Java中JSON数据的读取和解析
json
适用于现代 C++ 的 JSON。
项目地址:https://gitcode.com/gh_mirrors/js/json

·
在做springboot项目时用到了json文件读取和解析,所以在这里记录一下学习过程中总结的一些点,希望对大家有帮助~
-
配置fastJson
<!--引入fastjson依赖-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.35</version>
</dependency>
-
构建工具类(方便多次调用时重复使用)
public static JSONObject readJsonFile(String filename){
String jsonString = "";
File jsonFile = new File(filename);
try {
FileReader fileReader = new FileReader(jsonFile);
Reader reader = new InputStreamReader(new FileInputStream(jsonFile),"utf-8");
int ch = 0;
StringBuffer stringBuffer = new StringBuffer();
while ((ch = reader.read()) != -1){
stringBuffer.append((char) ch);
}
fileReader.close();
reader.close();
jsonString = stringBuffer.toString();
} catch (FileNotFoundException e){
JSONObject notFoundJson = new JSONObject();
notFoundJson.put("code",Code.GET_ERR);
notFoundJson.put("msg","该地区GeoJson文件不存在!");
return notFoundJson;
} catch (IOException e) {
e.printStackTrace();
}
return JSONObject.parseObject(jsonString);
}
-
json文件示例(以geojson为例,数据结构比较复杂,只是层次比较多)
{
"type": "FeatureCollection",
"features": [
{
"type": "Feature",
"properties": {
"adcode": 110101,
"name": "东城区",
"center": [
116.418757,
39.917544
],
"centroid": [
116.416739,
39.912912
],
"childrenNum": 0,
"level": "district",
"acroutes": [
100000,
110000
],
"parent": {
"adcode": 110000
}
},
"geometry": {
"type": "MultiPolygon",
"coordinates": [
[
[
[
116.387664,
39.960923
],
[
116.38948,
39.961038
],
[
116.389506,
39.963147
],
[
116.396959,
39.963204
]
]
]
]
}
}
]
}
-
调用工具类读取数据:
String filePath = "文件路径";
// 读取json文件
JSONObject jsonObejct = readJsonFile(filePath);
-
读取json对象中的"features"字段内容,是数组类型的,采用以下方式:
// 方式一
JSONArray featureArray = JSON.parseArray(jsonObejct.get("features").toString());
// 方式二
JSONArray featureArray = jsonObejct.getJSONArray("features");
-
读取对象类型字段:
// 方式一
JSONObject propertiesObject = JSONObject.parseObject(regionObject.getString("properties"));
// 方式二
JSONObject propertiesObject = jsonObejct.getJSONArray("features").getJSONObject(0).getJSONObject("properties");
-
读取字符串类型:
// 方式一
String type = jsonObejct.get("type").toString();
// 方式二
String type = jsonObejct.getString("type");
-
读取整数类型:
// 方式一
String type = jsonObejct.get("type").toString();
// 方式二
String type = jsonObejct.getString("type");
-
整体解析:
String filePath = "文件地址/文件名.json";
JSONObject jsonObejct = ReadJsonUtils.readJsonFile(filePath);
// 方式一(很复杂,语句分开,但是结构清晰)
// 读取json文件的features字段,并转换为json数组
JSONArray featureArray = JSON.parseArray(jsonObejct.get("features").toString());
// 读取数组第一个元素,为地区对象
JSONObject regionObject = JSONObject.parseObject(featureArray.get(0).toString());
// 读取地区对象中的参数对象
JSONObject propertiesObject = JSONObject.parseObject(regionObject.getString("properties"));
// 读取参数对象的名称
String name = propertiesObject.getString("name");
// 读取参数对象的地区代码
int adcode = propertiesObject.getIntValue("adcode");
// 读取地区对象的几何对象
JSONObject geometryObject = JSONObject.parseObject(regionObject.get("geometry").toString());
// 读取几何字段中的坐标数组
JSONArray coordinates = JSONObject.parseArray(geometryObject.get("coordinates").toString());
// 读取几何对象中的类型名称
String type = geometryObject.getString("type");
// 方式二(无需每次重新转换类型,一行搞定)
String name = jsonObejct.getJSONArray("features").getJSONObject(0).getJSONObject("properties").getString("name");
String type = jsonObejct.getJSONArray("features").getJSONObject(0).getJSONObject("geometry").getString("type");
JSONArray coordinates = jsonObejct.getJSONArray("features").getJSONObject(0).getJSONObject("geometry").getJSONArray("coordinates");
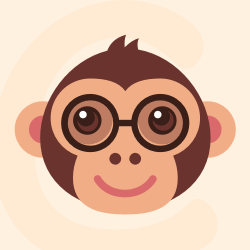



适用于现代 C++ 的 JSON。
最近提交(Master分支:1 个月前 )
960b763e
3 个月前
8c391e04
6 个月前
更多推荐
所有评论(0)