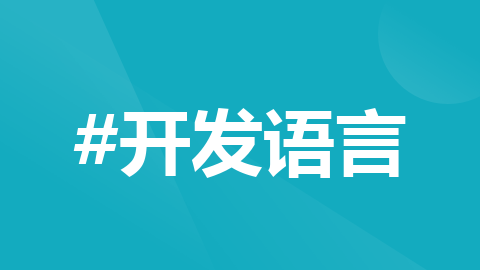
C 语言实现二维数组的 Hilbert 变换
bert
TensorFlow code and pre-trained models for BERT
项目地址:https://gitcode.com/gh_mirrors/be/bert

·
本文将介绍如何在 C 语言中实现对一个二维数组进行 Hilbert 变换。我们将使用 FFTW 库来完成快速傅里叶变换 (FFT) 和逆快速傅里叶变换 (IFFT),并对每一列应用 Hilbert 变换。以下是详细的代码和解释。
源码:文章末尾。
安装 FFTW 库
首先,确保你已经安装了 FFTW 库:
- 在 Ubuntu 上使用以下命令安装 FFTW 库:
sudo apt-get install libfftw3-dev
一维 Hilbert 变换函数
我们先实现一个函数,用于对一维信号进行 Hilbert 变换。
#include <stdio.h>
#include <fftw3.h>
#include <stdlib.h>
// Function to compute Hilbert transform of a 1D signal
void hilbert_1d(double* in, fftw_complex* out, int n) {
fftw_complex* fft_result = (fftw_complex*)fftw_malloc(sizeof(fftw_complex) * n);
fftw_complex* ifft_result = (fftw_complex*)fftw_malloc(sizeof(fftw_complex) * n);
fftw_plan p = fftw_plan_dft_r2c_1d(n, in, fft_result, FFTW_ESTIMATE);
fftw_plan q = fftw_plan_dft_c2r_1d(n, ifft_result, out, FFTW_ESTIMATE);
fftw_execute(p); // Perform FFT
// Apply the Hilbert transform in frequency domain
for (int i = 0; i < n; ++i) {
if (i > 0 && i < (n + 1) / 2) {
fft_result[i][0] *= 2.0; // Multiply real part by 2
fft_result[i][1] *= 2.0; // Multiply imaginary part by 2
} else if (i >= (n + 1) / 2) {
fft_result[i][0] = 0.0;
fft_result[i][1] = 0.0;
}
}
fftw_execute(q); // Perform IFFT
// Normalize the result
for (int i = 0; i < n; ++i) {
out[i][0] /= n;
out[i][1] /= n;
} fftw_destroy_plan(p);
fftw_destroy_plan(q);
fftw_free(fft_result);
fftw_free(ifft_result);
}
解释
- 分配内存:使用
fftw_malloc
分配用于存储 FFT 和 IFFT 结果的内存。 - 创建计划:使用
fftw_plan_dft_r2c_1d
和fftw_plan_dft_c2r_1d
分别创建 FFT 和 IFFT 的计划。 - 执行 FFT:调用
fftw_execute
执行 FFT 变换。 - Hilbert 变换:在频域中调整 FFT 结果,实现 Hilbert 变换。具体来说,将正频率部分乘以 2,将负频率部分设为 0。
- 执行 IFFT:调用
fftw_execute
执行 IFFT 变换。 - 归一化:对结果进行归一化处理。
- 清理:销毁计划并释放内存。
主函数
接下来,我们将这个一维 Hilbert 变换函数应用到二维数组的每一列。
int main() {
int rows = 32;
int cols = 1024;
double A[32][1024];
fftw_complex B[32][1024]; // Initialize the input array with some values
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
A[i][j] = (double)(i * cols + j); // Example initialization
}
}
for (int i = 0; i < cols; ++i) {
double col[32];
fftw_complex col_hilbert[32];
for (int j = 0; j < rows; ++j) {
col[j] = A[j][i];
}
hilbert_1d(col, col_hilbert, rows);
for (int j = 0; j < rows; ++j) {
B[j][i][0] = col_hilbert[j][0];
B[j][i][1] = col_hilbert[j][1];
}
} // Print the result
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
printf("(%f, %f) ", B[i][j][0], B[i][j][1]);
}
printf("\n");
}
return 0;
}
解释
- 初始化输入数组:将输入数组
A
初始化为示例值,你可以根据实际需求进行初始化。 - Hilbert 变换:对每一列应用
hilbert_1d
函数,并将结果存储在二维数组B
中。 - 打印结果:打印变换后的二维数组结果。
编译和运行
使用以下命令进行编译和运行:
gcc -o hilbert_transform hilbert_transform.c -lfftw3 -lm ./hilbert_transform
确保在编译时链接了 FFTW 库 (-lfftw3
) 和数学库 (-lm
)。
总结
本文介绍了如何在 C 语言中实现对二维数组进行 Hilbert 变换。通过使用 FFTW 库,我们能够高效地进行快速傅里叶变换和逆傅里叶变换,从而实现 Hilbert 变换。
传送阵:hilbert转换源码
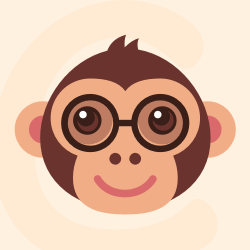



TensorFlow code and pre-trained models for BERT
最近提交(Master分支:2 个月前 )
eedf5716
Add links to 24 smaller BERT models. 4 年前
8028c045 - 4 年前
更多推荐
所有评论(0)