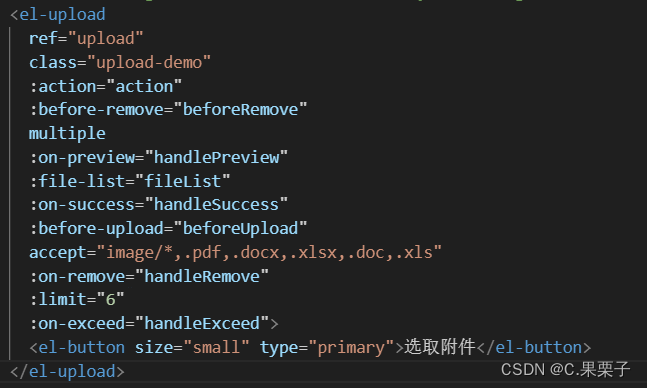
element-ui附件上传及在线查看详细总结,后续赋源码

一、附件上传
1、在element-ui上面复制相应代码
a、accept="image/*,.pdf,.docx,.xlsx,.doc,.xls" 是规定上传文件的类型,若是不限制,可以直接将accept=‘all'即可;
b、:action="action" 这个属性就是你的上传附件的地址;
一般情况下,上传一个文件,后端会给两个接口,第一个接口就是写在action里面的,这个接口的作用是返回一个id或则一个其他的唯一属性;接着第二个接口就是上传附件的接口,这个返回的唯一属性会被当做第二个接口的参数提交,此时就已经完成了附件的上传。
二、在线查看
1、首先先安装依赖包
用的是vue-office,地址:vue-office简介 | vue-office (501351981.github.io)
#docx文档预览组件
npm install @vue-office/docx vue-demi
#excel文档预览组件
npm install @vue-office/excel vue-demi
#pdf文档预览组件
npm install @vue-office/pdf vue-demi
//如果是vue2.6版本或以下还需要额外安装 @vue/composition-api
npm install @vue/composition-api
2、在vue文件中引入vue-office
3、开始判断文件的类型
接着我们要想实时的看到自己的附件,那么肯定一点就是得区分我们得附件类型
判断文件的类型我这里有两个方法推荐:
第一种:就是运用计算属性和includes来设置一个变量,再根据变量使用v-if控制显示
第二种:js的endsWith() 该方法用于测试字符串是否以指定的后缀结束,将获取到的文件名放入该方法中,判断后缀类型,不过这里的判断返回的是布尔值,然后再配合v-if使用以控制显示。
4、添加点击事件
这里设计的在线预览是点击附件后直接在下方显示
找到上传附件时的钩子,根据业务需求,判断是上传之前可以看还是上传之后可以看,有两种情况
第一种:在上传附件之前就想查看
这种情况我先放一下,因为这里我先讲得是直接上传附件,在上传之前查看,一般用在手动上传的时候,我后续再更,但是放心,我都会更新记录下来的~~
第二种:在上传附件成功之后再查看
给附件绑定一个点击事件:on-preview="handlePreview",绑定这个事件之后,可以获取到参数里面的file,即一个对象,将这个对象赋值给一个新的对象this.currentFile = file,而这个currentFile在判断类型时不可缺少的;之后,取currentFile里面raw赋值给vue-office标签里的src属性就可以了
第三种:同时拥有,哈哈哈哈这种就把前两种都写上就行了
目前这一块我是以组件的形式在使用,毕竟用的比较多,这样更方便些,以上是我自己的总结,主要是给自己看的,因为我有健忘,一段时间不用就会忘记,大家要是有疑问可以随时私信我,我看到了就会回复,毕竟学习也是相互的,加油。
以下是源码----------------------------------
<template>
<div>
<!-- <el-dialog title="上传附件" :visible.sync="dialogFormVisible" width="50%" append-to-body="true"> -->
<el-upload
ref="upload"
class="upload-demo"
:action="action"
:before-remove="beforeRemove"
multiple
:on-preview="handlePreview"
:file-list="fileList"
:on-success="handleSuccess"
:before-upload="beforeUpload"
accept="image/*,.pdf,.docx,.xlsx,.doc,.xls"
:on-remove="handleRemove"
:limit="6"
:on-exceed="handleExceed">
<el-button size="small" type="primary">选取附件</el-button>
</el-upload>
<!-- 查看 -->
<div v-if="currentFile">
<div v-if="currentFileType === 'excel'" class="officeShow">
<vue-office-excel :src="fileSrc" style="height: 40vh;width: 100%;" />
</div>
<div v-else-if="currentFileType === 'pdf'" class="officeShow">
<vue-office-pdf :src="fileSrc" style="height: auto;width: 100%;" />
</div>
<div v-else-if="currentFileType === 'word'" class="officeShow">
<vue-office-docx :src="fileSrc" style="height: 40vh;width: 100%;overflow: scroll;" />
</div>
<div v-else class="officeShow">
<img :src="fileSrc" style="height: auto;width: 100%;">
</div>
</div>
<!-- <div slot="footer" class="dialog-footer">
<el-button @click="cancellation">取 消</el-button>
<el-button type="primary" @click="save">保 存</el-button>
<el-button type="primary" @click="upClick">上传</el-button>
</div> -->
<!-- </el-dialog> -->
</div>
</template>
<script>
// 引入VueOfficePdf组件
import VueOfficePdf from '@vue-office/pdf'
// docx
import VueOfficeDocx from '@vue-office/docx'
import '@vue-office/docx/lib/index.css'
// 引入VueOfficeExcel组件
import VueOfficeExcel from '@vue-office/excel'
// 引入相关样式
import '@vue-office/excel/lib/index.css'
export default {
components: {
VueOfficeExcel,
VueOfficePdf,
VueOfficeDocx
},
props: {
projectId: {
type: String,
default: ''
}
},
data() {
return {
dialogFormVisible: false,
action: process.env.VUE_APP_BASE_API + '/file/upload',
fileList: [],
currentFile: null,
files: []
}
},
computed: {
currentFileType() {
let type = ''
if (this.currentFile.name) {
const arr = this.currentFile.name && this.currentFile.name.split('.')
type = arr[arr.length - 1]
}
switch (true) {
case ['xls', 'xlsx'].includes(type):
return 'excel'
case ['doc', 'docx'].includes(type):
return 'word'
case ['pdf'].includes(type):
return 'pdf'
default:
return 'img'
}
},
fileSrc() {
if (this.currentFileType === 'img') {
const windowURL = window.URL || window.webkitURL
const src = windowURL.createObjectURL(this.currentFile.raw)
return src
} else {
return this.currentFile.raw
}
}
},
methods: {
show() {
this.dialogFormVisible = true
},
cancellation() {
this.dialogFormVisible = false
this.fileList = []
},
// 暂存
// save() {
// this.dialogFormVisible = false
// this.$notify({
// title: '成功',
// message: '已保存',
// type: 'success',
// duration: 1000
// })
// },
handleRemove(file, fileList) {
this.fileList = fileList
// 判断溢出的文件是否当前预览中的文件
if (fileList.findIndex(item => item.uid === this.currentFile.uid) === -1) {
this.currentFile = null
}
},
handlePreview(file) {
// console.log(file)
this.currentFile = file
},
handleExceed(files, fileList) {
this.$message.warning(`当前限制选择 6 个文件,本次选择了 ${files.length}个文件,共选择了 ${files.length + fileList.length}个文件`)
},
beforeRemove(file, fileList) {
return this.$confirm(`确定移除 ${file.name} ?`)
},
handleSuccess(response, file, fileList) {
console.log(response, file, fileList)
this.files.push(response)
},
beforeUpload(file) {
const isLt20M = file.size / 1024 / 1024 < 20
if (!isLt20M) {
this.$message.error('上传文件大小不能超过 20MB!')
}
return isLt20M
}
}
}
</script>
<style lang="scss" scoped></style>
.....后续马上更,我有其他事情需要去处理一下
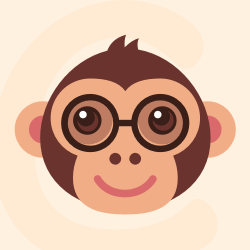



更多推荐
所有评论(0)