(四) carla中创建小车、采集数据

(四) Carla中创建小车、连接相机、采集图像
carla中放置小车、控制过程
参考安装包里面的
P
y
t
h
o
n
A
P
I
/
e
x
a
m
p
l
e
s
/
PythonAPI/examples/
PythonAPI/examples/ 中给出的一些学习程序,总结在
c
a
r
l
a
carla
carla 中放置小车、控制小车步骤。
1.首先,添加
c
a
r
l
a
carla
carla 包
#找到carla包的路径
try:
sys.path.append(glob.glob('../carla/dist/carla-*%d.%d-%s.egg' % (
sys.version_info.major,
sys.version_info.minor,
'win-amd64' if os.name == 'nt' else 'linux-x86_64'))[0])
except IndexError:
pass
import carla
s
y
s
.
p
a
t
h
.
a
p
p
e
n
d
sys.path.append
sys.path.append 将
g
l
o
b
glob
glob 定位到的dist文件夹中的
c
a
r
l
a
−
0.9.4
−
p
y
3.5
−
l
i
n
u
x
−
x
86
_
64.
e
g
g
carla-0.9.4-py3.5-linux-x86\_64.egg
carla−0.9.4−py3.5−linux−x86_64.egg 添加到
i
m
p
o
r
t
import
import 的路径当中,使得可以导入
c
a
r
l
a
carla
carla 包。
2.将
c
a
r
l
a
carla
carla 添加到路径后,开始创建客户端,与
c
a
r
l
a
carla
carla 进行相连。
#创建client连接到Carla中
client = carla.Client('localhost', 2000)
#seconds,设置连接超时时间
client.set_timeout(2.0)
3.然后获取世界,获取世界的方法有两种,一种直接加载当前 c a r l a carla carla 仿真环境中的地图所对应的 w o r l d world world;另一种是加载自己想要的地图,更改当前 c a r l a carla carla 中的仿真环境地图。
#方法一
#world = client.get_world()
#方法二
world = client.load_world('Town05')
4.获取 w o r l d world world 中可用的 B l u e p r i n t Blueprint Blueprint,从 B l u e p r i n t Blueprint Blueprint 中选取要创建的 a c t o r actor actor 模型。这里比如要创建一辆车。
#通过world获取world中的Blueprint_Library
blueprint_library = world.get_blueprint_library()
#通过find()和filter()函数以及random.choice随机选择一个车辆模型
my_vehicle_bp = random.choice(blueprint_library.filter("vehicle.lincoln.mkz2017"))
5.通过 t r a n s f o r m transform transform 创建车辆的生成位置,这里有两种方法,一种是人为手动设置生成点,但是这种情况经常因为选取不合理导致发生碰撞。另一种是从地图上随机选择一个不发生碰撞的点作为车辆的生成点。
#方法一,手动设置生成点
location = carla.Location(0, 10, 0)
rotation = carla.Rotation(0, 0, 0)
transform_vehicle = carla.Transform(location, rotation)
#方法二,自动选择生成点
#transform_vehicle = random.choice(world.get_map().get_spawn_points())
6.使用 s p a w n a c t o r spawn_actor spawnactor 在仿真环境中生成车辆
my_vehicle = world.spawn_actor(my_vehicle_bp, transform_vehicle)
7.在仿真环境中生成车辆以后,控制车辆行驶。控制车辆行驶可以选择手动控制,也可以选择调用自动驾驶模式。
#方法一,自动驾驶模式
my_vehicle.set_autopilot(enabled=True)
#方法二,手动控制模式
#my_vehicle.apply_control(carla.VehicleControl(throttle=1.0, steer=0.0))
至此,完成了小车的创建、控制。
整个代码如下:
#找到carla包的路径
try:
sys.path.append(glob.glob('../carla/dist/carla-*%d.%d-%s.egg' % (
sys.version_info.major,
sys.version_info.minor,
'win-amd64' if os.name == 'nt' else 'linux-x86_64'))[0])
except IndexError:
pass
import carla
##############################################################
#创建client连接到Carla中
client = carla.Client('localhost', 2000)
#seconds,设置连接超时时间
client.set_timeout(2.0)
##############################################################
#方法一
#world = client.get_world()
#方法二
world = client.load_world('Town05')
##############################################################
#通过world获取world中的Blueprint_Library
blueprint_library = world.get_blueprint_library()
#通过find()和filter()函数以及random.choice随机选择一个车辆模型
my_vehicle_bp = random.choice(blueprint_library.filter("vehicle.lincoln.mkz2017"))
##############################################################
#方法一,手动设置生成点
location = carla.Location(0, 10, 0)
rotation = carla.Rotation(0, 0, 0)
transform_vehicle = carla.Transform(location, rotation)
#方法二,自动选择生成点
#transform_vehicle = random.choice(world.get_map().get_spawn_points())
##############################################################
my_vehicle = world.spawn_actor(my_vehicle_bp, transform_vehicle)
##############################################################
#方法一,自动驾驶模式
my_vehicle.set_autopilot(enabled=True)
#方法二,手动控制模式
#my_vehicle.apply_control(carla.VehicleControl(throttle=1.0, steer=0.0))
carla中安装相机、采集图片过程
一般情况下,
c
a
r
l
a
carla
carla 中相机都是在车辆上附着的。因此,接下来就在上述车辆创建、控制的基础上进行增添相机处理过程。
1.获取
w
o
r
l
d
world
world 中可用的
B
l
u
e
p
r
i
n
t
Blueprint
Blueprint,从
B
l
u
e
p
r
i
n
t
Blueprint
Blueprint 中选取要创建的
a
c
t
o
r
actor
actor 模型。这里创建一个
R
G
B
RGB
RGB 相机。
#通过world获取world中的Blueprint_Library
blueprint_library = world.get_blueprint_library()
#选择相机模型
my_camera_bp = blueprint_library.find("sensor.camera.rgb")
2…设置
a
c
t
o
r
actor
actor 中相关传感器参数
这里设置相机采集图像的宽、高以及相机前方视角
IM_WIDTH = 640
IM_HEIIGHT = 480
# set the attribute of camera
my_camera_bp.set_attribute("image_size_x", "{}".format(IM_WIDTH))
my_camera_bp.set_attribute("image_size_y", "{}".format(IM_HEIIGHT))
my_camera_bp.set_attribute("fov", "90")
3.设置相机安装位置,这里是设置相机相对于附着物,在附着物坐标系下的位置。
transform_camera = carla.Transform(carla.Location(x=2.5, z=0.7))
4.生成相机,将相机安装在车上
my_camera = world.spawn_actor(my_camera_bp, transform_camera, attach_to=my_vehicle)
5.至此,相机安装完成。下面监听相机数据
想要读取相机实时采集的图片,一般通过传感器的
l
i
s
t
e
n
(
)
listen()
listen() 函数进行获取数据,同时,还可以使用回调函数对数据进行相应处理。例如:
#方法一,这里直接使用lambda进行处理,将图像进行存储
my_camera.listen(lambda image: image.save_to_disk('output/%06d.png' % image.frame_number))
#方法二,
def callback(event):
#event就是时时刻刻采集的image,可以对图片进行各种处理,然后存储
......
event.save_to_disk('_out/%08d' % event.frame)
my_camera.listen(callback)
整体代码
将相机这一部分加入刚才小车控制程序中。
记得在程序末尾加上运行时间以及运行结束销毁前面所有的
a
c
t
o
r
actor
actor 这个步骤,为了销毁方便,使用一个列表将添加过的
a
c
t
o
r
actor
actor 存起来。
整个代码如下:
#找到carla包的路径
try:
sys.path.append(glob.glob('../carla/dist/carla-*%d.%d-%s.egg' % (
sys.version_info.major,
sys.version_info.minor,
'win-amd64' if os.name == 'nt' else 'linux-x86_64'))[0])
except IndexError:
pass
import carla
def callback(event):
#event就是时时刻刻采集的image,可以对图片进行各种处理,然后存储
......
event.save_to_disk('_out/%08d' % event.frame)
#存创建的actor
actor_list = []
try:
##############################################################
#创建client连接到Carla中
client = carla.Client('localhost', 2000)
#seconds,设置连接超时时间
client.set_timeout(2.0)
##############################################################
#方法一
#world = client.get_world()
#方法二
world = client.load_world('Town05')
##############################################################
#通过world获取world中的Blueprint_Library
blueprint_library = world.get_blueprint_library()
#通过find()和filter()函数以及random.choice随机选择一个车辆模型
my_vehicle_bp = random.choice(blueprint_library.filter("vehicle.lincoln.mkz2017"))
#选择相机模型
my_camera_bp = blueprint_library.find("sensor.camera.rgb")
##############################################################
#方法一,手动设置生成点
location = carla.Location(0, 10, 0)
rotation = carla.Rotation(0, 0, 0)
transform_vehicle = carla.Transform(location, rotation)
#方法二,自动选择生成点
#transform_vehicle = random.choice(world.get_map().get_spawn_points())
##############################################################
my_vehicle = world.spawn_actor(my_vehicle_bp, transform_vehicle)
actor_list.append(my_vehicle)
##############################################################
IM_WIDTH = 640
IM_HEIIGHT = 480
# set the attribute of camera
my_camera_bp.set_attribute("image_size_x", "{}".format(IM_WIDTH))
my_camera_bp.set_attribute("image_size_y", "{}".format(IM_HEIIGHT))
my_camera_bp.set_attribute("fov", "90")
##############################################################
transform_camera = carla.Transform(carla.Location(x=2.5, z=0.7))
##############################################################
my_camera = world.spawn_actor(my_camera_bp, transform_camera, attach_to=my_vehicle)
actor_list.append(my_camera)
##############################################################
#方法一,自动驾驶模式
my_vehicle.set_autopilot(enabled=True)
#方法二,手动控制模式
#my_vehicle.apply_control(carla.VehicleControl(throttle=1.0, steer=0.0))
##############################################################
#方法一,这里直接使用lambda进行处理,将图像进行存储
my_camera.listen(lambda image: image.save_to_disk('output/%06d.png' % image.frame_number))
#方法二,
#my_camera.listen(callback)
time.sleep(1000)
finally:
for actor in actor_list:
actor.destroy()
print("All cleaned up!")
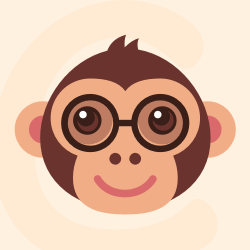



更多推荐
所有评论(0)