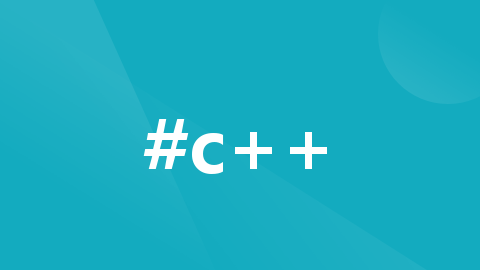
C++-openssl-aes-加密解密
openssl
传输层安全性/安全套接层及其加密库
项目地址:https://gitcode.com/gh_mirrors/ope/openssl

·
hmac Hash-based Message Authentication Code
MAC 定义: Message Authentication Code 一种确认完整性并进行认证的技术。
1.openssl基本版 加密解密
#include "openssl/rand.h"
#include "openssl/md5.h"
#include "openssl/hmac.h"
#include "openssl/aes.h"
//1.向量在运算过程中会被改变,为了之后可以正常解密,拷贝一份副本使用
void main()
{
unsigned char key[16] = "123456789ABCDEF"; //1.key
unsigned int n_keylength = strlen((char*)key); // strlen()长度15
unsigned char iv[16] = "123456789ABCDEF"; //2.偏移量
unsigned char iv_copy1[16] = "123456789ABCDEF"; //2.偏移量副本1
unsigned char iv_copy2[16] = "123456789ABCDEF"; //2.偏移量副本2
unsigned int n_ivlength = strlen((char*)iv); // strlen()长度15
unsigned char buf_in[16] = "123456789ABCDEF"; //3.明文 in
unsigned char buf_encrypt[16] = ""; //4.明文加密encrypt
unsigned char buf_out[16] = ""; //5.解密后明文out
AES_KEY aesKey;
//加密
//memcpy(iv_copy, iv, 16);
AES_set_encrypt_key(key, 128, &aesKey);
int m_bufinlen = sizeof(buf_in); // sizeof() 16
AES_cbc_encrypt(buf_in, buf_encrypt, sizeof(buf_in), &aesKey, iv_copy1, 1);
//解密
//memcpy(iv_copy, iv, 16);
AES_set_decrypt_key(key, 128, &aesKey);
int m_bufencryptlen = sizeof(buf_encrypt); // sizeof() 16
AES_cbc_encrypt(buf_encrypt, buf_out, sizeof(buf_encrypt), &aesKey, iv_copy2, 0);
}
2. 字符拼接
2.1C中的字符拼接
//1.snprintf 长度+1
#include <iostream> // C++的头文件不带.h
#include <string.h> // 兼容原C语言头文件
using namespace std;
//
int main()
{
//1. snprintf 复制拼接 size值为待拷贝字符串长度+1,保证'\0'结尾符加入进来。
char dest1[10] = "abc"; // 指针类型无法扩展长度,需标识为数组形式
char *src1 = "def";
snprintf(dest1+3, strlen(src1)+1, "%s", src1 ); // dest1+3 = dest1+3*sizeof(char)
//strlen(src1)+1 因为最后一个\0
// dest1: abcdef
//2.把 src 所指向的字符串复制到 dest,最多复制 n 个字符
// 当 src 的长度小于 n 时,dest 的剩余部分将用空字节填充
char dest2[10] = "abcd";
char *src2 = "efg";
strncpy(dest2+3, src2, strlen(src2)); //dest2: abcefg
//3.
char dest3[10] = "abc";
char *src3 = "def";
strncat(dest3, src3 , strlen(src3 )+1); // dest3:abcdef
return 0;
}
2.2 C++中的字符拼接
#include <iostream> // C++的头文件不带.h
#include <string.h> // 兼容原C语言头文件
using namespace std;
int main()
{
//char s1[] = {'a','b','c', '\0'}; // 定义1:不建议使用此定义方式,经常忘记加入'\0'
//char s1[] = "abc"; // 定义2:使用C语言数组形式定义字符串
string s1 = "abc"; // 定义3:使用string类定义
string s2 = "def";
string dest;
dest = s1+s2; //1.直接+ dest 输出 abcdef
printf("%s");
return 0;
}
3 c/c++字符串格式化输出
#include <stdio.h>
int main() {
int num = 42;
printf("The answer is %d\n", num);
char dest[50];
sprintf(dest, "The answer is %d", num);
printf("%s\n", dest);
return 0;
}
sprintf sprintf_s的区别
4 双引号
string str = "{\"OperatorID\":\"";
str = str + s_config_operatorid_;
str=str+ ",\"OperatorSecret\":\"";
str = str + s_config_operatorsecret_;
str = str + "\"}";
char csrc[256]="";
sprintf_s(csrc,256,"{\"OperatorID\":\" %s\"\",\"OperatorSecret:\"%s\"}",s_config_operatorid_.c_str(),s_config_operatorsecret_.c_str());
5 time
localtime_s | Windows |
localtime_r | linux |
//WINDOWS
void main()
{
string stime;
struct tm tnow;
time_t now = time(NULL);
localtime_s(&tnow, &now);
char buf_time[sizeof("yymmddhhmmss")] = { '\0' };
sprintf(buf_time, "%02d%02d%02d%02d%02d%02d", tnow.tm_year+1900, tnow.tm_mon+1,
tnow.tm_mday, tnow.tm_hour, tnow.tm_min, tnow.tm_sec);
}
linux
#include <stdio.h>
#include <time.h>
int main()
{
time_t time_seconds = time(0);
struct tm* now_time = localtime_r(&time_seconds);
printf("%d-%d-%d %d:%d:%d\n", now_time->tm_year + 1900, now_time->tm_mon + 1,
now_time->tm_mday, now_time->tm_hour, now_time->tm_min,
now_time->tm_sec);
}
g++ -std=c++11 lolcatimetest.cpp -o localtimetest
./localtimetest
6 char 转string
// char 转换成string 3种
void main()
{
unsigned char bufc1[5]="ABCD";
string s1((char*)bufc1,5); //1.使用 string 的构造函数
unsigned char bufc2[5]="1234";
string s2; //2.声明string 后将char push_back
for (int i = 0; i < 5; i++)
{
s2.push_back(bufc2[i]);
}
unsigned char bufc3[5]="9876";
stringstream ss; //3.使用stringstream
ss << bufc3;
string str3 = ss.str();
}
7. 对比两个string
void main()
{
string A("aBcdef");
string B("AbcdEf");
string C("123456");
string D("123dfg");
string E("aBcdef");
int m = A.compare(E); //完整的A和E的比较 2个相同返回0
int l = A.compare(B); //完整的A和B的比较 2个不同返回1
int n = A.compare(1, 5, B, 0, 5); //"Bcdef"和"AbcdEf"比较 //不同返回1
int p = A.compare(1, 5, B, 4, 2); //"Bcdef"和"Ef"比较 返回ff
int q = C.compare(0, 3, D, 0, 3); //"123"和"123"比较 //相同返回0
}
8. 字符串中的字符无效
1.如果已经分配过内存,可能是越界导致的指令错误。
2.可能是字符数组成员的值超出了ASCII码表示范围,导致字符无效
如:arr[[1]]=0x89;
此时,在调试时,就会显示"字符串中的字符无效".
格式化输出:可以打印
void printf_buff(char* buff, int size)
{
int i = 0;
for (i = 0; i < size; i++)
{
printf("%02X ", (unsigned char)buff[i]); //每个字符以16进制输出
if ((i + 1) % 8 == 0) //每8个字节换行
{
printf("\n");
}
}
printf("\n\n\n\n"); //空4行
}
9.cout和printf 区别
cout | printf | |
不需要格式 cout<<是一个函数,cout<<后可以跟不同的类型 | 需要指定格式 | |
#include <iostream> | #include <stdio.h> | |
在linux中,当遇到 \n 回车换行符时,进行IO操作输入输出 |
void main()
{
int a=6;
cout<<a<<endl;
printf("%d\n",a); //需要告诉他格式
}
int main()
{
cout << "Hello world!" << endl;//C++
cout.operator<<("Hello,World!");
cout.operator<<(endl);
printf("Hello world!"); //C
return 0;
}
1.printf是函数。cout是ostream对象,和<<配合使用。
2.printf是变参函数,没有类型检查,不安全。cout是通过运算符重载实现的,安全。
3.如果printf碰到不认识的类型就没办法了,而cout可以自己重载进行扩展。
4.有时候printf比cout灵活。
c++中也能使用printf,但是c中不能使用cout
10. new malloc区别
new | malloc | |
new是关键字,需要编译器支持 | malloc是库函数,需要头文件支持 | |
从自由存储区(free store)上为对象动态分配内存空间 | 从堆上动态分配内存 | |
void main()
{
int* p=new int; //分配大小为sizeof(int)的空间 4字节
int* p=new int(6); //分配大小为sizeof(int)的空间,并且初始化为6
int* p=(int)malloc(sizeof(int)100);//分配可以放下100个int的内存空间
int* ptr=new int[100];//分配100个int的内存空间
int* p=(int)malloc(sizeof(int)100);//分配可以放下100个int的内存空间
}
11.printf打印格式
// %x 以16进制打印一个整数(4字节)
// hh: char 1个字节
// h: short 2个字节
char c[] = "12345678";
int i= 12345678;
printf("char-----%02hhx,%02hx,%02x\n", c, c, c); // e4,fce4,12ffce4
// 从后往前
printf("int -----%02hhx,%02hx,%02x\n", i, i, i); // 4e,614e,bc614e
printf("-----%s\n", c); //12345678
printf("-----%c\n", c); //@
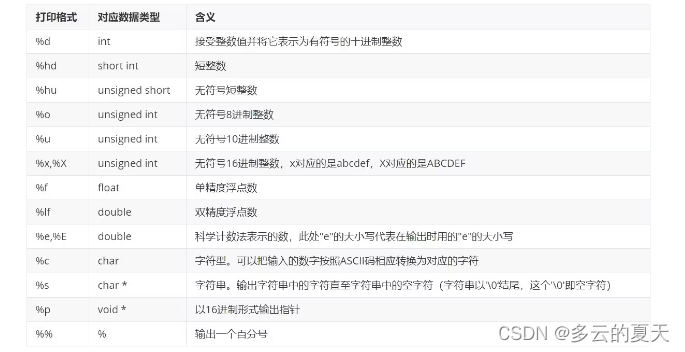
12.字符串转16进制输出
char* str2hex(char* strin)
{
char* ret = NULL; //0.返回字符串
int str_len = strlen(strin); //1.输入字符串strin
assert((str_len % 2) == 0); //2.判断输入长度是否为2的倍数 如果不是返回
ret = (char*)malloc(str_len / 2); //3.分配一个空间
for (int i = 0; i < str_len; i = i + 2) //4.
{
sscanf(strin + i, "%2hhx", &ret[i / 2]); //5. 格式化放入 ret中
}
// printf("%s\n", ret);
return ret;
}
void printf_buff(char* buff, int size)
{
int i = 0;
for (i = 0; i < size; i++)
{
// printf("i=%d %02X ,", i,(unsigned char)buff[i]); //每个字符以16进制输出
printf("%02X ", (unsigned char)buff[i]);
if ((i + 1) % 8 == 0) //每8个字节换行
{
printf("\n");
}
}
printf("\n\n\n\n"); //空4行
}
void main()
{
char in1[] = "0123456789ABCDEF0123456789\0";
char* out1 = str2hex((char*)in1);
int m_outlen1 = strlen(out1);
printf("---in1\n");
printf_buff(out1, m_outlen1);
char in2[] = "0123456789ABCDEF\0";
char *out2 = str2hex((char*)in2);
int m_outlen2 = strlen(out2);
printf("---in2\n");
printf_buff(out2, m_outlen2);
}
“%02x” 以0补齐2位数,如果超过2位就显示实际的数;
"%hhx" 是只输出2位数,即便超了,也只显示低2位。
“%02hhx”
只能对字符串为16进制的数字做正确转换,字符串个数还不对。
13.byte和char 区别
头文件<windows> 定义了 byte
byte | char | |
有符号 -128-127 | 无符号 0-65535 | |
不可以 | 可以表中文 | |
输出结果都会转化成数字 | char 输出结果都会转化为字符 | |
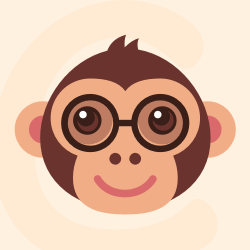



传输层安全性/安全套接层及其加密库
最近提交(Master分支:1 个月前 )
fd39d1c8
Reviewed-by: Tomas Mraz <tomas@openssl.org>
Reviewed-by: Shane Lontis <shane.lontis@oracle.com>
(Merged from https://github.com/openssl/openssl/pull/25095)
2 个月前
ae87c488
Reviewed-by: Tomas Mraz <tomas@openssl.org>
Reviewed-by: Shane Lontis <shane.lontis@oracle.com>
(Merged from https://github.com/openssl/openssl/pull/25095)
2 个月前
更多推荐
所有评论(0)