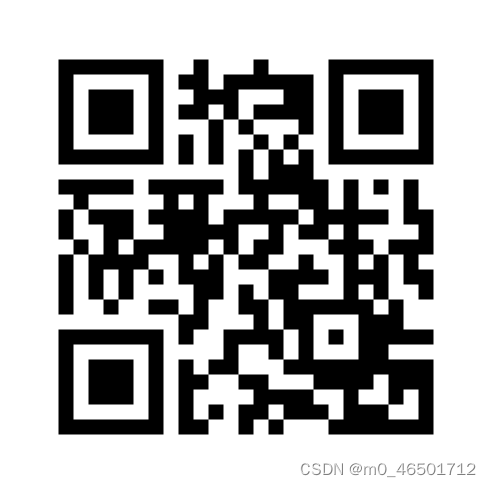
python+opencv-二维码和datamartix码读取
opencv
OpenCV: 开源计算机视觉库
项目地址:https://gitcode.com/gh_mirrors/opencv31/opencv

·
python+opencv-二维码和datamartix码读取
1、二维码读取
1.1实现方法:
采用现有的二维码识别库对图片进行识别,网上有许多传统的和深度学习的二维码读取库,本次就是通过现有的pyzbar库进行二维码识别,该库为比较一系列二维码识别库得出来的效果较好的库。
from pyzbar.pyzbar import decode
1.2实现步骤:
1)读取图片(不是灰度图的需要转化为灰度图);
2)高斯滤波,去除噪音干扰;
3)二值化,突出二维码黑白颜色区分;
4)调用pyzbar.decode库进行识别;
5)返回识别的信息,常为四个角点的坐标和二维码的信息。
1.3实现代码:
#!/usr/bin/python3
# -*- coding: utf-8 -*-
import cv2
import numpy as np
from pyzbar.pyzbar import decode
from PIL import Image, ImageDraw
import time
src = cv2.imread("5.bmp")
print(src.shape)
# 灰度转换
gray = cv2.cvtColor(src, cv2.COLOR_BGR2GRAY)
text2 = cv2.medianBlur(gray,3)
ret,text2 = cv2.threshold(text2, 180, 255, cv2.THRESH_BINARY)
for code in decode(text2):
data = code.data.decode('utf-8')
print("条形码/二维码数据:", data) #解码数据
rect = code.rect
print(rect)
cv2.putText(src, data, (300,500 ), cv2.FONT_HERSHEY_SIMPLEX, 5, (0, 0,255), 6)
cv2.rectangle(src, (rect.left, rect.top), (rect.left + rect.width, rect.top + rect.height), (0,0,255), 2)
polygon = code.polygon
print(polygon)
box = np.intp(polygon)
print(type(box))
cv2.drawContours(src, [box], -1, (255,0, 0), 3)
result = cv2.resize(src,dsize=None,fx=0.2,fy=0.2,interpolation=cv2.INTER_LINEAR)
cv2.imshow("result", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
2、datamartix码识别
2.1实现方法:
使用现有的pylibdmtx进行datamartix码识别,该库为专门识别datamartix的库
from pylibdmtx import pylibdmtx
2.2实现步骤:
1)读取图片(不是灰度图的需要转化为灰度图);
2)高斯滤波,去除噪音干扰;
3)二值化,突出二维码黑白颜色区分;
4)调用pylibdmtx.decode库进行识别;
5)返回识别的信息,常为四个角点的坐标和二维码的信息。
2.3实现代码:
实现代码采用的是电脑生成的datamartix码比较清楚,所以未进行相关的滤波和二值化的预处理。
#!/usr/bin/python3
# -*- coding: utf-8 -*-
import cv2
from pylibdmtx import pylibdmtx
import time
# 加载图片
image = cv2.imread('DataMatrixcode.png')
# 解析二维码
all_barcode_info = pylibdmtx.decode(image, timeout=500, max_count=1)
print(all_barcode_info)
print(all_barcode_info[0].data.decode("utf-8"))
text=all_barcode_info[0].data.decode("utf-8")
cv2.putText(image, text, (5,20), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 0, 255), 1)
image = cv2.resize(image,dsize=None,fx=4,fy=4,interpolation=cv2.INTER_LINEAR)
cv2.imshow('image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
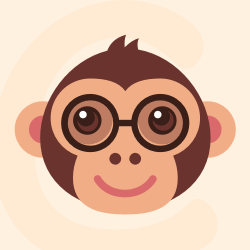



OpenCV: 开源计算机视觉库
最近提交(Master分支:2 个月前 )
48668119
dnn: use dispatching for Winograd optimizations 6 天前
3dace76c
flann: remove unused hdf5 header 6 天前
更多推荐
所有评论(0)