vue中Element-ui 表格 (Table)合并行、列单元格
element
A Vue.js 2.0 UI Toolkit for Web
项目地址:https://gitcode.com/gh_mirrors/eleme/element

·
实现效果
实现方式
先在el-table里加个属性:span-method绑定方法objectSpanMethod(),这个用来把你想合并的列根据你写的逻辑来合并,再写个getSpanArr(),这个写合并的逻辑。
快速应用
加一个属性两个方法,然后在获取表格数据的时候调用一下getSpanArr(),示例代码写了四个逻辑,根据id相同合并,根据id和其他字段相同登则进行合并,执行完后会得到四个tableArr数组,记录了每个逻辑需要合并的列数,objectSpanMethod()里根据你的合并逻辑来合并这些列,哪些列是用哪个逻辑你就用哪个数组,改成你想要的模样。
注意事项
你的后端传过来的数据要排序好,因为getSpanArr()里是判断当前元素与上一个元素是否相同来进行合并的。
代码实现
<el-table
v-loading="loading"
:data="tableData"
@selection-change="handleSelectionChange"
highlight-current-row
style="width: 100%"
:span-method="objectSpanMethod"
stripe
>
// 数据指定列动态合并行
getSpanArr() {
// 把需要合并行的归类, this.xxx是表格数据
this.tableOneArr = [];
this.tableOnePos = 0;
this.tableTwoArr = [];
this.tableTwoPos = 0;
this.tableThreeArr = [];
this.tableThreePos = 0;
this.tableFourArr = [];
this.tableFourPos = 0;
for (var i = 0; i < this.tableData.length; i++) {
if (i === 0) {
// 第一行必须存在
this.tableOneArr.push(1);
this.tableOnePos = 0;
this.tableTwoArr.push(1);
this.tableTwoPos = 0;
this.tableThreeArr.push(1);
this.tableThreePos = 0;
this.tableFourArr.push(1);
this.tableFourPos = 0;
} else {
// 判断当前元素与上一个元素是否相同
if (this.tableData[i].id === this.tableData[i - 1].id) {
this.tableOneArr[this.tableOnePos] += 1;
this.tableOneArr.push(0);
} else {
this.tableOneArr.push(1);
this.tableOnePos = i;
}
//判断当前元素是否与上一元素相同且为空
if (this.tableData[i].id === this.tableData[i - 1].id &&
this.tableData[i].itemNum === null && this.tableData[i].itemStdName === null && this.tableData[i].testMethod === null && this.tableData[i].stdChName === null && this.tableData[i].testCycle === null && this.tableData[i].splQty === null && this.tableData[i].subcCycle === null && this.tableData[i].priceInclTax === null && this.tableData[i].priceExTax === null && this.tableData[i].remark === null) {
this.tableTwoArr[this.tableTwoPos] += 1;
this.tableTwoArr.push(0);
} else {
this.tableTwoArr.push(1);
this.tableTwoPos = i;
}
//判断当前元素是否与上一元素相同且为空
if (this.tableData[i].id === this.tableData[i - 1].id &&
this.tableData[i].contractNo === null) {
this.tableThreeArr[this.tableThreePos] += 1;
this.tableThreeArr.push(0);
} else {
this.tableThreeArr.push(1);
this.tableThreePos = i;
}
//判断当前元素是否与上一元素相同且为空
if (this.tableData[i].id === this.tableData[i - 1].id &&
this.tableData[i].qualName === null && this.tableData[i].qualNum === null && this.tableData[i].deadline === null) {
this.tableFourArr[this.tableFourPos] += 1;
this.tableFourArr.push(0);
} else {
this.tableFourArr.push(1);
this.tableFourPos = i;
}
}
}
// 表格序号
let numSort = 0
for (const n in this.tableOneArr) {
if (this.tableOneArr[n] > 0) {
numSort += 1
this.$set(this.tableData[n], 'numSort', numSort)
}
}
},
objectSpanMethod({ row, column, rowIndex, columnIndex }) {
if (
columnIndex === 0 || columnIndex === 1 || columnIndex === 2 || columnIndex === 3 || columnIndex === 15 || columnIndex === 19 || columnIndex === 20 || columnIndex === 21
) {
// 第一列的合并方法,省
const _row = this.tableOneArr[rowIndex]
const _col = _row > 0 ? 1 : 0 // 如果被合并了_row=0则它这个列需要取消
return {
rowspan: _row,
colspan: _col
}
}else if(
columnIndex === 4 || columnIndex === 5 || columnIndex === 6 ||columnIndex === 7 || columnIndex === 8 || columnIndex === 9 || columnIndex === 10 || columnIndex === 11 || columnIndex === 12 || columnIndex === 13
){
const _row = this.tableTwoArr[rowIndex]
const _col = _row > 0 ? 1 : 0
return {
rowspan: _row,
colspan: _col
}
}else if(
columnIndex === 14
){
const _row = this.tableThreeArr[rowIndex]
const _col = _row > 0 ? 1 : 0
return {
rowspan: _row,
colspan: _col
}
}else if(
columnIndex === 16 || columnIndex === 17 || columnIndex === 18
){
const _row = this.tableFourArr[rowIndex]
const _col = _row > 0 ? 1 : 0
return {
rowspan: _row,
colspan: _col
}
}
},
详细说明
getSpanArr()这个函数就是用来返回 spanArr 数组的,定义每一行的 rowspan
if( index === 0),第一行,直接先给数组 push 进一个1,表示自己先占一行,position 是数组元素的位置
(此时是从数组元素的第一个开始,所以position 为 0), position为 0 意思表示的就是数组的第一个元素。
当到了 index 为 2 的时候,if(this.tableData[i].id === this.tableData[i - 1].id),
让第二行与第一行作比较:
(1)如果第二行与第一行相等的话,position 就 +1,当有 n 行第一行相同,position 就为 n,表示向下合并 n 行;
第二行自己就 spanArr.push(0),表示第二行“消失”,因为第一行和第二行合并了;
(2)如果第二行与第一行不相等的话,那么 spanArr.push(1);就让第二行自己独占一行;
position = index :把指针拿到 index 这行来,表示设置数组 spanArr[position] 的元素值,然后定义从此行开始向下合并几行
(可以根据示例研究下,当 index 为 2 时,position 为 2,当 index 为 3 时,第四行与第三行需要合并,
那么在数组的 position 元素就要 +1 了,也就是 spanArr[position] += 1)
:span-method="objectSpanMethod"
这个是官方给定的绑定属性和对应的方法,objectSpanMethod 传入了 { row, column, rowIndex, columnIndex }
row: 当前行
column: 当前列
rowIndex:当前行号
columnIndex :当前列号
该函数可以返回一个包含两个元素的数组,第一个元素代表rowspan,第二个元素代表 colspan。
也可以返回一个键名为 rowspan 和 colspan 的对象。
const _col = _row > 0 ? 1 : 0; 定义的这个单元格列的合并,我们项目只合并行,不合并列;
_row:代表合并行的行数,_row 的值要么是 1,或者更大的自然正整数,要么是 0。
1代表:独占一行
更大的自然数:代表合并了若干行
0:代表“消失”的哪那一个单元格,后面的单元格向前推一格
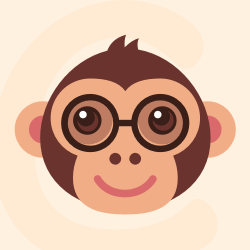



A Vue.js 2.0 UI Toolkit for Web
最近提交(Master分支:1 个月前 )
c345bb45
5 个月前
a07f3a59
* Update transition.md
* Update table.md
* Update transition.md
* Update table.md
* Update transition.md
* Update table.md
* Update table.md
* Update transition.md
* Update popover.md 5 个月前
更多推荐
所有评论(0)