【面试官】springboot+vue3架构,跨域问题导致的前端接口状态码200,但是浏览器无响响应如何处理?
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
在Vue + Spring Boot架构中,如果你遇到了跨域问题导致前端接口状态码为200,但是浏览器无响应的情况,这通常是因为浏览器的CORS(跨源资源共享)策略阻止了请求的响应。尽管服务器返回了200状态码,但浏览器可能会忽略响应内容,因为它认为响应不安全。
以下是一些可能的解决方案:
1. Spring Boot配置CORS
确保Spring Boot应用正确配置了CORS。你可以在Spring Boot应用中全局配置CORS,或者为特定的控制器或方法配置CORS。
全局CORS配置
在Spring Boot的配置类中添加以下代码:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.cors.CorsConfiguration;
import org.springframework.web.cors.UrlBasedCorsConfigurationSource;
import org.springframework.web.filter.CorsFilter;
@Configuration
public class CorsConfig {
@Bean
public CorsFilter corsFilter() {
UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true); // 允许发送Cookie
config.addAllowedOrigin("*"); // 允许任何源
config.addAllowedHeader("*"); // 允许任何头
config.addAllowedMethod("*"); // 允许任何方法
source.registerCorsConfiguration("/**", config); // 对所有API生效
return new CorsFilter(source);
}
}
控制器或方法级别的CORS配置
在控制器或方法上使用@CrossOrigin
注解:
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MyController {
@CrossOrigin
@GetMapping("/data")
public String getData() {
return "This is the data you requested.";
}
}
2. Vue.js配置代理
在Vue项目中,你可以配置代理来避免跨域问题。在vue.config.js
中添加以下代理设置:
module.exports = {
devServer: {
proxy: {
'/api': {
target: 'http://localhost:8080', // Spring Boot服务器的地址
changeOrigin: true, // 是否改变源
pathRewrite: {
'^/api': '' // 重写路径: 去掉路径中的/api
}
}
}
}
};
这样,当你在Vue应用中发送请求到/api
时,它会被代理到http://localhost:8080
,从而避免了跨域问题。
3. 浏览器扩展或代理服务器
如果你正在开发环境中遇到这个问题,你可以使用浏览器扩展(如CORS Everywhere)或者设置一个代理服务器(如Charles)来临时绕过跨域限制。
4. 检查浏览器控制台和网络请求
在浏览器中,打开开发者工具,检查网络请求和响应。查看是否有任何CORS相关的错误信息,这可能会提供解决问题的线索。
确保你的前端代码中没有错误地处理了响应,例如,在Vue.js中,如果你使用了axios,确保你正确地处理了响应:
axios.get('/api/data')
.then(response => {
// 处理数据
console.log(response.data);
})
.catch(error => {
// 处理错误
console.error('请求失败:', error);
});
如果你已经正确配置了CORS,并且在前端正确处理了响应,但是仍然遇到问题,请确保没有其他中间件(如防火墙或反向代理服务器)阻止了CORS请求。
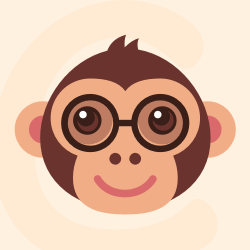



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:3 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 5 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 5 个月前
更多推荐
所有评论(0)