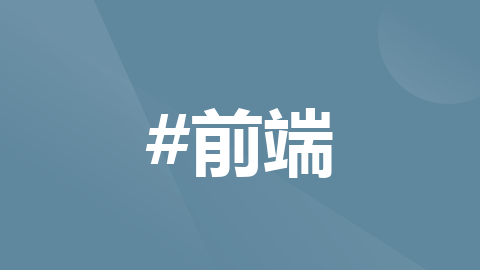
Vue应用中适配多端(移动端、PC端)时,使用px和rem单位进行布局是一种常见的做法
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
在Vue应用中适配多端(移动端、PC端)时,使用px
和rem
单位进行布局是一种常见的做法。px
是像素单位,而rem
是基于根元素(html
)的字体大小的相对单位。以下是一些使用px
和rem
相互转换的多场景详细使用案例:
1. 基础转换
场景:将设计稿的px
值转换为rem
值。
案例:
/* 假设设计稿中的设计尺寸为375px宽,即iPhone 6的屏幕宽度 */
html {
font-size: 16px; /* 1rem = 16px */
}
.container {
width: 23.4375rem; /* 375px / 16px */
margin: 10px; /* 10px / 16px */
}
2. 响应式设计
场景:创建响应式布局,适配不同屏幕尺寸。
案例:
html {
font-size: 16px; /* 默认字体大小 */
}
@media (min-width: 768px) {
html {
font-size: 18px; /* PC端字体大小 */
}
}
/* 使用rem单位创建响应式宽度 */
.content {
width: 90%;
max-width: 60rem; /* 960px / 16px */
}
3. 组件级别的适配
场景:在Vue组件中使用px
和rem
进行布局。
案例:
<template>
<div class="component">
<!-- 组件内容 -->
</div>
</template>
<style scoped>
.component {
padding: 20px; /* 20px / 16px */
font-size: 1rem; /* 16px */
}
</style>
4. 使用第三方库
场景:使用第三方库(如bootstrap-vue
)进行响应式布局。
案例:
<template>
<div>
<b-container>
<b-row>
<b-col cols="12" md="8" lg="6">
<!-- 内容 -->
</b-col>
</b-row>
</b-container>
</div>
</template>
<style>
/* 可以根据需要覆盖BootstrapVue的样式 */
</style>
5. 使用CSS变量
场景:使用CSS变量进行px
和rem
的转换。
案例:
:root {
--base-font-size: 16px;
}
html {
font-size: var(--base-font-size);
}
.card {
width: calc(375px / var(--base-font-size)); /* 375px / 16px */
padding: 0.625rem; /* 10px / 16px */
}
6. 动态调整根元素字体大小
场景:根据屏幕宽度动态调整根元素字体大小。
案例:
export default {
mounted() {
this.adjustFontSize();
window.addEventListener('resize', this.adjustFontSize);
},
beforeDestroy() {
window.removeEventListener('resize', this.adjustFontSize);
},
methods: {
adjustFontSize() {
const width = document.documentElement.clientWidth;
document.documentElement.style.fontSize = `${width / 10}px`; // 例如:屏幕宽度的10%
}
}
};
注意事项
- 使用
rem
单位时,确保根元素的字体大小适当,以便在不同设备上保持一致性。 - 在使用媒体查询进行响应式设计时,选择适当的断点。
- 动态调整根元素字体大小时,注意性能影响,避免频繁触发重排。
- 考虑使用CSS预处理器(如Sass或Less)来简化
px
和rem
的转换。
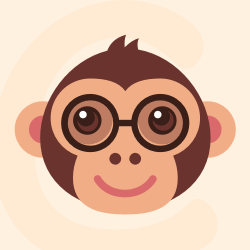



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:2 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 4 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 5 个月前
更多推荐
所有评论(0)