vue3 ts 使用 pinia
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
1. 安装 pinia, npm i pinia
2. 在 main.ts
文件中引入 pinia
import { createApp, App } from 'vue'
import { createPinia, Pinia } from 'pinia'
import AppComponent from './App.vue'
const app: App = createApp(AppComponent)
const pinia: Pinia = createPinia()
app.use(pinia)
app.mount('#app')
3. 新建 /src/store
目录存放公共状态文件,例如
3.1 新建 test.type.ts
定义类型
type TestState = {
count: number
user: {
age: number
name: string
}
}
type TestGetters = {
doubleCount: (state: TestState) => number
username: (state: TestState) => string
}
type TestActions = {
setCount: (count: number) => void
}
// 注意:如有导入导出的依赖数据或类型,自定义test类型需要使用export导出;否则可视为全局类型即可直接使用
3.2 新建 test.ts
创建test模块公共状态
import { defineStore } from 'pinia'
// 在此约束类型不管编写或使用都可有较好的提示
export const useTestStore = defineStore<string, TestState, TestGetters, TestActions>('TestId', {
state: () => ({
count: 10,
user: {
age: 18,
name: '小明'
}
}),
getters: {
doubleCount: (state) => state.count * 2,
username: (state) => state.user.name
},
actions: {
setCount(count: number) {
this.count = count
}
}
})
4. 最后在任意页面使用即可
<script setup lang="ts">
import { useTestStore } from '@/store/test'
const testStore = useTestStore()
console.log(testStore.count)
console.log(testStore.user.name)
console.log(testStore.doubleCount)
console.log(testStore.username)
// 修改state数据方式1
testStore.count = 11
testStore.$state.count = 11
// 修改state数据方式2
testStore.$patch({
count: 11
})
testStore.$patch(state => {
state.count = 11
})
// 修改state数据方式3(不建议)
testStore.$state = {
count: 11
}
// 修改state数据方式4(建议)
testStore.setCount(11)
</script>
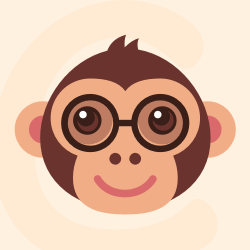



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:2 个月前 )
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 4 个月前
e428d891
Updated Browser Compatibility reference. The previous currently returns HTTP 404. 5 个月前
更多推荐
所有评论(0)