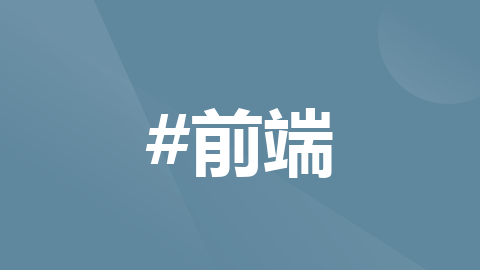
Vue使用PDFJS实现前端水印(印章)
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
const getJavsScript = (src) => {
return new Promise((resolve, reject) => {
const script = document.createElement('script')
script.onload = resolve
script.onerror = reject
script.src = src
document.body.append(script)
})
}
const getCss = (href) => {
return new Promise((resolve, reject) => {
const link = document.createElement('link')
link.onload = resolve
link.onerror = reject
link.setAttribute('rel', 'stylesheet')
link.setAttribute('type', 'text/css')
link.href=href
document.body.append(link)
})
}
const getPdfjsDist = (pdfjsDistPath) => {
return getJavsScript(`${pdfjsDistPath}/pdf/build/pdf.js`)
.then(() => {
return Promise.all([
getJavsScript(`${pdfjsDistPath}/pdf/web/pdf_viewer.js`),
getCss(`${pdfjsDistPath}/pdf/web/pdf_viewer.css`)
])
})
}
export default getPdfjsDist
import getPdfjsDist from './getPdfjsDist'
export default {
name: 'Pdf',
props: {
url: {
type: String,
default: ''
},
type: {
type: String,
default: 'canvas'
},
pdfjsDistPath: {
type: String,
default: '.'
}
},
data() {
return {
pdfViewer: null,
pdfLinkService: null,
currentScale: '1.0',
loadingTask: null
}
},
methods: {
onPagesInit({ source }) {
source.currentScaleValue = this.currentScale
},
async pdfLibInit() {
let pdfjsLib = window.pdfjsLib;
let pdfjsViewer = window.pdfjsViewer
if (!pdfjsLib || !pdfjsViewer) {
try {
await getPdfjsDist(this.pdfjsDistPath)
this.CMAP_URL = `${this.pdfjsDistPath}/pdf/cmaps/`;
pdfjsLib = window.pdfjsLib;
pdfjsLib.GlobalWorkerOptions.workerSrc = `${this.pdfjsDistPath}/pdf/build/pdf.worker.js`
pdfjsViewer = window.pdfjsViewer
} catch (error) {
return
}
}
const container = this.$refs.container
const eventBus = new pdfjsViewer.EventBus();
const pdfLinkService = new pdfjsViewer.PDFLinkService({
eventBus: eventBus,
});
this.pdfLinkService = pdfLinkService
const pdfViewer = new pdfjsViewer.PDFViewer({
container: container,
eventBus: eventBus,
linkService: pdfLinkService,
renderer: this.type,
textLayerMode: 0,
downloadManager: new pdfjsViewer.DownloadManager({}),
enableWebGL: true
});
this.pdfViewer = pdfViewer
pdfLinkService.setViewer(pdfViewer);
eventBus.on("pagesinit", this.onPagesInit);
},
renderPdf() {
if (!this.url) { return }
if (this.pdfViewer === null || this.pdfLinkService === null) {
return
}
if (this.loadingTask !== null) {
this.loadingTask.destroy()
this.loadingTask = null
}
this.loadingTask = window.pdfjsLib.getDocument({
cMapUrl: this.CMAP_URL,
cMapPacked: true,
url: this.url,
});
return this.loadingTask.promise.then((pdfDocument) => {
if (pdfDocument.loadingTask.destroyed || !this.url) { return }
this.pdfViewer.setDocument(pdfDocument)
this.pdfLinkService.setDocument(pdfDocument, null);
this.loadingTask = null
}).catch(error => {
console.log(error)
});
}
},
mounted() {
this.pdfLibInit().then(() => {
this.renderPdf()
})
},
watch: {
url() {
if (this.pdfViewer) {
this.pdfViewer._cancelRendering()
}
this.renderPdf()
}
},
render() {
return (
<div class="pdf-view">
<div id="viewerContainer" ref="container">
<div id="viewer" class="pdfViewer"/>
</div>
</div>
)
}
}
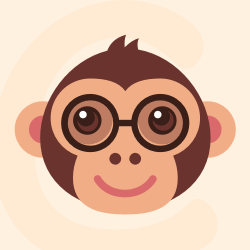



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:2 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 4 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 5 个月前
更多推荐
所有评论(0)