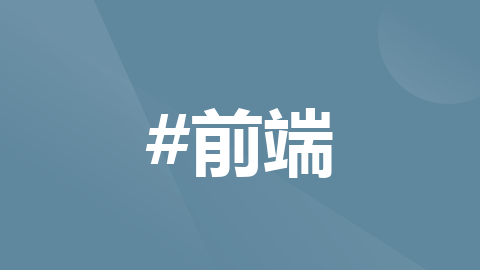
elementui表格el-table自定义复选框,并给复选框增加悬浮提示
element
A Vue.js 2.0 UI Toolkit for Web
项目地址:https://gitcode.com/gh_mirrors/eleme/element

·
实现方法:
需求
给el-table的全选复选框、禁用的复选框、未禁用的复选框用el-tooltip分别增加不同的悬浮提示信息。
实现代码
html代码:
<el-table
ref="multipleTable"
:data="tableData"
tooltip-effect="dark"
border>
<el-table-column align="center" width="55">
<template slot="header" slot-scope="{row}">
<!-- 全选按钮 -->
<div>
<el-tooltip placement="top-start" effect="light" popper-class="table_select_tooltip">
<div slot="content">
<p>全选:悬浮展示的信息</p>
</div>
<el-checkbox v-model="isCheck" :indeterminate="indeterminate" @change="handleCheckAllChange"></el-checkbox>
</el-tooltip>
</div>
</template>
<template slot-scope="{row}">
<!-- 多选按钮 -->
<el-checkbox-group v-model="selectionData">
<el-tooltip placement="top-start" effect="light" popper-class="table_select_tooltip">
<div slot="content">
<p>{{ row.disabled ? '禁用:悬浮展示的信息':'悬浮展示的信息' }}</p>
</div>
<!-- 此处的label是复选框右边显示的值,也是选中后的值。如果要一行的数据,直接使用row即可 -->
<!-- label的内容会显示在页面上,需要配合css把label的内容隐藏掉 -->
<el-checkbox :disabled="row.disabled" :key="row.id" :label="row.id"></el-checkbox>
</el-tooltip>
</el-checkbox-group>
</template>
</el-table-column>
</el-table>
js代码
export default {
name:'',
components: {},
props:[],
data() {
return {
tableData: [],//表格数据
enabledDataList: [],//这个指表格中没有被禁用的行数据,进来组件的时候需要自己处理下
isCheck: false,//全选按钮绑定值
indeterminate: false,//全选按钮的不确定状态,选中数据selectionData的长度length符合 0 < selectionData.length < enabledDataList.length ,值为true
selectionData: [],//表格多选选中的数据
};
},
computed: {},
watch: {
// 监听选中的数据,选中的数据发生变化,通过判断长度给多选按钮赋值
selectionData: {
handler(v) {
if (this.enabledDataList.length) {
let checkedCount = v.length;
this.isCheck = checkedCount === this.enabledDataList.length;
this.indeterminate = checkedCount > 0 && checkedCount < this.enabledDataList.length;
}
},
immediate: true
}
},
created() {
// 给enabledDataList赋值,需要去掉被禁用的数据
this.enabledDataList = this.tableData.filter(v=>!v.disabled)
},
mounted() {},
methods: {
// 全选
handleCheckAllChange(value){
this.selectionData = value ? this.enabledDataList.map(el=>el.id) : [];
this.indeterminate = false;
},
}
};
实现效果
完整代码
<template>
<div class='el-table-con'>
<p>选中的数据:{{ selectionData }}</p>
<el-table
ref="multipleTable"
:data="tableData"
tooltip-effect="dark"
border>
<el-table-column align="center" width="55">
<template slot="header" slot-scope="{row}">
<div>
<el-tooltip placement="top-start" effect="light" popper-class="table_select_tooltip">
<div slot="content">
<p>全选:悬浮展示的信息</p>
</div>
<el-checkbox v-model="isCheck" :indeterminate="indeterminate" @change="handleCheckAllChange"></el-checkbox>
</el-tooltip>
</div>
</template>
<template slot-scope="{row}">
<el-checkbox-group v-model="selectionData">
<el-tooltip placement="top-start" effect="light" popper-class="table_select_tooltip">
<div slot="content">
<p>{{ row.disabled ? '禁用:悬浮展示的信息':'悬浮展示的信息' }}</p>
</div>
<!-- 此处的label是复选框右边显示的值,也是选中后的值。如果要一行的数据,直接使用row即可 -->
<!-- label的内容会显示在页面上,需要配合css把label的内容隐藏掉 -->
<el-checkbox :disabled="row.disabled" :key="row.id" :label="row.id"></el-checkbox>
</el-tooltip>
</el-checkbox-group>
</template>
</el-table-column>
<el-table-column
prop="id"
label="id"
width="50">
</el-table-column>
<el-table-column
prop="name"
label="姓名">
</el-table-column>
</el-table>
</div>
</template>
<script>
export default {
name:'',
components: {},
props:[],
data() {
return {
tableData: [{
id: 1,
name: '王小虎1',
disabled: true
}, {
id: 2,
name: '王小虎2',
disabled: false
}, {
id: 3,
name: '王小虎3',
disabled: true
}, {
id: 4,
name: '王小虎4',
disabled: false
}, {
id: 5,
name: '王小虎5',
disabled: true
}, {
id: 6,
name: '王小虎6',
disabled: false
}, {
id: 7,
name: '王小虎7',
disabled: true
}],
enabledDataList: [],//这个指没有被禁用的行,进来组件的时候需要自己处理下
isCheck: false,//全选按钮绑定值
indeterminate: false,//全选按钮的不确定状态
selectionData: [],//表格选中的数据
};
},
computed: {},
watch: {
// 监听选中的数据
selectionData: {
handler(v) {
if (this.enabledDataList.length) {
let checkedCount = v.length;
this.isCheck = checkedCount === this.enabledDataList.length;
this.indeterminate = checkedCount > 0 && checkedCount < this.enabledDataList.length;
}
},
immediate: true
}
},
created() {
// 给enabledDataList赋值,需要去掉被禁用的数据
this.enabledDataList = this.tableData.filter(v=>!v.disabled)
},
mounted() {},
methods: {
// 全选
handleCheckAllChange(value){
this.selectionData = value ? this.enabledDataList.map(el=>el.id) : [];
this.indeterminate = false;
},
}
};
</script>
<style lang='scss' scoped>
.el-table {
/deep/ .el-checkbox__label {
display: none;
}
}
</style>
效果:
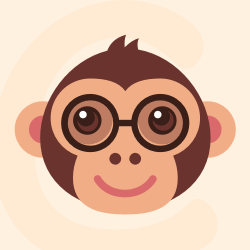



A Vue.js 2.0 UI Toolkit for Web
最近提交(Master分支:3 个月前 )
c345bb45
7 个月前
a07f3a59
* Update transition.md
* Update table.md
* Update transition.md
* Update table.md
* Update transition.md
* Update table.md
* Update table.md
* Update transition.md
* Update popover.md 7 个月前
更多推荐
所有评论(0)