springboot启动后如何读取静态json资源文件
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
在Spring Boot应用程序启动后,您可以通过多种方式读取指定JSON资源文件。以下是一些常用的方法:
- 使用
ResourceLoader
import org.springframework.beans.factory.annotation.Autowired; import org.springframework.core.io.Resource; import org.springframework.core.io.ResourceLoader; import org.springframework.stereotype.Component; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; @Component public class JsonFileReader { @Autowired private ResourceLoader resourceLoader; public String readJsonFile(String filePath) { StringBuilder json = new StringBuilder(); try { Resource resource = resourceLoader.getResource("classpath:" + filePath); InputStream inputStream = resource.getInputStream(); BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream)); String line; while ((line = reader.readLine()) != null) { json.append(line); } reader.close(); } catch (Exception e) { e.printStackTrace(); } return json.toString(); } }
- 使用
ClassPathResource
import org.springframework.core.io.ClassPathResource; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; import java.util.stream.Collectors; public class JsonFileReader { public String readJsonFile(String filePath) { StringBuilder json = new StringBuilder(); try { ClassPathResource classPathResource = new ClassPathResource(filePath); InputStream inputStream = classPathResource.getInputStream(); String content = new BufferedReader(new InputStreamReader(inputStream)).lines() .collect(Collectors.joining("\n")); json.append(content); } catch (Exception e) { e.printStackTrace(); } return json.toString(); } }
- 使用
File
类(不推荐)
这不是推荐的方法,因为它假设JSON文件位于文件系统的固定位置,而不是在应用程序的类路径中。这在打包为JAR时可能不会正常工作。import java.nio.file.Files; import java.nio.file.Paths; public class JsonFileReader { public String readJsonFile(String filePath) { StringBuilder json = new StringBuilder(); try { Files.lines(Paths.get(filePath)).forEach(json::append); } catch (Exception e) { e.printStackTrace(); } return json.toString(); } }
- 使用
@Value
和Resource
import org.springframework.beans.factory.annotation.Value; import org.springframework.core.io.Resource; import org.springframework.stereotype.Component; import java.io.BufferedReader; import java.io.InputStream; import java.io.InputStreamReader; import java.util.stream.Collectors; @Component public class JsonFileReader { @Value("classpath:config.json") private Resource resource; public String readJsonFile() { try { InputStream inputStream = resource.getInputStream(); return new BufferedReader(new InputStreamReader(inputStream)) .lines().collect(Collectors.joining("\n")); } catch (Exception e) { e.printStackTrace(); return null; } } }
在这些示例中,filePath
是相对于类路径的JSON文件路径。例如,如果您有一个名为config.json
的文件位于src/main/resources
目录下,那么filePath
将是config.json
。
请注意,如果您正在读取配置文件,Spring Boot提供了更高级的配置文件处理机制,例如使用@ConfigurationProperties
或@Value
注解直接将配置属性注入到您的bean中。
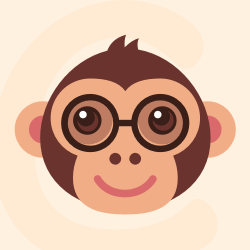



适用于现代 C++ 的 JSON。
最近提交(Master分支:2 个月前 )
960b763e
5 个月前
8c391e04
8 个月前
更多推荐
所有评论(0)