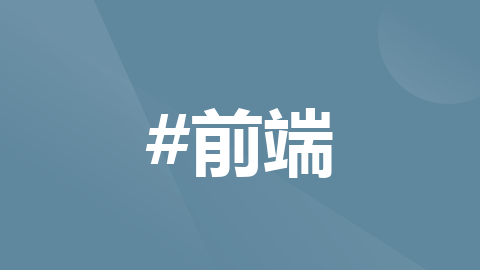
vue3使用Ant-Design组件a-table表格实现多层表头及合并单元格
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
vue3使用Ant-Design组件a-table表格实现多层表头及合并单元格
效果图:
多层表头: 通过columns中的children来设置多个值实现多层表头;
表身单元格合并: 从图中可以看出第一列(班次)和最后一列(值班员签名)需要表格行合并单元格(通过表格的某个字段中相同值进行合并);
整体代码如下:
<template>
<div class="tableContent">
<a-table :columns="columns" :data-source="dataSource" bordered size="small" :pagination="false"
:scroll="{ y: 640 }">
</a-table>
</div>
</template>
<script lang="ts" setup>
import { ref } from 'vue';
const columns = [
{
title: '班次',
dataIndex: 'tableTime',
key: 'tableTime',
width: 50,
align: 'center',
customCell: ({ rowSpan }) => {
return {
rowSpan: rowSpan
}
}
},
{
title: '时间',
dataIndex: 'time',
key: 'time',
width: 100,
align: 'center'
},
{
title: '直供井',
children: [
{
title: 'PH',
dataIndex: 'PH1',
key: 'PH1',
width: 80,
align: 'center'
},
{
title: '浊度(NTU)',
dataIndex: 'turbidity1',
key: 'turbidity1',
width: 80,
align: 'center'
},
{
title: '电导率(μs/cm)',
dataIndex: 'conductivity1',
key: 'conductivity1',
width: 80,
align: 'center'
},
{
title: '温度(°C)',
dataIndex: 'temperature1',
key: 'temperature1',
width: 80,
align: 'center'
},
{
title: '余氯(mg/L)',
dataIndex: 'residual1',
key: 'residual1',
width: 80,
align: 'center'
},
],
},
{
title: '出厂水',
children: [
{
title: 'PH',
dataIndex: 'PH2',
key: 'PH2',
width: 80,
align: 'center'
},
{
title: '浊度(NTU)',
dataIndex: 'turbidity2',
key: 'turbidity2',
width: 80,
align: 'center'
},
{
title: '电导率(μs/cm)',
dataIndex: 'conductivity2',
key: 'conductivity2',
width: 80,
align: 'center'
},
{
title: '温度(°C)',
dataIndex: 'temperature2',
key: 'temperature2',
width: 80,
align: 'center'
},
{
title: '余氯(mg/L)',
dataIndex: 'residual2',
key: 'residual2',
width: 80,
align: 'center'
},
],
},
{
title: '管网末梢水',
children: [
{
title: 'PH',
dataIndex: 'PH3',
key: 'PH3',
width: 80,
align: 'center'
},
{
title: '浊度(NTU)',
dataIndex: 'turbidity3',
key: 'turbidity3',
width: 80,
align: 'center'
},
{
title: '电导率(μs/cm)',
dataIndex: 'conductivity3',
key: 'conductivity3',
width: 80,
align: 'center'
},
{
title: '温度(°C)',
dataIndex: 'temperature3',
key: 'temperature3',
width: 80,
align: 'center'
},
{
title: '余氯(mg/L)',
dataIndex: 'residual3',
key: 'residual3',
width: 80,
align: 'center'
},
],
},
{
title: '值班员签名',
dataIndex: 'signature',
key: 'signature',
width: 100,
align: 'center',
customCell: ({ rowSpan }) => {
return {
rowSpan: rowSpan
}
}
},
];
const dataSource = ref([
{ tableTime: '早班', time: 1, PH1: 7.61, turbidity1: 0.32, conductivity1: 671.61, temperature1: 23.73, residual1: 0.02, PH2: 7.61, turbidity2: 0.32, conductivity2: 671.61, temperature2: 23.73, residual2: 0.02, PH3: 7.61, turbidity3: 0.32, conductivity3: 671.61, temperature3: 23.73, residual3: 0.02, signature: '张三' },
{ tableTime: '早班', time: 2, PH1: 7.61, turbidity1: 0.32, conductivity1: 671.61, temperature1: 23.73, residual1: 0.02, PH2: 7.61, turbidity2: 0.32, conductivity2: 671.61, temperature2: 23.73, residual2: 0.02, PH3: 7.61, turbidity3: 0.32, conductivity3: 671.61, temperature3: 23.73, residual3: 0.02, signature: '张三' },
{ tableTime: '中班', time: 9, PH1: 7.61, turbidity1: 0.32, conductivity1: 671.61, temperature1: 23.73, residual1: 0.02, PH2: 7.61, turbidity2: 0.32, conductivity2: 671.61, temperature2: 23.73, residual2: 0.02, PH3: 7.61, turbidity3: 0.32, conductivity3: 671.61, temperature3: 23.73, residual3: 0.02, signature: '李四' },
{ tableTime: '中班', time: 10, PH1: 7.61, turbidity1: 0.32, conductivity1: 671.61, temperature1: 23.73, residual1: 0.02, PH2: 7.61, turbidity2: 0.32, conductivity2: 671.61, temperature2: 23.73, residual2: 0.02, PH3: 7.61, turbidity3: 0.32, conductivity3: 671.61, temperature3: 23.73, residual3: 0.02, signature: '李四' },
{ tableTime: '中班', time: 11, PH1: 7.61, turbidity1: 0.32, conductivity1: 671.61, temperature1: 23.73, residual1: 0.02, PH2: 7.61, turbidity2: 0.32, conductivity2: 671.61, temperature2: 23.73, residual2: 0.02, PH3: 7.61, turbidity3: 0.32, conductivity3: 671.61, temperature3: 23.73, residual3: 0.02, signature: '李四' },
{ tableTime: '晚班', time: 17, PH1: 7.61, turbidity1: 0.32, conductivity1: 671.61, temperature1: 23.73, residual1: 0.02, PH2: 7.61, turbidity2: 0.32, conductivity2: 671.61, temperature2: 23.73, residual2: 0.02, PH3: 7.61, turbidity3: 0.32, conductivity3: 671.61, temperature3: 23.73, residual3: 0.02, signature: '王五' },
{ tableTime: '晚班', time: 18, PH1: 7.61, turbidity1: 0.32, conductivity1: 671.61, temperature1: 23.73, residual1: 0.02, PH2: 7.61, turbidity2: 0.32, conductivity2: 671.61, temperature2: 23.73, residual2: 0.02, PH3: 7.61, turbidity3: 0.32, conductivity3: 671.61, temperature3: 23.73, residual3: 0.02, signature: '王五' },
])
//合并单元格方法
function groupData(data) {
let currentTableTime = '';
let currentSignature = '';
return data.map((item, index) => {
if (currentTableTime !== item.tableTime || currentSignature !== item.signature) {
currentTableTime = item.tableTime;
currentSignature = item.signature;
let rowSpan = 0;
for (let i = 0; i < data.length; i++) {
if (i >= index) {
const currentItem = data[i];
if (
currentTableTime === currentItem.tableTime && currentSignature === currentItem.signature
) {
rowSpan += 1;
} else {
break;
}
}
}
item.rowSpan = rowSpan;
} else {
item.rowSpan = 0;
}
return item;
});
}
groupData(dataSource.value)
</script>
<style lang="less" scoped></style>
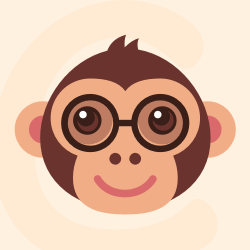



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:2 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 4 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 5 个月前
更多推荐
所有评论(0)