> <span>压力正常</span></div>
<div class="dialog-item"><span>监测情况</span> <span>压力正常</span></div>
<div class="detail">查看详情</div>
</div>
<div class="dialog1">
<div class="dialog-title">历史告警</div>
<div class="dialog-item"><span>2023年3月3日13:00-13:40 </span> <span> 管道压力90KPa</span></div>
<div class="dialog-item"><span>2023年3月3日13:00-13:40 </span> <span> 管道压力90KPa</span></div>
<div class="dialog-item"><span>2023年3月3日13:00-13:40 </span> <span> 管道压力90KPa</span></div>
<div class="dialog-item"><span>2023年3月3日13:00-13:40 </span> <span> 管道压力90KPa</span></div>
<div class="dialog-item"><span>2023年3月3日13:00-13:40 </span> <span> 管道压力90KPa</span></div>
</div>
</div>
</div>
</template>
<script setup>
import AMapLoader from '@amap/amap-jsapi-loader';
/*在Vue3中使用时,需要引入Vue3中的shallowRef方法(使用shallowRef进行非深度监听,
因为在Vue3中所使用的Proxy拦截操作会改变JSAPI原生对象,所以此处需要区别Vue2使用方式对地图对象进行非深度监听,
否则会出现问题,建议JSAPI相关对象采用非响应式的普通对象来存储)*/
import { shallowRef } from '@vue/reactivity';
import { ref, reactive, getCurrentInstance } from "vue";
const { proxy } = getCurrentInstance();
const allMap = shallowRef(null);
const allInfoWindow = shallowRef(null);
function initMap() {
window._AMapSecurityConfig = {
securityJsCode: '',
}
AMapLoader.load({
key: "", // 申请好的Web端开发者Key,首次调用 load 时必填
version: "2.0", // 指定要加载的 JSAPI 的版本,缺省时默认为 1.4.15
plugins: ["AMap.GeoJSON"], // 需要使用的的插件列表,如比例尺'AMap.Scale'等
}).then((AMap) => {
const map = new AMap.Map("container", { //设置地图容器id
viewMode: "2D", //是否为3D地图模式
zoom: 10, //初始化地图级别
center: [119.898881, 30.594178], //初始化地图中心点位置
mapStyle: 'amap://styles/',
});
allMap.value = map
const markerdata = reactive([[119.898881, 30.794178], [119.998881, 30.794178], [119.798881, 30.794178], [119.698881, 30.794178], [120.098881, 30.794178]])
markerdata.forEach(element => {
// 创建 AMap.Icon 实例:
const icon = new AMap.Icon({
size: new AMap.Size(50, 60), // 图标尺寸
image: new URL('../assets/images/压力表.png', import.meta.url).href, // Icon的图像
// imageOffset: new AMap.Pixel(0, -60), // 图像相对展示区域的偏移量,适于雪碧图等
imageSize: new AMap.Size(50, 60), // 根据所设置的大小拉伸或压缩图片
imageOffset: new AMap.Pixel(0, 0)
});
// 创建一个 Marker 实例:
const marker = new AMap.Marker({
position: new AMap.LngLat(element[0], element[1]), // 经纬度对象,也可以是经纬度构成的一维数组[116.39, 39.9]
// title: 'hhhhhh',
icon: icon
});
marker.content = proxy.$refs.dialog
// 将创建的点标记添加到已有的地图实例:
map.add(marker);
//信息窗口实例
const infoWindow = new AMap.InfoWindow({
anchor: "top-left",
//
offset: new AMap.Pixel(50, 30),
//隐藏原生弹窗边框和关闭按钮
isCustom: true,
autoMove: true,
avoid: [20, 20, 20, 20],
// 点击地图关闭
closeWhenClickMap: true,
});
allInfoWindow.value = infoWindow
const markerClick = (e) => {
console.log(e, 'e');
// 标注的点击事件
infoWindow.setContent(e.target.content);
infoWindow.open(map, e.target.getPosition());
}
marker.on('click', markerClick);
});
}).catch(e => {
console.log(e);
})
}
initMap()
const closeInfoWindow = () => {
console.log(allInfoWindow, 'allInfoWindow');
// 关闭信息窗体
allMap.value.clearInfoWindow()
}
const seeDetail = () => {
console.log(1, 'see');
}
</script>
<style lang="scss" scoped>
.app-container {
position: absolute;
top: 0;
left: 0;
z-index: -1;
}
#container {
padding: 0px;
margin: 0px;
width: 1920px;
height: 1080px;
}
.dialog {
width: 230px;
height: 200px;
background-image: url('../assets/images/弹窗1.png');
background-size: 100% 100%;
background-repeat: no-repeat;
padding: 10px;
color: #fff;
display: flex;
flex-direction: column;
.dialog-title {
font-size: 18px;
font-family: 优设标题黑;
font-weight: 400;
text-align: left;
display: flex;
align-items: center;
justify-content: space-between;
img {
width: 10px;
height: 10px;
}
}
.dialog-item {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
margin: 5px 0;
}
.detail {
font-size: 14px;
font-family: Microsoft YaHei-Regular, Microsoft YaHei;
font-weight: 400;
color: #2FD7D7;
line-height: 60px;
text-align: center;
cursor: pointer;
}
}
.dialog1 {
width: 320px;
height: 200px;
background-image: url('../assets/images/弹窗2.png');
background-size: 100% 100%;
background-repeat: no-repeat;
padding: 10px;
color: #fff;
display: flex;
flex-direction: column;
margin-top: 30px;
.dialog-title {
font-size: 18px;
font-family: 优设标题黑;
font-weight: 400;
text-align: left;
}
.dialog-item {
display: flex;
justify-content: space-between;
flex-wrap: wrap;
margin: 7px 0;
}
}
</style>
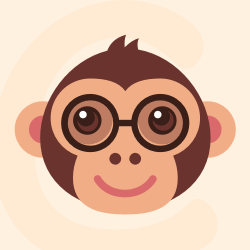



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:2 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 4 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 5 个月前
更多推荐
所有评论(0)