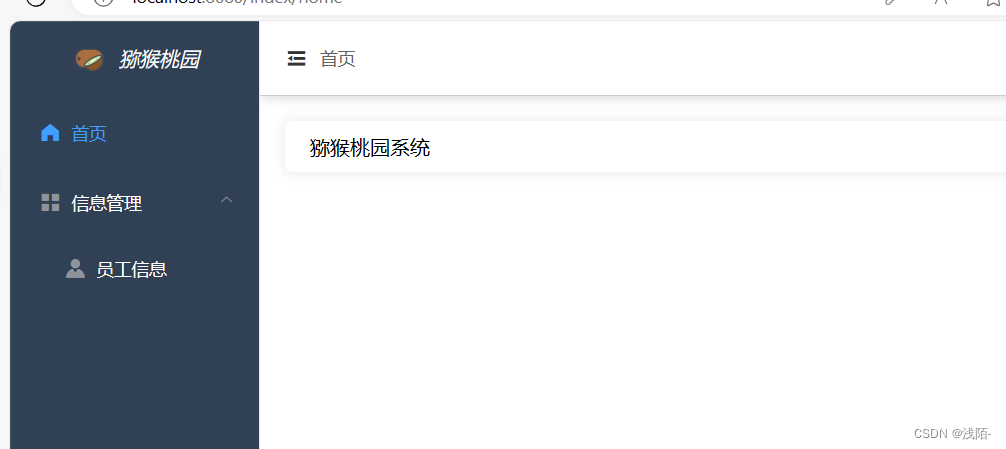
vue-cli使用侧边栏和面包屑以及三级路由跳转(通俗易懂,超易上手)

目录
该教程是为了给新手使用的,使用的是最普通的路由方式进行侧边栏和面包屑的显示(升级版有使用递归显示)
查看效果
路由设置
在router包下的index.js进行设置(chiledren代表的是该路由下的子路由)
index是我们的首页框架(里边并没有实际内容,我们需要通过路由跳转的方式进行内容展现)(<router-view></router-view>)
const routes = [
{
path:'/',
name: 'login',
component: Login
},
{
path:'/index',
name: 'index',
component:()=>import('../views/index.vue'),
//重定向
redirect:'/index/home',
children:[
{path:'home', name:'首页',component:()=>import('../views/Home.vue')},
{path:'user',name:"员工信息",component:()=>import('../views/user/index.vue')}
]
},
//访问其他非注册页面
{path:'*',name:'404',component:()=>import('../views/404.vue')}
]
侧边栏设置
我们是通过<el-menu-item>中设置index的值来进行跳转的,所以必须在<el-menu>中设置:default-active="this.$route.path"和router两个属性,index的值就是我们在router包中设置的要跳转的path值。因为我们这个是管理系统所以有用户和管理员多个角色。如果我们不想将某些内容展现给有些角色只需要使用v-if进行判断即可
<el-aside :width="sideWidth + 'px'" style="overflow-x: hidden;height: 100%;">
<el-menu style="height:100%;overflow-x: hidden;" background-color="rgb(48,65,86)" text-color="#fff"
active-text-color="#409eff" :collapse-transition="false" :collapse="isCollapse" :default-active="this.$route.path"
router>
<div style="height: 60px;line-height: 60px;text-align: center;">
<img src="@/assets/images/kiwi.png" alt="" style="width: 30px;position: relative;top: 10px; margin-right: 9px;">
<i style="color: #fff;" v-show="logoShow">猕猴桃园</i>
</div>
<el-menu-item index="/index/home">
<i class="el-icon-s-home"></i>
<span slot="title">首页</span>
</el-menu-item>
<el-submenu index="info">
<template slot="title">
<i class="el-icon-menu"></i>
<span>信息管理</span>
</template>
<el-menu-item index="/index/user">
<i class="el-icon-s-custom"></i>
<span>员工信息</span>
</el-menu-item>
</el-submenu>
</el-menu>
</el-aside>
然后我们设置
<el-main>
<router-view></router-view>
</el-main>
这代表的就是我们的main去要展现的就是我们路由中调转的页面
面包屑设置
我是通过查看父子路由来进行设置的,所以一定要在router中设置children路由,路由中的name就是我们要展现的面包屑中的名字。
因为我的一级路由是index.vue他只是一个框架并没有内容所以我将他替换成了home.vue(首页)
缺点:
刷新网页时因为路由没有变化,所以会清空pathList的值,会导致面包屑中的内容为空,因为我觉得这个影响不大就没有解决。
解决方案:
想要解决的话也很简单,每回获取路由后将pathList写入sessionStorage中就可以,然后每回先执行created方法从里边取出pathList的值就可以了(因为created比watch先执行,所以不影响其余变化)
<template>
<el-breadcrumb separator-class="el-icon-arrow-right" style="display: inline-block; margin-left: 10px;">
<el-breadcrumb-item :to="index !== pathList.length - 1 ? item.path : null" v-for="(item, index) in pathList"
:key="index">
{{ item.name }}
</el-breadcrumb-item>
</el-breadcrumb>
</template>
<script>
export default{
data(){
return{
pathList: [],
}
},
watch: {
//监听路由变化
'$route': function () {
let _this = this
_this.pathList = []
// console.log(this.$route.matched)
this.$route.matched.forEach(function (element, index, array) {
// console.info(element); //当前元素的值
// console.info(index); //当前下标
//第一个地址(index)不存,其余存入
if (index == 0 ) {
_this.pathList.push({ name: '首页', path: '/index/home' })
}
if (index != 0 && element.name != '首页') {
_this.pathList.push({ name: element.name, path: element.path })
}
});
}
}
}
</script>
完整代码
router路由
import Vue from 'vue'
import VueRouter from 'vue-router'
import Login from '../views/login/Login.vue'
Vue.use(VueRouter)
const routes = [
{
path:'/',
name: 'login',
component: Login
},
{
path:'/index',
name: 'index',
component:()=>import('../views/index.vue'),
//重定向
redirect:'/index/home',
children:[
{path:'home', name:'首页',component:()=>import('../views/Home.vue')},
{path:'user',name:"员工信息",component:()=>import('../views/user/index.vue')}
]
},
//访问其他非注册页面
{path:'*',name:'404',component:()=>import('../views/404.vue')}
]
const router = new VueRouter({
mode:'history',
base:process.env.BASE_URL,
routes
})
export default router
index.vue页面
<template>
<el-container style="height: 100%;">
<el-aside :width="sideWidth + 'px'" style="overflow-x: hidden;height: 100%;">
<el-menu style="height:100%;overflow-x: hidden;" background-color="rgb(48,65,86)" text-color="#fff"
active-text-color="#409eff" :collapse-transition="false" :collapse="isCollapse" :default-active="this.$route.path"
router>
<div style="height: 60px;line-height: 60px;text-align: center;">
<img src="@/assets/images/kiwi.png" alt="" style="width: 30px;position: relative;top: 10px; margin-right: 9px;">
<i style="color: #fff;" v-show="logoShow">猕猴桃园</i>
</div>
<el-menu-item index="/index/home">
<i class="el-icon-s-home"></i>
<span slot="title">首页</span>
</el-menu-item>
<el-submenu index="info">
<template slot="title">
<i class="el-icon-menu"></i>
<span>信息管理</span>
</template>
<el-menu-item index="/index/user">
<i class="el-icon-s-custom"></i>
<span>员工信息</span>
</el-menu-item>
</el-submenu>
</el-menu>
</el-aside>
<el-container>
<el-header style=" font-size: 12px;">
<!-- 收缩按钮 -->
<div style="flex: 1; font-size: 18px;">
<span :class="collapseBtnClass" style="cursor: pointer" @click="collapse"></span>
<el-breadcrumb separator-class="el-icon-arrow-right" style="display: inline-block; margin-left: 10px;">
<el-breadcrumb-item :to="index !== pathList.length - 1 ? item.path : null" v-for="(item, index) in pathList"
:key="index">
{{ item.name }}
</el-breadcrumb-item>
</el-breadcrumb>
</div>
<el-dropdown style="width: 70px; cursor: pointer">
<span>用户名</span><i class="el-icon-arrow-down" style="margin-Left:5px"></i>
<el-dropdown-menu slot="dropdown">
<el-dropdown-item>个人信息</el-dropdown-item>
<el-dropdown-item>退出</el-dropdown-item>
</el-dropdown-menu>
</el-dropdown>
</el-header>
<el-main>
<router-view></router-view>
</el-main>
</el-container>
</el-container>
</template>
<style>
.el-header {
/* background-color: #B3C0D1; */
color: #333;
line-height: 60px;
border-bottom: 1px solid #ccc;
box-shadow: 0 0 10px rgba(0, 0, 0, .2);
display: flex;
}
.el-aside {
color: #333;
}
.el-menu-item-group__title {
padding: 0 !important;
}
</style>
<script>
export default {
data() {
return {
//收缩按钮
collapseBtnClass: 'el-icon-s-fold',
isCollapse: false,
sideWidth: 200,
logoShow: true,
curUser: {
userId: '',
userName: '',
isAdmin: '',
},
pathList: [],
}
},
methods: {
//收缩按钮
collapse() {
this.isCollapse = !this.isCollapse;
if (this.isCollapse) {
this.sideWidth = 64
this.collapseBtnClass = 'el-icon-s-unfold'
this.logoShow = false
} else {
this.sideWidth = 200
this.collapseBtnClass = 'el-icon-s-fold'
this.logoShow = true
}
},
},
created() {
},
watch: {
//监听路由变化
'$route': function () {
let _this = this
_this.pathList = []
// console.log(this.$route.matched)
this.$route.matched.forEach(function (element, index, array) {
// console.info(element); //当前元素的值
// console.info(index); //当前下标
//第一个地址(index)不存,其余存入
if (index == 0 ) {
_this.pathList.push({ name: '首页', path: '/index/home' })
}
if (index != 0 && element.name != '首页') {
_this.pathList.push({ name: element.name, path: element.path })
}
});
console.log(_this.pathList)
}
}
};
</script>
home.vue页面
<template>
<div>
<div style="box-shadow: 0 0 10px rgba(0,0,0,.1);padding: 10px 20px;border-radius: 5px;margin-bottom: 10px;">猕猴桃园系统</div>
</div>
</template>
<style>
.el-menu-item-group__title {
padding: 0 !important;
}
</style>
<script>
export default {
data() {
};
return {
sideWidth: 200,
logoShow: true
}
},
methods: {
},
};
</script>
user页面
<template>
<div>员工信息</div>
</template>
<script>
export default{
name:'User',
data(){
return{}
},
methods:{},
created(){}
}
</script>
App.vue
<template>
<div id="app">
<router-view/>
</div>
</template>
<script>
</script>
<style>
html,body,#app{
height: 100%;
margin: 0;
padding: 0;
}
</style>
三级路由跳转
前言
这是我在写三级路由时的解决方案,因为我写前文时并没有遇到三级路由这个问题,所以会对前文的一些代码有所改动,不需要三级路由的可以直接看前文。
因为我们的管理界面有时候需要再一个页面再次进行跳转(比如新增数据,如果我们没有使用弹窗来完成的话就需要去新的一个页面),而这可能导致一个问题,我们的路由跳转过去了,但是页面没有变化。这是因为二级路由和三级路由共用了router-view所以导致三级路由界面没有显示。
而我也在查阅了大量资料后想到了解决办法。话不多说先上效果图
效果图
这是二级路由界面
当我们点击新增时跳转到三级路由
关键代码
我是将原本的二级路由放到和三级路由平级,然后通过重定向去访问原本的二级路由来实现的
代码变动
因为我改的代码也不多,所以就不全放了,完整代码前面有,我只将改动代码放这里就好了
router路由
这个是核心,我还将他们的路径给变动了一下,需要注意
注意:不需要给三级路由中原本的二级路由添加name,因为我在面包屑中对他判空了,如果添加面包屑会异常(它本身也会使用原本的名字,所以也不需要添加)
{
path:'/index',
name: 'index',
component:()=>import('../views/index.vue'),
//重定向
redirect:'/index/home',
children:[
{path:'home', name:'首页',component:()=>import('../views/Home.vue')},
{path:'/index/user',name:"员工信息",component:{render(c){return c('router-view')}}
,redirect:'/index/user',
children:[
{path:'/index/user',component:()=>import('../views/user/index.vue')},
{path:'/index/user/add',name:"新增员工",component:()=>import('../views/user/Add.vue')}
]}
]
},
index.vue的侧边栏
因为我将路由的路径改动了,所以侧边栏的路径也需要变动
<el-menu-item index="/index/home">
<i class="el-icon-s-home"></i>
<span slot="title">首页</span>
</el-menu-item>
<el-submenu index="info">
<template slot="title">
<i class="el-icon-menu"></i>
<span>信息管理</span>
</template>
<el-menu-item index="/index/user">
<i class="el-icon-s-custom"></i>
<span>员工信息</span>
</el-menu-item>
index.vue的watch监控
因为我将二级路由改为了三级路由,所以面包屑会有所变动,所以我也需要将判断条件修改一下
watch: {
//监听路由变化
'$route': function () {
let _this = this
_this.pathList = []
// console.log(this.$route.matched)
this.$route.matched.forEach(function (element, index, array) {
// console.info(element); //当前元素的值
// console.info(index); //当前下标
//第一个地址(index)不存,其余存入
if (index == 0) {
_this.pathList.push({ name: '首页', path: '/index/home' })
}
//这里使用了三级路由跳转,所以需要判断name是否为null
if (index != 0 && element.name != '首页' && element.name != null) {
_this.pathList.push({ name: element.name, path: element.path })
}
});
console.log(_this.pathList)
}
}
三级路由跳转
我们只需要给按钮添加个事件让他跳转即可
<el-button icon="el-icon-circle-plus-outline" type="primary" style="margin-left: 10px;" @click="addUser">新增</el-button>
addUser(){
this.$router.push({ path: "/index/user/add" }).catch(() => { });
},
完结撒花(如果后续这方面我还遇到问题我还会继续补充的)
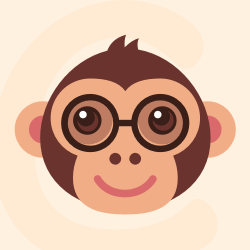



更多推荐
所有评论(0)