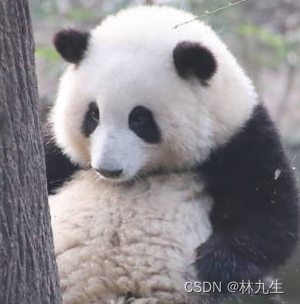
【Python】OpenCV-使用ResNet50进行图像分类
opencv
OpenCV: 开源计算机视觉库
项目地址:https://gitcode.com/gh_mirrors/opencv31/opencv

·
使用ResNet50进行图像分类
如何使用ResNet50模型对图像进行分类。
import os
import cv2
import numpy as np
from tensorflow.keras.applications.resnet50 import ResNet50, preprocess_input, decode_predictions
from tensorflow.keras.preprocessing import image
# 设置代理
os.environ["HTTP_PROXY"] = "http://127.0.0.1:1080"
os.environ["HTTPS_PROXY"] = "http://127.0.0.1:1080"
# 加载ResNet50模型
model = ResNet50(weights='imagenet')
# 读取和预处理图像
def preprocess_image(img_path):
# 加载图像并调整大小为(224, 224)
img = image.load_img(img_path, target_size=(224, 224))
# 将图像转换为numpy数组
img_array = image.img_to_array(img)
# 在第0轴上添加维度,将其变为(1, 224, 224, 3)
img_array = np.expand_dims(img_array, axis=0)
# 对图像进行预处理,以适应ResNet50模型的输入要求
img_array = preprocess_input(img_array)
return img_array
# 加载图像
img_path = 'pandas.jpg'
img = preprocess_image(img_path)
# 进行预测
predictions = model.predict(img)
# 解码预测结果,获取前三个预测结果
decoded_predictions = decode_predictions(predictions, top=3)[0]
# 打印结果
print("Predictions:")
for i, (imagenet_id, label, score) in enumerate(decoded_predictions):
print(f"{i + 1}: {label} ({score:.2f})")
# 显示图像
img = cv2.imread(img_path)
cv2.imshow('Image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
-
测试图片:
-
运行效果:
-
翻译一下
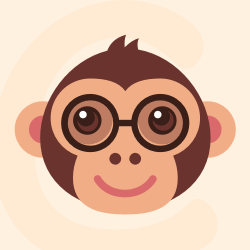



OpenCV: 开源计算机视觉库
最近提交(Master分支:1 个月前 )
08f7f13d
Move the gcc6 compatibility check to occur on a per-directory basis, … #26234
Proposed fix for #26233 https://github.com/opencv/opencv/issues/26233
### Pull Request Readiness Checklist
See details at https://github.com/opencv/opencv/wiki/How_to_contribute#making-a-good-pull-request
- [x] I agree to contribute to the project under Apache 2 License.
- [x] To the best of my knowledge, the proposed patch is not based on a code under GPL or another license that is incompatible with OpenCV
- [x] The PR is proposed to the proper branch
- [x] There is a reference to the original bug report and related work
- [ ] There is accuracy test, performance test and test data in opencv_extra repository, if applicable
Patch to opencv_extra has the same branch name.
- [ ] The feature is well documented and sample code can be built with the project CMake
2 天前
0f234209
Added buffer-based model loading to FaceRecognizerSF 3 天前
更多推荐
所有评论(0)