Vue组件通信(父传子、子传父、兄弟通信)

一、父传子
首先在父组件的子组件上定义一个属性,在属性上挂载要传输的变量,然后在子组件中通过
props
来接收
1、父组件parent.vue
<template>
<div class="parent">
<h2>{{ msg }}</h2>
<son :fa-msg="msg"></son> <!-- 子组件绑定faMsg变量,注意驼峰-->
</div>
</template>
<script>
import son from './Son' //引入子组件
export default {
name: 'HelloWorld',
data () {
return {
msg: '父组件',
}
},
components:{son},
}
</script>
2、子组件son.vue
<template>
<div class="son">
<p>{{ sonMsg }}</p>
<p>子组件接收到内容:{{ faMsg }}</p>
</div>
</template>
<script>
export default {
name: "son",
data(){
return {
sonMsg:'子组件',
}
},
props:['faMsg'],//接收psMsg值
}
</script>
子组件通过props来接受数据
,一共有三种接收数据的方式
第一种:
props: ['faMsg']
第二种
props: {
faMsg: String //这里指定了字符串类型,如果类型不一致会警告的哦
}
第三种
props: {
childCom: {
type: String,
default: 'sichaoyun'
}
}
二、子传父
在父组件的子组件上定义一个方法,挂载子组件传递过来的参数,然后在子组件中,通过
this.$emit("方法名",数据)
来传送
1、父组件代码:
<template>
<div class="parent">
<h2>{{ msg }}</h2>
<p>父组件接手到的内容:{{ username }}</p>
<son psMsg="我是你爸爸" @transfer="getUser"></son>
<!-- 监听子组件触发的transfer事件,然后调用getUser方法 -->
</div>
</template>
<script>
import son from './Son'
export default {
name: 'HelloWorld',
data () {
return {
msg: '父组件',
username:'',
}
},
components:{son},
methods:{
getUser(msg){
this.username= msg
}
}
}
</script>
2、子组件代码:
<template>
<div class="son">
<p>{{ sonMsg }}</p>
<p>子组件接收到内容:{{ psMsg }}</p>
<!--<input type="text" v-model="user" @change="setUser">-->
<button @click="setUser">传值</button>
</div>
</template>
<script>
export default {
name: "son",
data(){
return {
sonMsg:'子组件',
user:'子传父的内容'
}
},
props:['psMsg'],
methods:{
setUser:function(){
this.$emit('transfer',this.user)//触发transfer方法,this.user 为向父组件传递的数据
}
}
}
</script>
三、兄弟之间通信
首先在src目录新建一个bus.js文件,抛出一个空的vue实例
在发送数据的一方,引入bus.js,再通过Bus. e m i t ( " 方 法 名 " , 参 数 ) 来 传 送 , 在 接 收 数 据 的 一 方 , 引 入 b u s . j s , 再 通 过 B u s . emit("方法名",参数)来传送, 在接收数据的一方,引入bus.js,再通过Bus. emit("方法名",参数)来传送,在接收数据的一方,引入bus.js,再通过Bus.on(“方法名”,参数)来接收
在发送数据的一方
<!-- A.vue -->
<template>
<button @click="sendMsg()">-</button>
</template>
<script>
import bus from "../bus.js";
export default {
methods: {
sendMsg() {
bus.$emit("aMsg", '来自A页面的消息');
}
}
};
</script>
在接收数据的一方
<!-- IncrementCount.vue -->
<template>
<p>{{msg}}</p>
</template>
<script>
import bus from "../bus.js";
export default {
data(){
return {
msg: ''
}
},
mounted() {
bus.$on("aMsg", (msg) => {
// A发送来的消息
this.msg = msg;
});
}
};
</script>
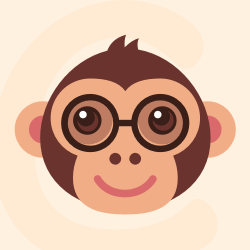



更多推荐
所有评论(0)