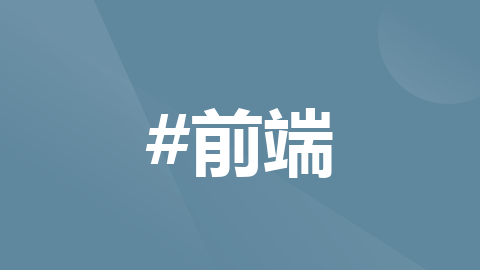
vue3高德地图API结合Echarts实现地图下钻功能
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
首先安装amap-jsapi-loader/echarts
npm i amap-jsapi-loader --save
npm i echarts
导入依赖
import * as echarts from "echarts";
import AMapLoader from '@amap/amap-jsapi-loader'
创建AMapKey,AMapKey是申请高德地图账号后,配置引用的key
你可以使用AmapLoader从高德地图加载API,然后在地图准备好后使用AmapMap组件进行展示
// 历史记录
const history = ref([
{
title: "全国",
adcode: 100000,
},
])
onMounted(async () => {
getJson(100000);
})
// 地图数据
const getJson = (val) => {
var MapJsons = [];
var MapJson = [];
//去拿地图json数据
getGeoJson(val).then((res) => {
// console.log(res)
//模拟的假数据
MapJsons = res.features?.map((item) => {
if (item.properties.name == '海南省') {
console.log(item, 'item')
// 隐藏海南省下面的地图部分
item.geometry.coordinates = coordinates
}
return {
adcode: item.properties.adcode,
name: item.properties.name,
value: findValue(item.properties.name),
level: item.properties.level
};
});
MapJson = MapJsons?.sort(function (a, b) {
return a.value - b.value;
});
//模拟的假数据
//调用绘制地图方法
drawMap(res, MapJson);
console.log(history.value, '54')
});
};
// echarts
const drawMap = (map, json) => {
//拿到标签的dom
var mapChart = echarts.init(document.getElementById("map"));
echarts.registerMap("SC", map); //注册地图
//配置项
let mapOption = {
tooltip: {
trigger: "item",
formatter: (p) => {
let val = p.value;
if (p.name == "南海诸岛") return;
if (window.isNaN(val)) {
val = 0;
}
let txtCon =
"<div style='text-align:left'>" +
// p.name
// +
"图表展示title:" +
val + "(个)" +
"</div>";
return txtCon;
},
},
visualMap: {
show: false, // 控制滑动条
min: 0,
max: 30,
right: "0%",
bottom: "0%",
calculable: true,
inRange: {
color: ['#1E62AC', "#fc9700", '#ffde00', '#fc9700', '#00eaff'],
// color: ['#dddddd', '#fc9700', '#ffde00', '#ffde00', '#00eaff']
},
textStyle: {
color: "#24CFF4",
},
},
series: [
{
name: "SC",
type: "map",
map: "SC",
zoom: 1.2, //当前视角的缩放比例
// center: [105.191, 36.582], // 地图中心
roam: true, //是否开启平游或缩放
// scaleLimit: {
// //滚轮缩放的极限控制
// min: 1,
// max: 20,
// },
label: {
normal: {
show: true,
color: "rgb(249, 249, 249)", //省份标签字体颜色
},
emphasis: {
show: true,
color: "#f75a00", //鼠标放上去字体颜色
},
},
itemStyle: {
//省突起来的效果
normal: {
areaColor: "#24CFF4",
borderColor: "#53D9FF",
borderWidth: 1,
shadowBlur: 15,
shadowColor: "rgb(58,115,192)",
shadowOffsetX: 0,
shadowOffsetY: 0,
},
emphasis: {
areaColor: "#8dd7fc",
borderWidth: 1.6,
shadowBlur: 25,
},
},
data: json,
},
],
};
window.addEventListener("resize", () => {
mapChart.resize();
});
//加载进去,后面的true为了重新绘制
mapChart.setOption(mapOption, true);
//点击事件
mapChart.on("click", (params) => {
// console.log(params)
if (history.value[history.value.length - 1].adcode == params.data.adcode) {
} else {
if (params.data.level == 'district') {
return false
}
index.value++
let obj = {
title: params.data.name,
adcode: params.data.adcode,
level: params.data.level
};
console.log(params.data.level)
if (params.data.level == 'province') {
req.province = params.data.name
getOnhand()
} else if (params.data.level == 'city') {
req.province = null
req.city = params.data.name
getOnhand()
}
//存储点击下钻的数据,方便回到上级
history.value.push(obj);
//调用获取json
getJson(params.data.adcode);
}
})
// console.log(history.value)
// 右键返回
// mapChart.on("contextmenu", params => {
// console.log(params, history.value)
// if (history.value.length - 1 > 0) {
// history.value.splice(-1)
// let code = history.value[history.value.length - 1].adcode
// console.log(code)
// getJson(code)
// // params.event.preventDefault();
// }
// });
};
const getGeoJson = (adcode, geoJson = []) => {
return new Promise((resolve, reject) => {
AMapLoader.load({
key: '申请好的Web端开发者Key', // 申请好的Web端开发者Key,首次调用 load 时必填
// version: '1.4.4',// 指定要加载的 JSAPI 的版本,缺省时默认为 1.4.15
AMapUI: {
// version: '1.1', // AMapUI 缺省 1.1
plugins: [], // 需要加载的 AMapUI ui插件
},
}).then(() => {
new AMapUI.loadUI(['geo/DistrictExplorer'], DistrictExplorer => {
var districtExplorer = new DistrictExplorer();
districtExplorer.loadAreaNode(adcode, function (error, areaNode) {
if (error) {
console.error(error);
reject(error, 'error 191');
return;
}
let Json = areaNode.getSubFeatures();
let mapJson = {
features: [],
};
if (Json.length === 0) {
Json = geoJson.features.filter(
(item) => item.properties.adcode == adcode
);
}
mapJson.features = Json;
resolve(mapJson);
})
})
}).catch(err => {
console.log(err)
})
})
}
let coordinates = [
[
[
[110.106396, 20.026812],
[110.042339, 19.991384],
[109.997375, 19.980136],
[109.965346, 19.993634],
[109.898825, 19.994196],
[109.855093, 19.984073],
[109.814441, 19.993072],
[109.76147, 19.981261],
[109.712195, 20.017253],
[109.657993, 20.01163],
[109.585312, 19.98801],
[109.526797, 19.943573],
[109.498464, 19.873236],
[109.411001, 19.895184],
[109.349407, 19.898561],
[109.300748, 19.917693],
[109.25948, 19.898561],
[109.255784, 19.867045],
[109.231147, 19.863105],
[109.159082, 19.79048],
[109.169553, 19.736411],
[109.147379, 19.704863],
[109.093792, 19.68965],
[109.048829, 19.619764],
[108.993394, 19.587065],
[108.92872, 19.524468],
[108.855424, 19.469182],
[108.806148, 19.450561],
[108.765496, 19.400894],
[108.694047, 19.387346],
[108.644772, 19.349518],
[108.609048, 19.276661],
[108.591186, 19.141592],
[108.598577, 19.055633],
[108.630606, 19.003017],
[108.637997, 18.924346],
[108.595497, 18.872256],
[108.593033, 18.809386],
[108.65278, 18.740258],
[108.663866, 18.67337],
[108.641077, 18.565614],
[108.644772, 18.486738],
[108.68912, 18.447571],
[108.776583, 18.441894],
[108.881293, 18.416344],
[108.905315, 18.389087],
[108.944735, 18.314107],
[109.006329, 18.323198],
[109.108575, 18.323766],
[109.138756, 18.268081],
[109.17448, 18.260125],
[109.287813, 18.264671],
[109.355566, 18.215221],
[109.441182, 18.199303],
[109.467051, 18.173718],
[109.527413, 18.169169],
[109.584696, 18.143579],
[109.661688, 18.175424],
[109.726362, 18.177698],
[109.749767, 18.193618],
[109.785492, 18.339672],
[109.919767, 18.375457],
[110.022629, 18.360121],
[110.070672, 18.376025],
[110.090382, 18.399309],
[110.116867, 18.506602],
[110.214186, 18.578662],
[110.246215, 18.609859],
[110.329366, 18.642185],
[110.367555, 18.631977],
[110.499366, 18.651824],
[110.499366, 18.751592],
[110.578206, 18.784458],
[110.590525, 18.838841],
[110.585597, 18.88075],
[110.619474, 19.152334],
[110.676756, 19.286264],
[110.706321, 19.320153],
[110.729727, 19.378878],
[110.787009, 19.399765],
[110.844292, 19.449996],
[110.888023, 19.518827],
[110.920668, 19.552668],
[111.008747, 19.60398],
[111.061718, 19.612436],
[111.071573, 19.628784],
[111.043856, 19.763448],
[111.013675, 19.850159],
[110.966248, 20.018377],
[110.940994, 20.028499],
[110.871393, 20.01163],
[110.808567, 20.035808],
[110.778386, 20.068415],
[110.744509, 20.074036],
[110.717408, 20.148778],
[110.687843, 20.163947],
[110.655814, 20.134169],
[110.562191, 20.110006],
[110.526467, 20.07516],
[110.495054, 20.077408],
[110.387265, 20.113378],
[110.318279, 20.108882],
[110.28933, 20.056047],
[110.243135, 20.077408],
[110.144585, 20.074598],
[110.106396, 20.026812]
]
]
]
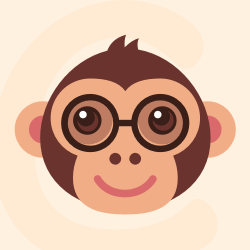



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:2 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 4 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 4 个月前
更多推荐
所有评论(0)